In the dynamic world of game development, landing your hands on the right tools and mechanisms can open up a Pandora’s box of possibilities. Roblox, with its event handling feature, does exactly that for game developers of all skill levels. Designed to captivate and inspire, this guide will help you delve into the fundamentals of Roblox event handling, making your journey smoother and more insightful.
Table of contents
What is Roblox Event Handling?
Roblox event handling is the mechanism by which Roblox Lua scripts respond to certain actions (events) occurring within a game. It’s an essential aspect of game development in Roblox, allowing developers to dictate how a game reacts to specific triggers, player actions, or scenarios.
What is it for?
Event handling is the backbone that orchestrates the interaction between the player and the game environment. It gives life to objects by enabling them to respond to player input and other game events, such as when a button is clicked, a collision occurs, or simply when a game starts or ends.
Why should I learn it?
Learning event handling in Roblox provides you with a powerful scripting tool, facilitating unique game mechanics and player interactions. It will empower your Roblox development skills, allowing you to create more complex and interactive games that keep players engaged. Let’s break this down with useful code examples and explanations.
Basics of Event Handling in Roblox
Understanding the basics of event handling often begins with getting acquainted with the `event:connect()` function. This function allows developers to associate a particular event with a function that will be executed when the event occurs. Here is a simple illustration:
local part = game.Workspace.Part local function onTouch(otherPart) print("Part was touched by " .. otherPart.Name) end part.Touched:Connect(onTouch)
In this code snippet, we have a simple game part in the workspace. When this part is touched by another part, it triggers the `Touched` event, and our function `onTouch()` is then executed, which prints a message to the console.
Working with Different Types of Events
Roblox supports a wide array of event types, including those triggered by user actions. Getting to grips with these types boosts your proficiency in designing diverse game dynamics. Let’s explore some relevant code examples:
1. **Click Detector Event**: This event occurs when a player clicks on part tied with a Click Detector.
local part = game.Workspace.Part local clickDetector = Instance.new('ClickDetector', part) local function onClick() print('Part was clicked!') end clickDetector.MouseClick:Connect(onClick)
This code leverages a `ClickDetector` object introduced into our game part. When the part is clicked by a player, it activates the `MouseClick` event and the function `onClick()` is executed, outputting a message to the console.
2. **Player Added Event**: This event triggers when a new player joins the game.
local function onPlayerAdded(player) print(player.Name .. ' has joined the game') end game.Players.PlayerAdded:Connect(onPlayerAdded)
In this snippet, the `PlayerAdded` event is coupled with a `onPlayerAdded()` function. Whenever a player joins the game, a welcome message containing the player’s name is printed.
3. **KeyPress Event**: This event occurs when the player presses a specific key.
local player = game.Players.LocalPlayer player:GetMouse().KeyDown:connect(function(key) print('Key ' .. key .. ' was pressed') end)
Here, a key press from the player triggers the `KeyDown` event, following which the code prints the key pressed. This method of event handling allows developers to associate different game dynamics with different key presses.
As you start delving into the myriad possibilities of event handlers in Roblox, you’ll find an empowering tool at your disposal, capable of revamping your game designs in more ways than one.
Deep Dive into Roblox Event Handling
The versatility of event handling in Roblox is virtually endless. Let’s explore more comprehensive examples of event handling, ranging from triggering game actions to handling player interactions.
Manipulating Game Objects through Events
1. **Changing Object Properties:**
Events can also be used to dynamically change the properties of in-game objects. Check out this example:
local part = game.Workspace.Part local clickDetector = Instance.new('ClickDetector', part) local function onClick() part.BrickColor = BrickColor.new('Bright red') end clickDetector.MouseClick:Connect(onClick)
In this script, clicking the part triggers the `MouseClick` event, which in turn changes the color of the part to bright red.
2. **Creating Objects On-the-Fly:**
We can use events to create new game objects on the fly. Here’s an interesting example:
local function onPlayerAdded(player) local leaderstats = Instance.new("Folder") leaderstats.Name = "leaderstats" leaderstats.Parent = player local gold = Instance.new("IntValue") gold.Name = "Gold" gold.Value = 0 gold.Parent = leaderstats end game.Players.PlayerAdded:Connect(onPlayerAdded)
In this example, the `onPlayerAdded()` function creates a new Leaderstats folder with a Gold value for each player as they join the game.
Handling Dynamic Player Interactions
1. **Chat Message Event:**
You can handle events related to player chat messages:
local function onChat(player, message) print(player.Name .. ' said: ' .. message) end game.Players.PlayerChatted:Connect(onChat)
In this code, the `PlayerChatted` event will trigger the `onChat` function whenever a player sends a chat message, printing it out.
2. **Character Added Event:**
To deal with events related to a player’s character spawn, use `CharacterAdded`:
local function onCharAdded(character) print('A new character has been added.') end game.Players.PlayerAdded:Connect(function(player) player.CharacterAdded:Connect(onCharAdded) end)
Here, when a player spawns within the game, the `CharacterAdded` event fires, and the `onCharAdded` function executes, printing a message.
3. **Handling Team Changes:**
Similarly, you can handle team changes with the `TeamChange` event.
local function onTeamChange() print('Team has changed.') end game.Players.PlayerAdded:Connect(function(player) player.TeamChange:Connect(onTeamChange) end)
In this scenario, whenever a player switches teams, the `TeamChange` event is called, thus printing the provided message.
Getting your hands dirty with the practical implementation of events like these should give you a fair idea of Roblox’s versatile and dynamic event handling system. Remember, mastery comes from persistent practice. So, keep experimenting, exploring, and creating with Roblox Lua!
Unleashing Interactivity with Event Handling
As you start mastering different types of Roblox events, you can unlock the ability to create impressive interactive dynamics within your games. Let’s delve deeper.
1. **Triggering Animation:**
Event handlers can help initiate certain Roblox animations when an event occurs.
local clickDetector = Instance.new('ClickDetector', workspace.Part) local function animate() workspace.Part.Anchored = false workspace.Part.Velocity = Vector3.new(0, 50, 0) end clickDetector.MouseClick:Connect(animate)
In this code, clicking the game part triggers the `MouseClick` event resulting in animating the part to ascend upward.
2. **Touch Activated Sounds:**
We can associate sounds with the `Touched` event to make our game environment more plentiful.
local part = workspace.Part local sound = Instance.new('Sound', part) sound.SoundId = 'rbxassetid://301964312' local function playSound(otherPart) sound:Play() end part.Touched:Connect(playSound)
In this instance, when our game part is touched, it activates the `Touched` event, and our function `playSound()` is executed, playing the associated sound.
Managing Game Mechanics with Events
Event handling is particularly powerful when it comes to managing game mechanics. Levels, scoring, time limits, constraints – all of these can be managed through the clever use of events.
3. **Timer-Based Events:**
A common use of event handlers is to manage timer-based game mechanics.
local timer = 5 local function onTimerComplete() print('Timer is complete!') end while timer > 0 do wait(1) timer = timer - 1 end onTimerComplete()
This simple example prints a message to the console after a 5-second timer completes.
4. **Scoring Events:**
Managing scoring events is a typical utilization of event handlers.
local points = 0 local function onScore() points = points + 1 print('Score: ' .. points) end onScore() onScore() onScore()
The `onScore()` function is called each time a score event is supposed to occur, incrementing the score and printing the new score.
5. **Level-Based Events:**
Level-based mechanics can also be managed using event handlers.
local level = 1 local function nextLevel() level = level + 1 print('Moved to Level ' .. level) end nextLevel() nextLevel() nextLevel()
Here, the `nextLevel()` function is triggered each time the player progresses to a new level, increasing the level and printing out the new level number.
In conclusion, event handling in Roblox gives you an extraordinary ability to control and manage the interactivity and mechanics of your game. While the examples presented in this guide merely skim the surface of what event handlers are capable of, we hope they have given you a solid foundation to build your Roblox scripting knowledge upon. As with anything, practice makes perfect. Keep coding, experimenting, and learning!
Where to go Next with Your Roblox Adventures?
Having decoded the basics of Roblox event handling, you’re likely excited about the boundless possibilities at your disposal. Well, why stop here? Keep that momentum going! There’s a wealth of knowledge to be explored, many complexities to unravel, and limitless gaming dynamics waiting for you to bring them to life.
Zenva Academy, known for its top-notch online courses devoted to programming, game development, and AI, brings you the incredibly comprehensive Roblox Game Development Mini-Degree. A specially designed collection of courses that walk you through the realm of game creation using Roblox Studio and Lua, this Mini-Degree touches upon a wide array of game genres, including obstacle courses and FPS games. Incorporating 3D level creation, platforming mechanics, multiplayer combat and leaderboards, this flexible, high-paced learning format is suitable for beginners, as well seasoned developers, looking to polish their skills.
To cater to a broader range of learning requirements and interests, feel free to explore our diverse collection of Roblox-related courses. With Zenva Academy, your journey from novice to pro in your desired field is not only possible, but accelerated and enriched.
Keep coding, stay curious, and enjoy your journey into the fascinating world of Roblox game development!
Conclusion
Armed with the power and versatility of event handling in Roblox, the world of interactive and captivating gaming awaits your exploration. Your journey of learning and developing should always be thriving – a quest that can be exciting, enlightening, and empowering. At Zenva Academy, we are here to nurture your endeavors, providing you with high-quality, user-friendly learning paths to pave your way to becoming a sought-after game developer.
Enrich your learning experiences by applying these event handling skills in developing dynamic and engaging games in Roblox. Journey through our Roblox Game Development Mini-Degree, to give your game development career a strong kickstart, taking your creativity and technical skills to a whole new level. As you continue to experiment, create, and innovate, always remember that your passion and Zenva’s expertise are the ingredients to transforming your game development dreams into reality. Happy scripting!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
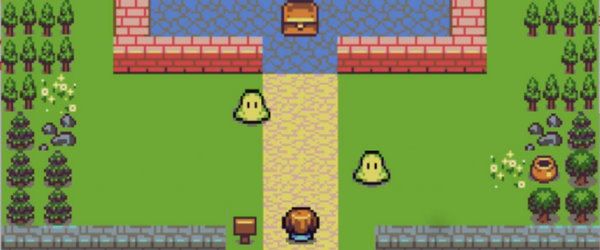
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.