Welcome to our tutorial covering the exciting topic of Roblox Lua reference. If you’re passionate about gaming and programming, or you’re just keen to explore a new hobby, this guide will introduce you to the fundamental concepts and applications of Roblox Lua.
For the uninitiated, Lua is the coding language used in Roblox, one of the world’s most popular gaming platforms. It allows you to create and share your very own games. In exploring Roblox Lua, you’re tapping into a powerful tool that can truly amplify your creativity. But, where to begin? That’s exactly what this tutorial’s all about!
Table of contents
What is Roblox Lua?
At its core, Roblox Lua is a lightweight yet powerful scripting language. It’s been specifically designed with ease of use and simplicity in mind, making it a popular choice for those embarking on their coding journey.
What’s it For?
Roblox Lua empowers you to create, manipulate, and enhance your own Roblox games. With it, you can construct gameplay mechanics, design game interfaces, and build dynamic, interactive elements. It serves as the backbone of every function and feature you see in Roblox games.
Why Should I Learn Roblox Lua?
Learning Roblox Lua not only allows you to create and share your own virtual worlds; it’s also a stepping stone into the broader field of programming. As an accessible, beginner-friendly language, it’s an ideal starting point for aspiring programmers. Not to mention, with millions of active users on Roblox, your games have the potential to be enjoyed by a vast audience worldwide.
Now, let’s dive into some practical applications of Roblox Lua!
Understanding Variables and Data Types in Roblox Lua
As with any programming language, understanding variables and data types are fundamental to getting started with Roblox Lua. Let’s start by looking at variables.
local myVariable = "Hello, Zenva!" print(myVariable) -- Output: Hello, Zenva!
In this example, “myVariable” is a variable which contains the string “Hello, Zenva!”. When we use the ‘print’ function, the output will display the text stored in “myVariable”.
Here are some common data types you’ll encounter in Roblox Lua:
- Strings: These are sequences of characters, as seen in the example above.
- Numbers: These are numerical values.
- Booleans: These represent either true or false. They are useful for conditional logic.
local myString = "Zenva" local myNumber = 2020 local myBoolean = true print(myString) -- Output: Zenva print(myNumber) -- Output: 2020 print(myBoolean) -- Output: true
Understanding Functions in Roblox Lua
Functions are reusable blocks of code that perform a certain task. Here’s a basic example that defines a function to print a greeting:
function sayHello() print("Hello, Zenva!") end sayHello() -- Output: Hello, Zenva!
This function, ‘sayHello’, is declared without parameters and simply prints “Hello, Zenva!” when called.
Functions can also take parameters, making them more versatile:
function greetUser(name) print("Hello, " .. name .. "!") end greetUser("Zenva") -- Output: Hello, Zenva!
In this example, the ‘greetUser’ function accepts a parameter (name) and concatenates it into the greeting message.
Understanding Tables in Roblox Lua
Tables in Lua are flexible data structures that can hold any data type, similar to arrays in other languages. They can be indexed with numbers, strings, or any other data type.
Let’s illustrate this with some code:
local myTable = {"Zenva", "Coding", "Academy"} print(myTable[1]) -- Output: Zenva print(myTable[2]) -- Output: Coding print(myTable[3]) -- Output: Academy
In this example, ‘myTable’ is a table containing strings. The syntax myTable[1] is used to access the first element of the table.
Tables can also be indexed with strings, essentially creating a key-value pair structure, similar to dictionaries or objects in other languages:
local zenva = { name = "Zenva", mission = "Democratize technology and education." } print(zenva.name) -- Output: Zenva print(zenva.mission) -- Output: Democratize technology and education
Understanding Control Structures in Roblox Lua
Control structures in Lua include conditional statements and loops, which are fundamental constructs in any programming language. Let’s explore some examples:
The ‘if’ statement is a conditional construct allowing your code to perform different actions based on specific criteria:
local x = 10 if x > 5 then print("x is greater than 5") -- Output: x is greater than 5 end
In the example above, the code within the ‘if’ block is executed since the condition ‘x > 5’ is true.
For loops, a common example is the ‘for’ loop, which repeats a block of code a set number of times:
for i = 1, 5 do print(i) -- Output: 1, 2, 3, 4, 5 end
This ‘for’ loop starts at 1 and ends at 5, printing each number in the range.
With this knowledge, you’re ready to dive deeper into the world of Roblox Lua programming. Remember, practice is key when learning a new language. Happy coding!
Understanding Events in Roblox Lua
Events in Roblox Lua allow scripts to respond to specific occurrences, such as clicks or key presses. This can make your game interactive and responsive.
Let’s explore how we can respond to an event where a user clicks a part in the game:
local part = game.Workspace.Part part.ClickDetector.MouseClick:Connect(function(player) print(player.Name .. " clicked the part!") end)
Here, when a user clicks the part, their username is printed with the message.
Working with Roblox Services
Roblox Services are built-in features that provide specific functionalities. To use a Service, it needs to be retrieved with the ‘GetService’ method.
As an example, let’s explore how we can create a script that changes the time of day in the game every 10 seconds with the Lighting service:
local lighting = game:GetService("Lighting") while true do lighting:SetMinutesAfterMidnight(lighting:GetMinutesAfterMidnight() + 10) wait(10) end
This script continuously increments the time of day by 10 minutes every 10 seconds, creating a fast-forward effect.
Working with Player Input
Player input is at the heart of any interactive game. The UserInputService allows you to handle player input like keyboard and mouse events.
Suppose we wish to create a script that prints a message when the player presses the ‘E’ key. The code will look something like this:
local userInputService = game:GetService("UserInputService") userInputService.InputBegan:Connect(function(input) if input.KeyCode == Enum.KeyCode.E then print("E key was pressed") end end)
The code above listens for any key press event. When the ‘E’ key is pressed, the script prints the message “E key was pressed”.
By now you should be well equipped to start exploring more advanced topics in Roblox Lua scripting. Keep practicing and you’ll be creating your own interactive virtual worlds on Roblox in no time!
Now that you’ve taken your first steps into the terrain of Roblox Lua, you might be wondering, “Where do I go next?”. We encourage you to keep fueling this passion for game creation you’ve ignited. Remember – every expert was once a beginner, and continued exploration will only enhance your mastery.
For those feeling inspired to further explore the world of Roblox game development, we at Zenva recommend our comprehensive Roblox Game Development Mini-Degree. A vast array of courses resides under this program, diving into diverse topics like Roblox basics, Lua scripting, arena games creation, and crafting first-person shooter games. Perfect for beginners and available 24/7, your game creation journey can continue at your own pace. Plus, upon completion, you earn a certificate to appreciate your dedication and hard work.
In addition to the Mini-Degree, Zenva offers a broad collection of courses and resources through our comprehensive Roblox series. With over 250 supported courses designed with both beginners and professionals in mind, your coding journey doesn’t have to stop here. Keep curious, keep exploring.
Conclusion
The thrilling world of Roblox Lua awaits your exploration, and the potential to bring to life your own interactive gaming environments is within your reach. Remember, everyone has to start somewhere, and with continued practice, your proficiency in Roblox game creation will burgeon.
Leaning into the joy of learning and creating is the key to success, and at Zenva we’re happy to have been part of your journey. Whether you’re eager to expand your knowledge or just starting out, our comprehensive Roblox Game Development Mini-Degree will provide the essential resources you need to keep growing in your coding adventure. So, keep making, keep learning, and most importantly, keep playing. Happy coding!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
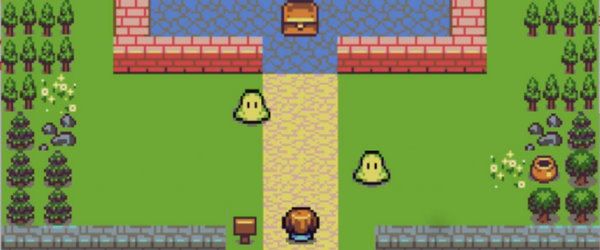
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.