Welcome to Roblox leaderboard scripting! In this tutorial, we’re taking an in-depth look into how to design and implement leaderboards on Roblox, an essential tool for enhancing players’ gameplay experience. If you’ve been on the lookout for ways to motivate players to give their best in your game or to spice up the competition level, then you’re in the right place!
Table of contents
What Is Roblox Leaderboard Scripting?
Roblox leaderboard scripting refers to using Lua, Roblox’s in-built scripting language, to design and implement a leaderboard in a Roblox game. Leaderboards are often used in games to track and display players’ scores or accomplishments, fostering a competitive environment and embedding a sense of achievement.
Why Should I Learn It?
Being able to script your own leaderboard in Roblox equips you with an integral asset for your game creation toolbox. By encouraging competition, leaderboards keep players engaged and coming back for more. Plus, designing your own leaderboard allows for a high degree of customization, meaning you can tailor it to fit your game’s specific needs and style.
Furthermore, as you learn scripting for leaderboards, you naturally enhance your proficiency in Lua, a valuable skill set in the world of game creation. This skill adds an extra layer of interactivity for your players, enriching their in-game experience and increasing the potential of your game’s success in the Roblox universe.
Setting Up Your Leaderboard
First things first, you need to set up your leaderboard. To do this, you have to write a script that will create a leaderboard for each player that joins your game. Follow the steps below:
Ensure there is a ServerScriptService in your Roblox environment. This is where your script will live.
-- Check for ServerScriptService local serverScriptService = game:GetService('ServerScriptService')
Next, insert a new script into the ServerScriptService. You can name this script ‘leaderboardScript’ for clarity.
-- Create new script in ServerScriptService local leaderboardScript = Instance.new('Script') leaderboardScript.Name = "leaderboardScript" leaderboardScript.Parent = serverScriptService
Adding A Player Points System
With your leaderboard set, you can now add a player points tracking system. Let’s add a feature to track each player’s points:
Firstly, create a function to add a new player to the leaderboard. Each time a new player joins, this function will create a leaderboard for them:
-- Function to add new player to leaderboard function addPlayerToLeaderboard(player) local leaderstats = Instance.new('Folder') leaderstats.Name = 'leaderstats' leaderstats.Parent = player local points = Instance.new('IntValue') points.Name = 'Points' points.Parent = leaderstats points.Value = 0 end -- Connect function to PlayerAdded event game.Players.PlayerAdded:Connect(addPlayerToLeaderboard)
The above script creates a new function addPlayerToLeaderboard where it first creates a new folder named ‘leaderstats’. In that folder, it creates a new IntValue named ‘Points’ and sets the initial value to 0.
It then connects the function to the PlayerAdded event, which means the function will run each time a new player joins the game.
Updating the Leaderboard
Now, if you want to increase a player’s points, you just need to access the ‘Points’ IntValue in the player’s leaderboard and update the value. Here’s how:
-- Function to add points function addPoints(player, points) player.leaderstats.Points.Value = player.leaderstats.Points.Value + points end
This script creates a new function addPoints that takes two arguments, the player and the number of points to add. It then reads the player’s current points, adds the new points to this total, and assigns the result back to the player’s points.
Now you can call this function whenever you want to add points to a player:
-- Call the function to add points to a player addPoints(game.Players["PlayerName"], 10)
Replace “PlayerName” with the actual name of the player you want to award points to.
Adding Other Leaderboard Features
Once you have the basics of Roblox leaderboard scripting down, you can start adding unique features that fit the design of your game. Here are a few examples:
Tracking Player Properties
If your game involves more than just scoring points, you can add other values to your leaderboard. Let’s add a value to track the player’s health:
function addPlayerToLeaderboard(player) local leaderstats = Instance.new('Folder') leaderstats.Name = 'leaderstats' leaderstats.Parent = player local points = Instance.new('IntValue') points.Name = 'Points' points.Parent = leaderstats points.Value = 0 local health = Instance.new('IntValue') health.Name = 'Health' health.Parent = leaderstats health.Value = 100 -- can set this to whatever initial health you prefer end
Now, when a new player joins your game, they will have a leaderboard tracking both their points and health.
You can update this value the same way you updated points:
-- Function to reduce health function reduceHealth(player, damage) player.leaderstats.Health.Value = player.leaderstats.Health.Value - damage end
Creating a Persistent Leaderboard
If you want your leaderboard to store player data between game sessions, then you will need to use Roblox’s built-in data store. Here’s how you can set this up:
-- Get the DataStore service local DataStoreService = game:GetService("DataStoreService") local myDataStore = DataStoreService:GetDataStore("MyDataStore") -- Add data store to addPlayerToLeaderboard function function addPlayerToLeaderboard(player) local leaderstats = Instance.new('Folder') leaderstats.Name = 'leaderstats' leaderstats.Parent = player local points = Instance.new('IntValue') points.Name = 'Points' points.Parent = leaderstats -- Load player data from data store local data = myDataStore:GetAsync(tostring(player.UserId)) if data then points.Value = data.Points else points.Value = 0 end end -- Save data to data store when player leaves game game.Players.PlayerRemoving:Connect(function(player) local data = { Points = player.leaderstats.Points.Value, } myDataStore:SetAsync(tostring(player.UserId), data) end)
This script sets up a data store that retrieves saved data when a player joins and stores player data when they leave. Note that data stored can persist across games, servers, and even game sessions!
Handling Multiple Leaderboards
In some cases, you might want multiple leaderboards for different game modes or maps. Creating multiple leaderboards is as easy as creating multiple ‘leaderstats’ folders and setting their parent to the player as shown above.
For example, you could create one leaderboard for a campaign mode and another for a survival mode:
function addPlayerToLeaderboard(player) -- set up campaign leaderboard local campaignStats = Instance.new('Folder') campaignStats.Name = 'CampaignStats' campaignStats.Parent = player local campaignPoints = Instance.new('IntValue') campaignPoints.Name = 'Points' campaignPoints.Parent = campaignStats campaignPoints.Value = 0 -- set up survival leaderboard local survivalStats = Instance.new('Folder') survivalStats.Name = 'SurvivalStats' survivalStats.Parent = player local survivalPoints = Instance.new('IntValue') survivalPoints.Name = 'Points' survivalPoints.Parent = survivalStats survivalPoints.Value = 0 end
This creates two different leaderboards for the player: one for the campaign mode and one for the survival mode. You can customize these stats to suit the needs of your particular game modes.
Displaying the Leaderboard
After setting up the leaderboards, players need to be able to see their stats. Here is how you can script a basic GUI to display a player’s points:
-- Function to update a player's points on GUI function updatePointsGUI(player) local pointsGUI = game.StarterGui:WaitForChild("PointsGUI") local pointsText = pointsGUI:WaitForChild("PointsText") -- loop to keep the GUI updated while true do pointsText.Text = "Points: "..player.leaderstats.Points.Value wait() -- this makes the loop wait for 1 second before repeating end end -- Connect the function to PlayerAdded event game.Players.PlayerAdded:Connect(function(player) player.CharacterAdded:Wait() updatePointsGUI(player) end)
The above script sets up a loop to consistently update the player’s points in the GUI. The “wait()” call at the end of the loop ensures a one-second delay before the panel updates again, reducing the computation needed to keep the GUI updated.
Incorporating Leaderboards into Gameplay
Let’s say you have a game where players score points by collecting items. Here, you can integrate the leaderboard to update whenever a player collects an item:
-- Function to add points function addPoints(player, points) player.leaderstats.Points.Value = player.leaderstats.Points.Value + points end -- Function to handle a player touching an item function handleTouchItem(player, points) -- only award points if the object touched is an item touched.Touched:Connect(function(part) if part.Parent == player.Character then addPoints(player, points) -- destroy item after collection item:Destroy() end end) end
This script adds a handleTouchItem function that checks if a player character has touched an item. When this happens, the game adds points to the player’s score and then destroys the item.
Challenging Players with Special Conditions
If you want to challenge your players further, you can set special conditions that can affect their leaderboard rankings. For example, you could create diminishing returns by reducing the points gained each time a player collects an item:
-- Function to add points with diminishing returns function addPoints(player, points) -- reduce points by half each time player.leaderstats.Points.Value = player.leaderstats.Points.Value + (points / 2) end
This script modifies the addPoints function so that each time it’s called, the points added to the player’s score become half of what they were the last time.
Sorting the Leaderboard
Your leaderboard may contain scores of hundreds of players. Here’s how you can sort the leaderboard based on player scores:
function sortLeaderboard() -- Get a table of all players local allPlayers = game.Players:GetPlayers() -- Sort the table based on player points table.sort(allPlayers, function(a, b) return a.leaderstats.Points.Value > b.leaderstats.Points.Value end) return allPlayers end
This function fetches all current players, sorts them based on their points from highest to lowest, and returns the sorted list of players. Now, you can display the sorted leaderboard to the players or use it for any other purposes.
Where to Go Next?
Now that you’ve gotten a taste of Roblox leaderboard scripting, there are limitless possibilities for how you can continue to grow and improve. It takes practice to become a proficient game developer, and what better way to learn than by doing? Try implementing leaderboards with different features in your Roblox games for a broader learning experience.
If you’re hungry for more knowledge, we encourage you to explore our wide variety of courses offered at Zenva Academy. From programming to game development and AI, we cater to learners at all levels – from beginners to professionals. Our robust collection of over 250 courses caters to those who want to hone their skills, create engaging games, and get certified.
Want to dive deeper into the world of Roblox game creation? Consider checking out our Roblox Game Development Mini-Degree. This comprehensive collection of courses covers game creation using Roblox Studio and Lua across various genres, equipping you with critical skills like multiplayer functionality and leaderboard design. Catering to both beginners and experienced developers, this mini-degree not only helps you build a professional portfolio but also equips you with in-demand skills for a promising career in game development. For a broader selection of courses, feel free to explore our collection of Roblox courses.
Remember, the journey of a thousand miles begins with a single step. So continue coding, keep gaming, and never stop learning!
Conclusion
There you have it – a comprehensive guide to creating, implementing, and enhancing leaderboards in Roblox! We hope this tutorial has sparked your imagination and enthusiasm to create engaging and competitive gaming experiences for your players.
No matter your game development journey – if you’ve just started or have been developing for years, there’s always something more to learn. Keep up the momentum! Continue exploring the vast domain of Roblox game creation with our Roblox Game Development Mini-Degree. At Zenva, we’re always with you at every step. Keep scripting, keep gaming, and most importantly – keep learning!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
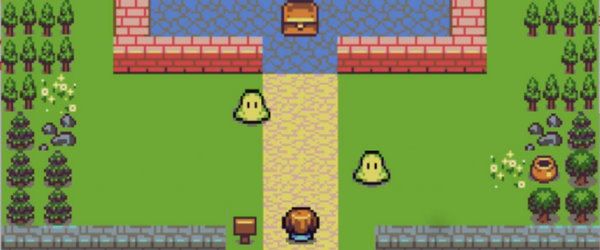
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.