Welcome to this informative and educational tutorial on the “Lua Environment”. Lua is a powerful, efficient, lightweight, and embeddable scripting language that is utilized in a variety of applications ranging from web development to game design. If you are interested in expanding your coding knowledge and abilities, particularly in the realm of game creation, this fundamental understanding of Lua and its environment is exactly what you need.
Table of contents
What is Lua?
Lua is a high-level, interpreted scripting language that is dynamically typed, runs by interpreting bytecode with a register-based virtual machine, and has automatic memory management. Originally developed in Brazil, Lua has gained global popularity due to its simplicity, efficiency and ease of embedding.
Why should I learn Lua?
So why should you learn Lua? Here is why:
- Wide Applications: Lua is used in many industrial applications, with an emphasis on embedded systems and games.
- Robust, Expressive, and Easy to Learn: Lua’s straightforward syntax and semantics make it an excellent first language for beginners.
- Efficient Execution: Lua scripts run efficiently on its lean runtime, making it optimal for environments with limited resources.
Learning Lua programming can open up doors for you to explore game development in a faster and easier way. It is used in commercial video games, embedded systems, image processing and more. Dive into this tutorial to begin your exciting journey into Lua coding.
Setting Up Lua Environment
Before diving into the code, you’ll need to install and set up Lua in your environment. There are multiple ways to install Lua, but likely the easiest is through Homebrew.
brew install lua
While the above command will install Lua on your machine, we strongly recommend using a standalone Lua version manager, such as Luaver. This allows you to install multiple Lua versions and switch them easily:
# Install Luaver wget https://raw.githubusercontent.com/DhavalKapil/luaver/master/install.sh -O - | bash # Reload your shell source ~/.bashrc # Install a version of Lua luaver install 5.3.4 # Use that version luaver use 5.3.4
Writing Your First Lua Program
Now that the environment is set up, let’s write your first Lua program. In Lua, ‘print’ function is used to display output. Save the following code in a file named ‘helloWorld.lua’:
-- This is a comment in Lua print("Hello, World!")
You can then run the program with lua helloWorld.lua command line syntax.
Variables and Types in Lua
Lua is a dynamically typed language, which means that variables don’t have types; only values do. There are eight basic types in Lua. These are, namely,: nil, boolean, number, string, userdata, function, thread, and table. Let’s look into a few examples.
-- Assigns the integer 10 to variable x local x = 10 -- Assigns the string "hello" to variable y local y = "hello" -- Prints their types print(type(x)) -- Output: number print(type(y)) -- Output: string
Control Structures in Lua
Control structures are a fundamental aspect to any programming language. In Lua, you have if-then-else and while loops amongst other constructs:
--if-then-else structure x = 12 if x > 10 then print('x is bigger than 10') -- Output: x is bigger than 10 else print('x is smaller or equal to 10') end
--while loop structure x = 5 while x > 0 do print(x) -- Output: 5 4 3 2 1 x = x - 1 end
With these basics covered, you’re ready to continue exploring Lua and start applying it to your next game project. Remember that practice is key in mastering a new language so be sure to experiment with what you’ve learned here in different ways. Happy coding!
Functions in Lua
In Lua, there are two types of functions, the Global Functions and the Standard Libraries Functions. Here’s how to declare a simple function:
-- Declare the function function sayHello(name) return "Hello, " .. name end -- Call the function print(sayHello("World")) -- Output: Hello, World
Functions can also return multiple values:
function calc(a, b) return a + b, a - b, a * b, a / b end sum, difference, product, quotient = calc(10, 5) print(sum) -- Output: 15 print(difference) -- Output: 5 print(product) -- Output: 50 print(quotient) -- Output: 2
Tables in Lua
Tables are the only data structuring mechanism in Lua. They are used to represent arrays, symbols, sets, lists, and records. Here’s an example of how to use a table like an array:
-- Define the array local array = {"Apple", "Pineapple", "Grape"} -- Fetch elements print(array[1]) -- Output: Apple print(array[2]) -- Output: Pineapple print(array[3]) -- Output: Grape
And here’s an example of a table as a dictionary:
-- Define the dictionary local dict = {name = "John", age = 25, country = "USA"} -- Fetch elements print(dict["name"]) -- Output: John print(dict["age"]) -- Output: 25 print(dict["country"]) -- Output: USA
Error Handling in Lua
Lua provides an error function, which terminates the running program and prints an error message. Here’s how it’s used:
-- Define the function function divide(a, b) if b == 0 then error("You tried to divide by zero!") end return a / b end -- Call the function print(divide(10, 0)) -- Output: Error: You tried to divide by zero!
Lua also provides the pcall (protected call) function, which runs a function and catches any errors that may happen during the execution:
-- Define the function function divide(a, b) if b == 0 then error("You tried to divide by zero!") end return a / b end -- Call the function and catch the error status, result = pcall(divide, 10, 0) -- Print the status and the result if status then print(result) else print(result) -- Output: You tried to divide by zero! end
Understanding functions, tables and error handling in Lua will give you a strong foundation in utilizing this powerful language for your coding projects. Practice these concepts until you’re comfortable with them and you’ll be well on your way to becoming proficient in Lua!
Lua Modules and Packages
Lua modules are similar to JavaScript modules. They divide a program into multiple parts to avoid the pollution of the global namespace. Here’s how you can create and use a module:
-- We're creating a module named mod. Save this in a file named mod.lua local M = {} -- This is the standard way to create a module function M.greet(name) return "Hello, " .. name end return M
-- Now let's use the module we just created. -- Import the module local mod = require "mod" -- Use the module print(mod.greet("World")) -- Output: Hello, World
Packages in Lua are modules that are organized in a directory hierarchy. Here’s how you can use packages:
-- Import a package local socket = require "socket" -- Use the package print(socket.gettime())
Metatables in Lua
Metatables in Lua are tables that can change the behavior of the original table. They have different metamethods, the most commonly used ones are __index, __newindex, and __call:
-- Create the metatable local mt = { __index = function (table, key) return "This key does not exist!" end } -- Create the table local t = {} -- Set the metatable for the table setmetatable(t, mt) -- Access a non-existent key print(t["non_existent_key"]) -- Output: This key does not exist!
-- Create the metatable local mt = { __call = function (table, str1, str2) return str1 .. " and " .. str2 end } -- Create the table local t = {} -- Set the metatable for the table setmetatable(t, mt) -- Call the table as if it's a function print(t("Pineapple", "Apple")) -- Output: Pineapple and Apple
Understanding how to utilize modules, packages, and metatables will allow you to write cleaner, more efficient Lua code. Master these and you will be well on your way to creating professional-quality video games and applications. Keep practicing these particular concepts – don’t worry if you don’t get it straight away, with enough practice they will become second nature! Happy coding!
Where to go next?
Now that you have laid the foundation and gained a basic understanding of Lua, it’s the perfect time to apply and expand on what you’ve learned. We, at Zenva, are keen on empowering you with the skills to elevate your coding and game development journey to the next level.
Why not consider giving our Roblox Game Development Mini-Degree a shot? This comprehensive collection of courses covers game creation with Roblox Studio and Lua to further deepen your understanding. With a focus on Roblox basics, scripting, as well as creating melee battle and first-person shooter games, this curriculum is suitable for beginners seeking to gain practical, hands-on experience. Fitting learning into your busy day won’t be a hassle as all courses are meticulously designed to be flexible, allowing 24/7 access to interactive lessons and coding practice.
If you’re specifically interested in exploring more Roblox opportunities, you may want to delve into our wide range of Roblox courses.
Remember, learning is a lifelong journey. With constant practice and persistence, you’ll be amazed at how far you can go. We wish you all the best on your coding adventure!
Conclusion
Learning to code is an exciting journey, and mastering Lua is a significant step in that journey. Not only does it open up avenues in game development, but it also empowers you to develop a wide range of applications. By undertaking this tutorial, you’ve taken a crucial step towards conquering this versatile language, and we’re excited about the many doors of opportunity that will open for you.
Join us at Zenva for a deeper dive into Lua, its interesting applications, and much more. Check out our Roblox Game Development Mini-Degree. Go ahead, unleash the coder in you. We’re thrilled to have you on board, and we can’t wait to see where your coding journey takes you next!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
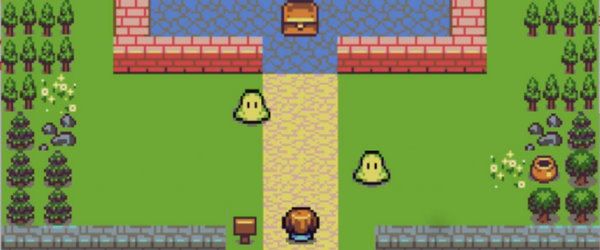
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.