Welcome to our deep dive into the fascinating world of the Roblox API. In this tutorial, we aim to unpack everything you need to know as a beginner, ensuring that you walk away with practical knowledge you can apply right away. No prior experience with APIs is required, making this the perfect starting point for your coding journey.
Table of contents
What is the Roblox API?
The Roblox API, also referred to as Roblox Lua API, is a set of services, functions and classes provided by Roblox for interacting with the game’s server and client. Through this API, you can create, control, and manipulate different gaming elements, essentially turning your visions into playable games.
What is it for?
Roblox API is used to power the functionality of Roblox games – from controlling in-game physics to creating game-level interactive systems such as player inventory or NPC behavior. It’s the magical cog that makes the gears of your game world turn.
Why should I learn it?
Learning to use the Roblox API is key for anyone interested in creating games on the Roblox platform. Not only does it allow you to add depth and complexity to your gaming experiences, but it also provides you with valuable programming skills that can be applied beyond Roblox. With a multitude of resources and a supportive community, there is no better time to get started.
Getting Started with Roblox API
Before diving into coding, it’s always a good idea to familiarize yourself with the environment you’ll be working with. Here’s how you can explore the Roblox API:
-- Sample code to output properties of the Workspace local Workspace = game:GetService('Workspace') for i,v in pairs(Workspace:GetProperties()) do print(i,v) end
This script will output all properties of the Workspace in the Roblox client. These properties include the Camera property, that tells you where the camera is positioned, and the Terrains property, that pertains to the terrain of the game.
Creating Your First Script
Now let’s get our hands dirty with scripting. We will use simple Lua scripts to interact with the Roblox API. Here’s a simple script to create a part in the workspace:
-- Create a new part local part = Instance.new("Part") part.Parent = game.Workspace part.BrickColor = BrickColor.new("Bright red") -- Sets the color of the part part.Anchored = true -- Makes sure the part doesn't fall due to gravity print("Part created!")
When you run this code, you will see a bright red part in your workspace. Sounds interesting, right?
Manipulating Parts in Workspace
After creating a part in the workspace, let’s learn how to manipulate its properties like position and size. We could create a function for that:
-- Adjust Part Properties function adjustPartProperties(part) part.Size = Vector3.new(1, 1, 1) -- Sets the size of the part part.Position = Vector3.new(0, 5, 0) -- Position the part end local NewPart = workspace.Part -- Reference the newly created part adjustPartProperties(NewPart) -- Call the function
By calling the function, the script changes the size of the part to a 1x1x1 cube and places the part at the position (0,5,0).
Adding Behavior to the Part
To make our game interactive, we can add behavior to our parts. Here’s a simple code to add a click detector to our part:
-- Add Click Detector local ClickDetector = Instance.new("ClickDetector") -- Creates a new ClickDetector ClickDetector.Parent = NewPart -- Assigns the ClickDetector to the new part -- Function to handle click event function onClick() print("Part was clicked!") end -- Connecting Click event to function ClickDetector.MouseClick:Connect(onClick)
When you run this, and click on the part in your game, it will print a message stating “Part was clicked!” in your output console.
Adding More Interactions
Making your game interactive enhances user engagement. Let’s enhance our game by introducing more interactive elements, starting with a simple movement control:
-- Move Part on Click function onClick() NewPart.Position = NewPart.Position + Vector3.new(0, 10, 0) print("Part was moved!") end
This code moves the Part 10 units upwards each time it is clicked.
Next, we could add functionality to change the color of our part on being clicked. You can replace the onClick function with this:
-- Change Color on Click function onClick() local colors = {"Bright red", "Bright blue", "Bright green", "Bright yellow", "Bright violet"} -- Array of colors local index = math.random(5) -- Random index from 1-5 NewPart.BrickColor = BrickColor.new(colors[index]) -- Sets the color print("Color changed! " .. colors[index]) end
Now, our game changes the color of the part to a random color from the array each time it’s clicked.
Expanding on User Interactions
Although the previous example makes single parts interactive, designing complex interactions requires us to take multiple parts into consideration. Let’s explore how this works:
-- Creating multiple parts for i=1,10 do local part = Instance.new("Part") -- Creates new part part.Parent = game.Workspace -- Adds the part to workspace part.Position = Vector3.new(i*10, 5, 0) -- Sets the position part.BrickColor = BrickColor.new(colors[math.random(5)]) -- Sets random color local ClickDetector = Instance.new("ClickDetector") -- Assigns click detector ClickDetector.Parent = part -- Adds the Click Detector to the part ClickDetector.MouseClick:Connect(onClick) -- Connects the click event to the function end
Here we are creating multiple parts, each with their own click detectors. This showcases how user interactions can be multiplied for a richer gaming experience.
Lastly, let’s weave in usercontrolled events into game variables:
-- Creating a score variable local score = 0 -- Update Score on Click function onClick() score = score + 1 print("Score: " .. score) end
Each time a part is clicked, the score increases by one. It’s an initial example of how you can create games with scores, levels, and other progress variables.
With this, you’ve now got a firm grasp of how to harness the power of the Roblox API to craft increasingly complex and interactive gaming experiences.
Mastering Advanced User Interactions
After understanding the basics, the next natural step is to delve into more complex interactions. One important concept to master is user input and how it affects your game. Consider the code below:
-- Function to handle Keyboard Input function onKeyPress(input) if input.KeyCode == Enum.KeyCode.W then NewPart.Position = NewPart.Position + Vector3.new(0, 10, 0) elseif input.KeyCode == Enum.KeyCode.A then NewPart.Position = NewPart.Position + Vector3.new(-10, 0, 0) elseif input.KeyCode == Enum.KeyCode.S then NewPart.Position = NewPart.Position + Vector3.new(0, -10, 0) elseif input.KeyCode == Enum.KeyCode.D then NewPart.Position = NewPart.Position + Vector3.new(10, 0, 0) end end game:GetService("UserInputService").InputBegan:Connect(onKeyPress)
This function moves the part based on the keyboard buttons W (up), A (left), S (down), and D (right). Thus, it allows players to move elements around in your game.
We can then extend this functionality further to encompass mouse wheel scroll that can change the size of parts:
-- Function to handle Mouse Wheel Scrolling function onMouseScroll(input) if input.UserInputType == Enum.UserInputType.MouseWheel then local change = input.Position.Z NewPart.Size = NewPart.Size + Vector3.new(change, change, change) end end game:GetService("UserInputService").InputChanged:Connect(onMouseScroll)
Now, scrolling with the mouse wheel increases or decreases the size of the part.
Ranging from text inputs…
-- Receive text from player function onTextEntered(text) print("Player said: "..text) end local TextService = game:GetService("TextService") TextService.StripWhitespacesAsync:Connect(onTextEntered)
This code receives text input from a player and prints it on the console.
…to even reacting to touch screens:
-- Handle <a class="wpil_keyword_link" href="https://gamedevacademy.org/unity-touch-input-tutorial/" target="_blank" rel="noopener" title="touch input" data-wpil-keyword-link="linked">touch input</a> function onTouch(input) NewPart.Position = input.Position end game:GetService("UserInputService").TouchTap:Connect(onTouch)
The part will now follow the location of the touch input on the touchscreen, enabling mobile players to interact with the game.
Working with Game Data
Equally crucial to mastering Roblox API is learning how to work with in-game data. This ranges from storing player progress…
-- Storing High Score for a player local DataStoreService = game:GetService("DataStoreService") local ScoreStore = DataStoreService:GetDataStore("HighScoreStore") -- Saving the score for a player function saveScore(userId, score) ScoreStore:SetAsync(tostring(userId), score) end
…to retrieving data and displaying it to the players:
-- Displaying high score for a player function showHighScore(userId) local score = ScoreStore:GetAsync(tostring(userId)) print("High Score: "..tostring(score)) end
Used together, these data manipulation functions can essentially allow you to create complete games with persistent player data, statistics, and more.
Taking the time to learn and master the Roblox API will open up endless possibilities to create fantastic and engaging games for players around the world. Happy coding!
Having familiarized yourself with the basics of Roblox API, the next step is to delve deeper and continue expanding your skills. As you further explore and experiment, your games will gradually become richer and increasingly robust.
One of the best ways to do this is through our Roblox Game Development Mini-Degree. It offers an in-depth understanding of Roblox Studio and Lua, and covers a wide range of game genres. This comprehensive course is designed for learners of all experience levels – whether you’re a complete novice or an experienced developer, you’ll find valuable insights here. Taught by qualified instructors certified by Unity Technologies and CompTIA, it’s a fantastic way to develop the skills needed to thrive in the growing Roblox market.
If you want to explore a wider array of topics, check out our vast collection of Roblox courses. These not only cover game creation but also delve into different aspects of Roblox development, giving you a more rounded understanding of the platform. We at Zenva aim to equip you with knowledge and skills that will help you succeed as you journey from beginner to professional, as a developer in not just Roblox, but also programming, AI and more.
Conclusion
Learning the Roblox API not only unlocks new doors of creativity for budding game developers, but also provides a solid foundation for understanding how APIs can power interactive experiences. Once thrust into the world of game development with such powerful tools at your disposal, the sky’s the limit.
So why wait? Kickstart your journey today and make your way towards becoming a proficient game developer with our meticulously designed, comprehensive Roblox Game Development Mini-Degree. Carve your path to success in the gaming industry and let’s build awesome games together!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
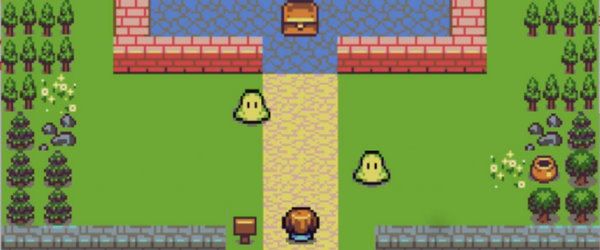
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.