Welcome to this exciting and comprehensive guide on Lua scripting. This tutorial will illuminate the ins and outs of Lua, a powerful, fast, and easy-to-use scripting language. With its lightweight yet robust capabilities, Lua has gained popularity in many sectors, including game development, web servers, and even embedded systems.
Table of contents
What is Lua?
Lua is a high-level, multi-paradigm programming language primarily designed for embedded use in applications. Its portability, extensibility, and simplicity have made it a language of choice for various fields.
What is It Used for?
In the gaming universe, Lua finds a large application. Its user-friendly nature presents game developers with a pathway to develop prototypes quickly. Lua finds extensive usage within game frameworks like Love2D and Roblox. In addition, it is in demand for web servers and embedded systems because of its efficiency.
Why Should I Learn Lua Scripting?
If you are interested in game development, extending applications, or even creating efficient web servers, then Lua is a language you should consider learning. Lua, with its soft learning curve, becomes an excellent place to start for beginners while offering experienced coders the speed and efficiency needed.
There’s no denying the rewarding experience and career opportunities that come with learning Lua. To thrive in this digital era, having Lua in your coding toolbox can be a significant advantage. Let’s dive deeper into the world of Lua scripting in the subsequent sections of this tutorial.
Lua Basics: Data Types and Variables
In Lua, there are eight basic data types: nil, boolean, number, string, userdata, function, thread, and table. Let’s look at some examples of these:
--nil local myValue print(myValue) -- prints 'nil' --boolean local iGameDev = true print(iGameDev) -- prints 'true' --number local students = 100 print(students) -- prints '100' --string local greet = "Hello, Zenva" print(greet) -- prints 'Hello, Zenva'
Furthermore, you can use the type() function to get the data type of a particular variable:
-- type function usage print(type(myValue)) -- prints 'nil' print(type(iGameDev)) -- prints 'boolean' print(type(students)) -- prints 'number' print(type(greet)) -- prints 'string'
Lua Basics: Functions
A function is a reusable block of code in Lua. They help to structure the code, make it more readable, and reduce repetition. Functions are defined using the keyword ‘function’ followed by the function name, i.e., function functionName(). Let’s look at some examples:
--function without parameters function greet() print("Hello, Zenva") end greet() -- calls the function and prints 'Hello, Zenva' --function with parameters function welcome(name) print("Welcome, " .. name) end welcome("GameDev") -- calls the function and prints 'Welcome, GameDev'
Lua Basics: Control Structures
Control structures direct the order of execution of the statements in a script. They include if, else, elseif, while, and for loops. Here are some examples:
-- if, else, elseif local score = 85 if score > 90 then print("Excellent!") elseif score > 60 then print("Good job!") else print("Try harder next time!") end -- while loop local i = 1 while i <= 5 do print(i) i = i + 1 end -- for loop for j = 1, 5 do print(j) end
Lua Basics: Tables
Tables in Lua are the only complex data type. They can store values of all types, including other tables. Lua uses tables to represent arrays, dictionaries, and even classes.
--table as array local fruits = {"Apple", "Banana", "Cherry"} for index, fruit in ipairs(fruits) do print("Fruit "..index..": "..fruit) end --table as dictionary local student = {name = "John", age = 21, grade = "A"} for key, value in pairs(student) do print(key..": "..value) end
Lua in Game Development
Due to its lightweight footprint and flexibility, Lua is extensively used in the game industry. For instance, the popular game development platform Roblox uses Lua as its core scripting language.
--Roblox Lua scripts typically look like the following: local player = game.Players.LocalPlayer local char = player.Character or player.CharacterAdded:Wait() local humanoid = char:WaitForChild("Humanoid") humanoid.WalkSpeed = 50
Concurrency in Lua: Coroutines
Lua supports concurrent programming through the use of coroutines. A coroutine is kind of like a thread; it’s a line of execution with its stack, its local variables, and its point of control.
-- creating a coroutine co = coroutine.create(function () print("Hi") end) print(co) -- prints: thread: 0x8071d98 -- running a coroutine coroutine.resume(co) -- prints: 'hi'
Using coroutines, developers can organize their code with the concept of cooperative multitasking where tasks yield control periodically or when idle.
Lua and C API
Lua has a powerful C API allowing the embedding of Lua into C applications and enabling C code to be called from Lua. The ability to extend Lua in such a way has made it ubiquitous in applications needing scripting capabilities.
/* Embedding Lua in a C application example */ #include #include #include int main (void) { char *lua_script = "return math.sqrt(144)"; lua_State *L = luaL_newstate(); luaL_openlibs(L); luaL_dostring(L, lua_script); double x = lua_tonumber(L,-1); printf("%f\n", x); lua_close(L); return 0; }
This script creates a Lua state, evaluates a script, retrieves the result from the Lua stack, and finally closes the Lua state.
Advanced Lua: Scripting and Modules
In Lua, a scripting file usually has the .lua extension. We can run Lua scripts in the terminal with the ‘lua’ command. For instance, we can create a script to print “Hello, Zenva”.
-- helloZenva.lua print("Hello, Zenva")
In the terminal, you would then execute:
lua helloZenva.lua
Lua also allows the use of modules, which are like libraries – they are simply script files containing functions that other scripts can utilize. Let’s look at an example of how to create and use modules in Lua:
-- creating a module named moduleExample.lua local M = {} function M.greet(name) return "Hello, " .. name end return M
You can access this module from another Lua script:
-- using moduleExample.lua local ME = require("moduleExample") print(ME.greet("Zenva")) -- prints 'Hello, Zenva'
The ‘require’ function is used to include modules. When it’s called, it checks if the module is already loaded. If not, it loads the module.
Using External Libraries in Lua
Lua can be extended with external libraries. The ‘luasocket’ library, for example, allows Lua scripts to use TCP/UDP sockets.
-- including luasocket in Lua script local socket = require("socket") --Creating a TCP client socket local tcp = assert(socket.tcp()) --Establishing TCP connection tcp:connect('localhost', 80); --Sending a request to the server tcp:send("GET / HTTP/1.0\r\n\r\n") -- Receiving the response and printing it while true do local s, status, partial = tcp:receive() print(s or partial) if status == "closed" then break end end
The above code will print the HTTP headers for the ‘localhost’ home page.
Error Handling in Lua
Errors in Lua can be handled using the ‘pcall’ (protected call) function. The first argument to pcall is the function we want to call, followed by any number of arguments to the function. ‘pcall’ returns true if no error occurred or false if an error occurred.
-- error handling using pcall local status, error = pcall(function () error({code=121}) end) print(error.code) -- prints '121'
It’s always good practice to include error handling in your Lua scripts to make debugging easier and improve overall code reliability.
Where to Go Next?
Congrats on finishing this comprehensive guide on Lua scripting! While this is a significant milestone, remember that the journey of discovery and learning in programming never ends.
We at Zenva, always strive to provide the highest quality learning materials to support you in your quest for knowledge. To continue learning and practicing, we encourage you to check out our Roblox Game Development Mini-Degree. This comprehensive set of courses is designed to impart the skills necessary for game creation with Roblox Studio and Lua, without any prerequisite of prior coding experience. By the end of these courses, you will arm yourself with hands-on experience and an enriching professional portfolio in game development.
We also offer a broader collection of courses on Roblox game development suitable for various learning preferences and experience levels. Embrace this opportunity to further enhance your Lua scripting abilities and rise towards an empowering future in programming and game development with Zenva.
Conclusion
With its rich features, simplicity, and wide usage in various sectors, Lua scripting opens doors to many lucrative career paths, including game development, web server building, and embedded systems. Thank you for joining us on this guided journey into the fascinating world of Lua scripting.
Ready to level up your Lua skills? Join us at Zenva Academy! Our comprehensive Roblox Game Development Mini-Degree offers a deep dive into Roblox Studio, Lua scripting, and more, setting you on the course to become an empowered game developer. Start building awesome games with us today!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
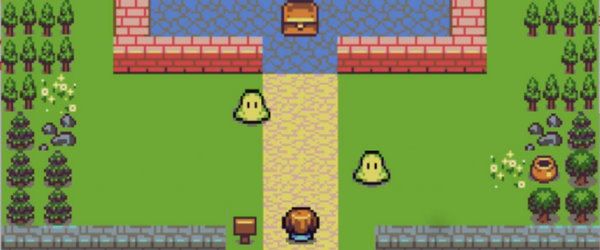
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.