Welcome, budding developers and seasoned code enthusiasts alike, to a deep dive into the Lua table library. In this tutorial, we invite you to journey with us into the mechanics of this powerful, yet accessible library. Our mission is to empower you to unlock the potential of Lua tables and unleash it in your programs and games.
Table of contents
What is the Lua table library?
At its core, the Lua table library is a collection of functions specifically designed to work with Lua’s tables. A table in Lua is the only compound data type which is used to represent various powerful data structures including arrays, sets, lists, and dictionaries. It’s, practically, the backbone of most of the data structures in Lua.
What is it for?
The Lua table library is vital in Lua programming for its role across a diverse range of applications. These functions give coders the tools to manipulate and manage data structures with ease, allowing you to sort, search, and manage tables with intuitive and effective methods. With Lua table library, data management becomes a cakewalk.
Why should I learn it?
The Lua table library isn’t just useful – it’s fundamental. It’s like the player’s toolbelt in a platform game, or an adventurer’s bag of tricks. As you travel deeper into the world of Lua, you’ll find that the table library is crucial at nearly every stage of development. Whether you’re creating or modifying data structures, or constructing complex game mechanics, understanding the Lua table library is key. Beyond that, Lua is embraced by popular game engines like Roblox and Love2D, owing to its lightweight, flexible nature and ease of learning. As a coder, armed with the knowledge of Lua table library, you are in a powerul position to craft engaging experiences in these platforms.
This is a beginner-friendly language that doesn’t skimp on power, so whether you’re just starting out or looking to expand your arsenal, the Lua table library is an excellent place to grow your coding journey.
Creating a Table in Lua
Let’s start with the basics. Here is how you create a table in Lua:
-- Create a table local myTable = {}
You can fill it with elements as follows:
-- Create a table with elements local myTable = {1, 2, 3, 4, 5}
Adding and Accessing Values in Tables
To add a value into your table, you would use the following code:
-- Add a value to the table table.insert(myTable, 6)
Accessing a value is as easy as specifying its index.
-- Access a value from the table print(myTable[1]) -- Will print 1, as Lua is 1-indexed
Removing Values from Tables
The Lua table library also facilitates removing values from tables. We can use the `remove` function for this.
-- Remove a value from the table table.remove(myTable, 1) -- Will remove the first element (in this case, 1)
Using Tables as Dictionaries
Another interesting aspect of Lua tables is that they can also act as dictionaries, permitting key-value pairs.
-- Create and fill a table as a dictionary local myDict = {key1 = 'Zenva', key2 = 'GameDev', key3 = 'Academy'} print(myDict['key1']) -- Will print "Zenva"
Manipulating Tables
You can use the `sort` function to sort your tables. Here’s how you can sort the elements of a table in ascending order:
-- Sort a table table.sort(myTable)
Remember, the Lua table library offers many other functions to help with your coding endeavors. Be sure to explore them all as you continue honing your Lua skills!
Sorting Tables in Descending Order
While sorting in ascending order is undoubtedly useful, sorting in descending order can be equally beneficial. Using anonymous functions, you can achieve this as follows:
-- Sort a table in descending order table.sort(myTable, function(a, b) return a>b end)
Combining Tables
There will be times when you may need to combine tables. Lua’s table library provides a handy function called `concat` which helps you achieve exactly that.
-- Combine tables local combinedTable = table.concat(myTable, mySecondTable)
Just remember that this function only works on tables with numeric indexes.
Finding the Size of a Table
Unsure about the size of the table? Forget the guesswork! Use the `#` operator in Lua to immediately get the size of your table.
-- Get the size of a table local tableSize = #myTable
Looping Through Tables
If you need to loop through each element in a table, you can use pairs (for key-value pairs) or ipairs (for integer-indexed tables).
-- Loop through a table for key, value in pairs(myTable) do print(key, value) end
-- Loop through an integer-indexed table for i, value in ipairs(myTable) do print(i, value) end
Wrapping Up
The Lua table library is your handy toolkit for managing data structures in Lua programming. From creating and managing tables to looping through each key-value pair, the table library has you covered. Plus, the syntax is straightforward and relatively easy to learn so you can be tinkering with complex data sets or game mechanics in no time. And the skills you develop here will translate easily into your next game development or coding project, making Lua a great place to start or continue your coding adventures.
Remember, we at Zenva believe in learning by doing. So the best way to master the Lua table library is by practical application. Happy coding!
More on Manipulating Tables
Let’s dive deeper into the ocean of the Lua table library. We’ll explore more functions that you can utilize to manage and manipulate your tables more efficiently.
Checking for an Element in a Table
The Lua table library does not contain a built-in function for checking whether a certain element exists in a table. But, with clever use of loops, we can still achieve this. Observe:
function table.contains(table, element) for _, value in pairs(table) do if value == element then return true end end return false end -- Check if 'Zenva' exists in the table if table.contains(myTable, 'Zenva') then print('Zenva is in the table!') end
Counting Number of Occurrences in a Table
Again, Lua table library doesn’t offer a built-in function for this purpose, but who said we couldn’t make our own?
function table.count(table, item) local count = 0 for _, value in pairs(table) do if value == item then count = count + 1 end end return count end -- Count the occurrences of 'Zenva' in the table local count = table.count(myTable, 'Zenva') print('Zenva appears ' .. count .. ' times.')
Reversing a Table
In some scenarios, you may need to reverse your table. Here’s how you can do it:
function table.reverse(tbl) local size = #tbl local newTable = {} for i, v in ipairs(tbl) do newTable[(size - i) + 1] = v end return newTable end -- reverse the table local myReversedTable = table.reverse(myTable)
Cloning a Table
While tables in Lua are referenced, sometimes we may need to completely clone a table creating new references. Here’s one way to do it:
function table.clone(org) return {table.unpack(org)} end -- Clone myTable local clonedTable = table.clone(myTable)
As you can see, while Lua table library offers functions that can help you in basic and intermediate tasks, it doesn’t cover more complex tasks. So, having the ability to write such functions for complex problems is a great skill to have. Don’t worry if you don’t understand them all right now, with practice and time you’ll be proficient at creating your solutions.
Carrying the Journey Forward
Now that you’ve mastered the intricacies of the Lua table library, your journey is only just beginning. There’s a galaxy of knowledge out there waiting to be explored, and Lua is but one star in that universe. From here, you can continue the voyage of discovery by diving in deeper, scripting more complex programs, or even experimenting with integrating Lua tables into games – the world is yours to code.
The realm of Roblox provides an excellent playground to test and apply your newfound knowledge. Roblox, a popular platform used by millions of players and developers, uses Lua as its scripting language. Game developers using Roblox can testify to the vital role Lua tables play in their creations, making this the perfect next step on your coding journey.
If Roblox game creation sounds exciting, we encourage you to check out our Roblox Game Development Mini-Degree. It’s an extensive set of courses covering not just the basics but also advanced concepts in game creation using Roblox Studio and Lua. With a wealth of topics ranging from 3D level creation to multiplayer combat and leaderboards, you can take your learning and application to the next level in an accessible and flexible manner.
Alternatively, for a wider range of skills and topics in Roblox, feel free to explore our ever-expanding collection of Roblox game development courses.
Remember, programming is a skill that gets better with practice – so keep creating, keep coding, and most importantly, keep learning. After all, with Zenva, from beginner to professional – we’ll be right there with you. To infinity, and beyond!
Conclusion
Realm of game development or the world of efficient problem-solving with data structures – Lua table library will pave the way for you. There’s immense joy and satisfaction to be derived from the act of creation – the ability to build something out of nothing but ideas and lines of code. We at Zenva firmly believe that anyone can code and create personal quests, battle scenes, or splendid simulations. With the power of Lua table library and our dedication towards quality learning, you are all set for a journey filled with code, creativity, and thrilling challenges.
Are you ready to venture into a dynamic world where codes bring imaginations alive? Seize the chance to progress your coding journey with our Roblox Game Development Mini-Degree. Set off on a quest filled with knowledge and application of Lua, and watch as you transform from being a coding learner into a confident creator. Safe journey, adventurer, we’re excited to see where your path takes you next!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
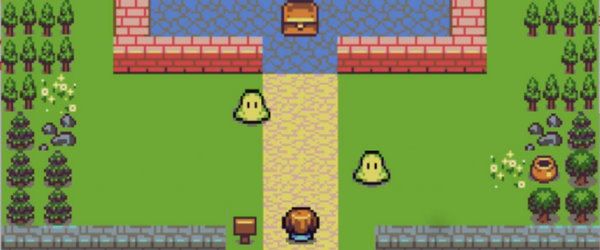
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.