Welcome to our interactive learning tutorial that dips your toes into the exciting world of Roblox raycasting. With this comprehensive guide, we aim to demystify this seemingly complex concept and simplify it into bite-sized, easy-to-understand content laced with engaging, clear-cut examples.
Table of contents
Understanding Roblox Raycasting
Roblox raycasting is a technique used within the Roblox game development environment to detect intersections between lines (rays) and objects within the game space. It’s a powerful tool that enables a variety of fascinating actions and interactions in your Roblox games.
Why Learn Roblox Raycasting?
Mastering Roblox raycasting opens up a plethora of possibilities in game logic and mechanics. This includes detection and tracking of entities, line of sight implementations, environmental scanning, and much more.
Moreover, learning it can enhance your problem-solving skills and encourage innovative thinking when designing dynamic game play interactions and mechanics.
Applying Roblox Raycasting
Concrete applications of Roblox raycasting range from creating natural light effects to designing dynamic aiming and shooting methods in action games.
Because of its immense potential to shape and manipulate game mechanics, learning this tool is highly instrumental in creating immersive gaming experiences.
Getting Started with Roblox Raycasting
Before we dive into code examples, it’s critical to understand the key components that make up a ray in Roblox. The primary element is the origin of the ray (where it starts in the workspace), and the secondary element is the direction of the ray (where it points).
Let’s see how to create a simple ray using the Ray.new() function:
local origin = Vector3.new(0, 0, 0) local direction = Vector3.new(1, 0, 0) local ray = Ray.new(origin, direction)
The above code snippet creates a new ray that originates at the position (0,0,0) and points along the x-axis.
Raycasting a Ray with Workspace:Raycast()
Once you’ve created your ray, you can cast it into your game environment using Workspace:Raycast(). This function returns the first BasePart that the ray intersects, or nil if the ray doesn’t intersect any BaseParts.
local intersection = game.Workspace:Raycast(ray.Origin, ray.Direction * raycastDistance) if intersection then print("Ray intersected with: " .. intersection.Instance.Name) else print("No intersection") end
This example prints the first object the ray intersects with, or “No intersection” if it doesn’t intersect with anything.
Adjusting Ray Direction
You can alter the direction of your ray by modifying the components of the direction vector. For example, let’s change our ray to point upwards:
local direction = Vector3.new(0, 1, 0) local ray = Ray.new(origin, direction)
This revised ray now points in the positive y-direction, which is upwards in the Roblox environment.
Handling Raycast Result
Workspace:Raycast() returns a RaycastResult instance. This instance includes the point of intersection, the normal at that point, the material of the intersected object, and the instance of the intersected object itself.
local intersection = game.Workspace:Raycast(ray.Origin, ray.Direction * raycastDistance) if intersection then print("Point: " .. tostring(intersection.Position)) print("Normal: " .. tostring(intersection.Normal)) print("Material: " .. tostring(intersection.Material)) print("Instance: " .. intersection.Instance.Name) end
The above example provides more detail about the intersection point, such as its exact location and the material of the object it intersects with.
Finding Multiple Intersections with Raycast
Up until now, we’ve only been examining the first object our ray intersects with. However, often we might need to know all objects that a ray intersects.
For finding multiple intersections, we can use the Workspace:RaycastAll(). Let’s take a look at a code snippet using RaycastAll:
local intersections = game.Workspace:RaycastAll(ray.Origin, ray.Direction * raycastDistance) for _, intersection in ipairs(intersections) do print("Ray intersected with: " .. intersection.Instance.Name) end
This example returns a table of all intersected instances in the ray’s path and prints all of them to the console.
Handling Non-Intersection Cases
In some cases, our ray may not intersect with any objects at all. By identifying these cases, we can avoid errors in our scripts and possibly add specific logic for empty intersections.
Handle this scenario by introducing a conditional check, as shown:
local intersection = game.Workspace:Raycast(ray.Origin, ray.Direction * raycastDistance) if intersection then print("Ray intersected with: " .. intersection.Instance.Name) else print("Ray did not intersect with any objects.") end
Filtering Specific Objects
Sometimes we want our ray to ignore certain objects or object types. In Roblox, we can achieve this by passing a table of objects we want to ignore to the Workspace:Raycast() method.
local origin = Vector3.new(0, 0, 0) local direction = Vector3.new(1, 0, 0) local ignoreList = { game.Workspace.IgnoreMePart, game.Workspace.IgnoreMeTooPart } local ray = Ray.new(origin, direction) local intersection = game.Workspace:Raycast(ray.Origin, ray.Direction * raycastDistance, ignoreList) if intersection then print("Ray intersected with: " .. intersection.Instance.Name) end
In this example, the ray would ignore any intersections with the parts “IgnoreMePart” and “IgnoreMeTooPart”.
Using Raycasting for Game Mechanics
Let’s now use Roblox raycasting in a real-world scenario: creating a laser gun mechanic in a game. Here’s an example of how you might achieve this:
local laserGunPart = game.Workspace.LaserGunPart function shootLaser() local origin = laserGunPart.Position local direction = laserGunPart.Orientation local ray = Ray.new(origin, direction) local intersection = game.Workspace:Raycast(ray.Origin, ray.Direction * raycastDistance) if intersection then print("Laser hit: " .. intersection.Instance.Name) end end shootLaser()
This script creates a ray that originates from the position of the laserGunPart and points in the direction the laserGunPart is facing. When the laserGun is fired, it prints the first object it hit.
As this guide reveals, Roblox raycasting is an incredibly versatile tool that helps unlock the full potential of your Roblox game development journey. Mastering raycasting means opening up the limitless possibilities of crafting interactive, immersive, and thrilling games for your audience.
Finding Closest Instances with Raycast
There are instances where you may need to find the closest object that a ray could potentially intersect with, even if the direct ray trajectory does not intersect with anything. This could be useful for scenarios such as proximity-based triggering mechanisms in games.
Here’s how you do just that:
local origin = Vector3.new(0,0,0) local direction = Vector3.new(1,0,0) local function closestIntersection(ray) local closest = nil local shortestDistance = math.huge for _, instance in ipairs(game.Workspace:GetChildren()) do local intersection = instance:FindPartOnRay(ray) if intersection then local distance = (ray.Origin - intersection).Magnitude if distance < shortestDistance then shortestDistance = distance closest = instance end end end return closest end local ray = Ray.new(origin, direction) local closest = closestIntersection(ray) if closest then print("Closest Intersected Instance: " .. closest.Name) end
This script systematically checks all the objects in the workspace for potential ray intersections, and stores the closest intersected instance.
Using Raycasting for Collision Detection
Raycasting can also play a crucial role in collision detection in Roblox games. Suppose you want to construct a mechanism where a player can only open a door upon making direct contact with it, you can handle this via raycasting in the following manner:
local player = game.Workspace.Player local doorPart = game.Workspace.DoorPart local doorOpen = false while true do -- Create a ray from player to door local origin = player.Position local direction = (doorPart.Position - origin) local raycastDistance = direction.Magnitude direction = direction.Unit local ray = Ray.new(origin, direction) local intersection = game.Workspace:Raycast(ray.Origin, ray.Direction * raycastDistance) -- If the ray intersects with the door, open it if intersection and intersection.Instance == doorPart and not doorOpen then doorOpen = true doorPart.Transparency = 0.5 print("Door opened!") -- If the ray no longer intersects with the door, close it elseif doorOpen and (not intersection or intersection.Instance ~= doorPart) then doorOpen = false doorPart.Transparency = 0 print("Door closed!") end wait(0.1) end
The script above continuously checks if the player’s position creates a ray that intersects with the door. If it does, the door opens by increasing the transparency of the doorPart.
Once the player moves and the ray is no longer intersecting with the door, it closes by returning the doorPart’s transparency back to its original state.
Helpful Tips:
When carrying out raycasting practices, it’s helpful to remember the following pointers:
- Always verify if an intersection exists before utilizing the intersection data.
- Distance is measured in studs, the standard Roblox unit of measurement.
- Keep in mind that rays don’t have to start within the boundaries of the workspace.
- Raycasting does not detect BaseParts that have the CanCollide property set to false.
Equipped with this robust understanding of Roblox raycasting, you’re now ready to experiment and innovate. Whether it’s creating light effects, shooting methods, detecting collisions, or revamping your game mechanics, the possibilities are endless. So, set on your journey, fellow developers, and explore the incredible world of Roblox game creation with us.
Where to Go Next?
Armed with the robust knowledge of Roblox raycasting, you’re now better equipped to explore the thrilling world of Roblox game development. But the journey doesn’t end here. To dive deeper and master the art of Roblox game creation, consider taking your learning journey a step further.
We here at Zenva encourage you to check out our Roblox Game Development Mini-Degree, a comprehensive set of courses that covers various genres like obstacle courses, melee combat games, and FPS games. It offers practical skills, in-browser practise coding, challenges, quizzes, and helps you build a professional portfolio. Perfectly suited for beginners with no coding experience, our courses are designed to be flexible and accessible on all modern devices.
For an even broader exploration of Roblox game development and to further hone your skills, do visit our collection of Roblox courses. At Zenva, we offer a variety of programming, game development, and AI courses – from beginners to professionals. Start your journey with us today and pave your path towards becoming a skilled game developer.
Conclusion
There’s no denying Roblox raycasting plays a vital part in creating fascinating, interactive, and immersive gaming experiences. From simulating realistic light patterns to crafting compelling mechanics such as proximity-based triggering mechanisms, raycasting offers limitless potential to elevate your Roblox games.
Are you excited to further harness the power of Roblox and become proficient in game development? We invite you to join us in our Roblox Game Development Mini-Degree. Our comprehensive curriculum, designed for a diverse range of learners, guarantees you an enriching learning experience. Equip yourself with the tools of modern game design and scripting with us at Zenva today!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
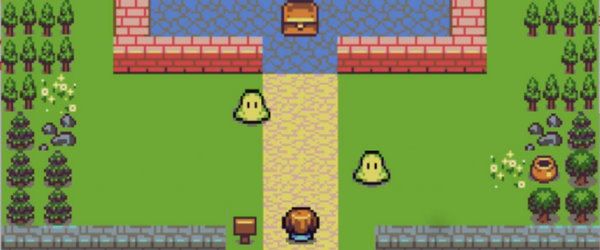
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.