Welcome to an exciting journey into the world of C# programming! C# is a powerful, versatile programming language that forms the backbone of many applications, and is particularly popular in the realm of game development. This tutorial will unlock your path to understanding and mastering C#, opening up an expansive world of coding opportunities.
Table of contents
What is C#?
C# (pronounced as “See Sharp”) is a modern, type-safe, object-oriented programming language developed by Microsoft. It is part of the .NET framework, and is widely used to build Windows desktop applications, web applications, and video games, particularly those on the Unity game engine.
What is C# Used For?
One of the biggest uses of C# is in game development, as it is used as the primary programming language within Unity, one of the leading game creation engines in the world. C# is also widely used to build professional business applications, including Windows desktop applications and web applications.
Why Should You Learn C#?
Learning C# opens up a wealth of opportunities. If you’re considering venturing into game development, mastering C# is a necessary step and a valuable skill in the industry. In addition, C# is high in demand in the job market, and knowing it will surely give you a competitive edge. Its robustness and flexibility make it a great choice for all kinds of applications – from manipulating databases to building stunning game mechanics.
Getting Started with C#
Before writing C# code, we’ll need an environment to write and run the code. We can leverage a tool, like Visual Studio Code or the full-blown Visual Studio IDE (Integrated Development Environment) for this purpose. Set up your IDE, and you are ready to begin!
Your First C# Program
Let’s start with the traditional “Hello World” program. This simple code demonstrates how to display output in C#. We’ll use the Console.WriteLine() method:
using System; class Program { static void Main(string[] args) { Console.WriteLine("Hello, World!"); } }
The “using System;” directive allows us to use classes from the System namespace. The Console.WriteLine() method is used to output lines to the console.
Data Types in C#
The main data types in C# are:
- int: stores whole numbers (e.g., 10, 20, -5).
- double: stores floating-point numbers (e.g., 10.5, -1.25).
- char: stores single characters (e.g., ‘a’, ‘B’, ‘1’).
- string: stores sequences of characters (e.g., “hello”, “C# tutorial”).
- bool: stores boolean values (either true or false).
int number = 10; double decimalNumber = 5.2; char letter = 'A'; string name = "C# Tutorial"; bool isCSharpFun = true;
Operators in C#
C# incorporates a wide range of operators that allow us to perform various operations on variables and constants. These operators include arithmetic operators, comparison operators, logical operators, and more. Here are some examples:
int x = 10; int y = 20; // Addition int sum = x + y; // 30 // Subtraction int difference = x - y; // -10 // Division int quotient = y / x; // 2 // Modulo int remainder = y % x; // 0
The result of the operations will be stored in the respective variables: sum, difference, quotient, and remainder.
Conditional Statements in C#
Conditional statements help us make decisions based on certain conditions. The two fundamental conditional statements in C# are if and else.
int x = 10; int y = 20; if (x > y) { Console.WriteLine("x is greater than y"); } else { Console.WriteLine("x is less than y"); }
The if statement checks whether the given condition (“x > y” in this case) is true. If true, it will execute the code within its block. If the condition is false, it will execute the code in the else block.
Loops in C#
Loops are fundamental to any programming language, including C#. We offer two primary types of loops – the for loop and the while loop.
// For loop for (int i = 0; i < 5; i++) { Console.WriteLine(i); } // While loop int i = 0; while (i < 5) { Console.WriteLine(i); i++; }
Both loops will print numbers 0 through 4 to the console. The for loop is contrived for situations where the number of iterations is known in advance, whereas the while loop is preferred in situations where the number of iterations is unknown.
Functions in C#
Functions are blocks of code that carry out a specific task. They can take inputs and return a result. Here’s a simple function in C#:
void SayHello() { Console.WriteLine("Hello C#"); } // Call the function SayHello();
This SayHello() function takes no input, performs an action (prints “Hello C#”), and returns no output.
More generally, functions can return values and take parameters, much like this example:
int Add(int x, int y) { return x + y; } // Call the function int sum = Add(5, 10); Console.WriteLine(sum); // Prints: 15
This function, Add, accepts two inputs x and y, adds them together, and returns the result. These examples demonstrate the basics of functions in C#, but their potential extends far beyond, giving you the tools to manage and structure your code effectively.
Arrays in C#
Arrays are used to store multiple values in a single variable. Let’s look at a simple example:
int[] numbers = {10, 20, 30, 40, 50}; foreach(int number in numbers) { Console.WriteLine(number); }
This will print all numbers in the array. C# arrays are zero-indexed, meaning the first element resides at index 0. Thus, ‘numbers[2]’ refers to the value 30.
Classes and Objects in C#
One of the central concepts in C# is Object-Oriented Programming (OOP). In OOP, we organize our code around objects and data, rather than logic and procedures. Classes and Objects are pivotal to this approach.
A Class is like a blueprint for creating objects. An Object is an instance of a class. Let’s examine a simple class:
public class Bicycle { public string color; } Bicycle myBike = new Bicycle(); myBike.color = "Blue"; Console.WriteLine(myBike.color); // Prints: Blue
We define a Bicycle class with a public member variable color. Then, we create an instance of the Bicycle class (an object), set its color to “Blue,” and then print that color.
Exceptions in C#
Exceptions represent errors that occur during the execution of the program. Let’s take a glance at a basic exception handling in C#.
try { int x = 10; int y = 0; int result = x / y; // This will cause a DivideByZeroException } catch (DivideByZeroException e) { Console.WriteLine("Cannot divide by zero"); }
This C# code tries to divide an integer by zero, which is not allowed and causes a DivideByZeroException. We catch this exception and print a friendly error message instead of letting the program crash.
Conclusion
We have now covered some of the most fundamental aspects of C# programming. You are now better equipped to embark on your game creation journey with a solid grasp of C# basics. We encourage you to continue diving deeper, practicing, and exploring more complex projects. At Zenva, we are committed to offering comprehensive learning materials that equip you to be a capable, creative game developer using the power of C#.
Continue Your C# Journey With Unity
Now that you’ve had a taste of C# programming, it’s time to channel your newly acquired skills into something extraordinary, such as creating your very own games with Unity! At Zenva, we provide a range of educational materials, from beginner to professional, to help you continue learning and apply your coding skills in a wide array of applications.
To help you build on your C# skills, we invite you to explore our Unity Game Development Mini-Degree. This comprehensive program will guide you through creating games using Unity, preferred globally by both AAA and indie developers. You’ll delve into various aspects of game development, such as game mechanics, animation, and audio effects, and carry out hands-on projects to give life to your ideas.
Moving beyond, should you wish to broaden your horizon, feel free to explore our entire collection of Unity courses. Whichever path you take, we at Zenva stand ready to provide support and high-quality content to transform you from a beginner into a professional. Let’s unlock the power of code together!
Conclusion
The journey into the world of C# and game development is exciting and full of opportunities for creativity. It’s truly a thrilling experience to use your coding skills to bring your unique game ideas to life, to troubleshoot and overcome challenges, and to continually improve and build upon your creations.
C# is a powerful tool in your game development arsenal. Mastering it will open countless doors for you. We at Zenva are committed to providing comprehensive, high-quality courses that will cover everything you need to become a proficient game developer. Ready to take the plunge? Dive into our Unity Game Development Mini-Degree and begin crafting your own games today!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
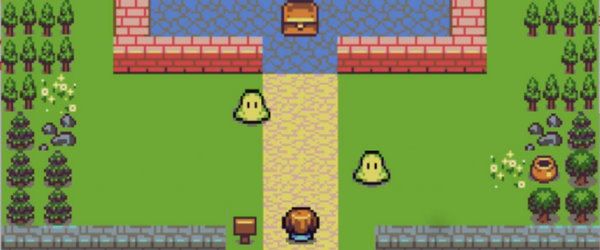
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.