Welcome to this engaging tutorial on Roblox variables! Unraveling the world of coding can feel like learning a new language, but worry not, because this guide promises to provide you a stepping stone into this exciting domain. Our focus here will mainly be on a key component of coding in Roblox, which is variables.
Table of contents
What Are Roblox Variables?
Variables in Roblox, like in any other programming language, are fundamental. They are nothing more than storage for your data. You can think of them as a container holding a value which you can use throughout your game’s script.
What is their role?
Roblox variables play a pivotal role in game development. They allow for the storage and manipulation of data, such as player scores, game settings or character states, which are crucial in creating dynamic and interactive gameplay.
Why Learn About Roblox Variables?
Mastering the use of variables is a basic yet critical step into coding in Roblox. It adds a layer of complexity and interactivity to your games. Plus, gaining a robust understanding of variables will make it easier to pick up other coding concepts later on.
Declaring a Variable in Roblox
The first step to using a variable is to declare it. This means creating it and giving it a name.
You can declare a variable in Roblox using the ‘local’ keyword followed by the name of the variable. Here is the basic syntax:
local variableName
For example, if you want to create a variable to store a player’s score, you can name it “playerScore”.
local playerScore
At this point, the variable “playerScore” has been created, but it’s empty. It doesn’t contain any value.
Assigning a Value to a Variable
The next step is to assign a value to our variable. We can directly assign a value while declaring the variable, or assign it later in the code.
To assign a value to a variable use the ‘=’ symbol, followed by the value. Let’s put a value into our “playerScore” variable:
playerScore = 10
Now, “playerScore” holds the value 10. We can also declare and assign a value to a variable at the same time:
local playerScore = 20
The variable “playerScore” is declared and given a value of 20 simultaneously.
Variable Types in Roblox
Roblox supports several types of data which variables can hold. These include:
- Numeric Values: These are numbers. Example:
local playerScore = 100
- String Values: These are sequences of characters. Example:
local playerName = "RobloxGamer"
- Boolean Values: These are either true or false. Example:
local isGameOn = true
Changing a Variable’s Value
You can update or change a variable’s value by simply assigning a new value to it. For instance, let’s assume that our player has earned more points, and we want to update “playerScore”:
playerScore = 150
Now our “playerScore” variable holds the new value 150. Always remember that a variable’s value will be the last value assigned to it.
Using Roblox Variables
Now that we’ve learned how to create and assign values to variables, let’s discuss how to use them in our code.
Performing Operations
You can perform various operations with variables. For example, if we have two variables representing player scores, we can add them together:
local playerScore1 = 100 local playerScore2 = 50 local totalScore = playerScore1 + playerScore2
The “totalScore” variable will now hold the value 150, which is the sum of “playerScore1” and “playerScore2”.
Printing Variables
Another usual practice is printing the value of a variable to the output. This is especially useful for debugging your scripts. You can use the “print” function in Roblox for this:
print(totalScore)
This will print the value of “totalScore” in the output.
Reusing Variables
The power of variables lies in their reusability. Once a variable is declared, it can be used elsewhere in the script. This reduces redundancy and keeps our code clean:
local playerName = "RobloxGamer" local message = "Welcome, " .. playerName print(message)
Here, we first declare a variable “playerName”. Then we use it to construct a welcome message which we print out. This will output “Welcome, RobloxGamer”.
Using Variables in Functions
Variables can also be used within functions. This allows us to make our functions more dynamic and adaptable to changing game conditions:
function updateScore(score) local newScore = totalScore + score totalScore = newScore print("Score updated to: "..totalScore) end updateScore(50) updateScore(100)
This function, updateScore, takes a score as parameter, adds it to the “totalScore”, and then updates the “totalScore”. By calling the function twice, we add 50 and then 100 to the total score, and print the updated score each time.
Through this exercise, we hope you’ve not only gained a clearer understanding of how Roblox variables function, but also experienced the practical applications of them in a game scripting context.
Conditions with Variables
Variables are often used in condition checking. For example, you can use if-else statements to perform different actions depending on a variable’s value.
local player_lives = 3 if player_lives > 0 then print("Player still has lives left.") print("Lives left: "..player_lives) else print("Game Over.") end
In this example, we check if the player still has lives left. If “player_lives” is greater than 0, we print out the number of lives left. If not, we display a “Game Over” message.
Loops with Variables
Variables are also frequently used in loops. For instance, you can loop through a certain number of times depending on a variable’s value.
local num_of_enemies = 5 for i = 1, num_of_enemies do print("Spawned enemy number: "..i) end
In this code snippet, “num_of_enemies” is the variable that determines how many times the loop should run. Each time the loop runs, an enemy is spawned and a message is printed. This is an efficient way of controlling the number of enemies spawned in our game.
Table Variables
Another essential type of variable in Roblox is a table variable, which can hold multiple related values and offer options for more complicated data handling.
local player = {} player["name"] = "RobloxGamer" player["score"] = 100 print(player["name"]) print(player["score"])
Here, the “player” variable is a table that holds both the player’s name and score, which can then be accessed individually with the table’s respective keys (“name”, “score”).
Roblox Instance Variables
To truly grasp the power of variables in Roblox, you must also understand instance variables. Instance variables can be used to define a particular attribute or property of a Roblox object or instance.
local part = Instance.new("Part") part.Parent = game.Workspace part.Name = "MyPart" print(part.Name)
In the above example, we create a new Part instance, assign it to the workspace, and name it “MyPart”. We can later access the part’s name via the “Name” property, which is an example of an instance variable.
Understanding and implementing variables in Roblox scripting offers seemingly endless possibilities for game customization, data recording, and player interaction, underscoring their fundamental importance in coding endeavors. The more comfortable you become with Roblox variables, the more sophisticated and engaging your game creations can become.
Embarking on your coding journey doesn’t have to be daunting. Fret not, because we, at Zenva, are committed to providing ample support throughout your journey, from beginner to professional. Warmed up with your initial adventure into Roblox variables? We assure you, this is just an exciting first look into the enormous universe of game creation.
For those enthusiastic about venturing deeper into game development, we highly recommend our Roblox Game Development Mini-Degree, a comprehensive collection of courses where you’ll learn to create different game genres using Roblox Studio and Lua. It’s perfect for beginners without prior experience, designed with flexibility allowing learning conveniently on any modern device, and upon completion, you’d have developed a professional portfolio and relevant skills for a thriving career in game development.
Moving forward, you might also wish to explore our exhaustive Roblox collection for more deep dives into specific topics. Remember, the more you practice, the better you become. So, let’s start coding!
Conclusion
And that’s a wrap on variables in Roblox! We hope you have found this guide helpful in acquiring a fundamental understanding of a key concept in the coding world. The realm of game development presents endless opportunities for creativity and engagement. Though this is but a stepping stone in mastering game creation, it is a crucial one. Games can become more nuanced, complex, and engaging as you delve deeper into coding, and variables are the basic building blocks.
Ready to take the next step in your game development journey? Be sure to check out our comprehensive Roblox Game Development Mini-Degree. As you delve deeper into the world of coding, remember – knowledge is power and the potential for creativity is limitless! Let’s embark on this incredible journey together. Happy coding!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
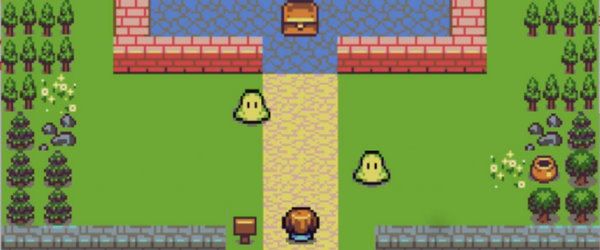
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.