Welcome aspiring coders and game developers to this exploratory journey into the world of Lua closures! This self-contained tutorial is your golden opportunity to dive deep into the fascinating and powerful world of Lua and learn about one of its most important but often misunderstood concepts: Closures.
Table of contents
Demystifying Closures
So, what exactly are closures? Within the realm of Lua, closures represent a fundamentally important concept that can immensely enhance the power of your scripts.
In simple terms, a closure is a function object that has access to variables from its parent scope, even after the parent function has terminated. In essence, closures enable you to add an extra layer of power and flexibility to your Lua code by enabling functions to carry some data around with them.
The Power of Closures
Why should you take the time to learn and master closures? Well, in addition to being one of the fundamental building blocks of Lua, closures play a vital role in game development, particularly when it comes to scripting interactive experiences and developing complex game mechanics.
Their power lies in their ability to foster cleaner, more organized code by giving functions the ability to remember and manipulate data over time. So, if you’re looking to become a confident Lua programmer and bring your wildest game ideas to life, understanding closures should definitely be at the top of your learning checklist.
Creating a Simple Lua Closure
Let’s start with the basics – creating a simple closure in Lua. Keep in mind, a closure is essentially a function that can access its parent scope variables, even after the parent function has finished its execution.
function outerFunction() local x = 5 local innerFunction = function () print(x) end return innerFunction end local closure = outerFunction() closure()
Here, the outerFunction()
holds a local variable x
and defines an `innerFunction()`. That innerFunction()
is also referred to as a closure because it can access the x
variable from its parent function. Note that even after outerFunction()
has finished its execution, innerFunction()
still has access to x
.
Manipulating Variables Within a Closure
As you get more comfortable with closures, it’s time to explore how they can be used to manipulate variables within a function. As you’ll see in the following example, a closure can not only access but also change the value of variables from its parent function.
function outerFunction() local x = 5 local innerFunction = function () x = x + 1 print(x) end return innerFunction end local closure = outerFunction() closure() -- This will print 6 closure() -- This will print 7
Here, not only does the closure innerFunction()
retain access to the parent function’s local variable x
, but it also changes its value with each call. This is a key concept of closures and demonstrates their ability to carry data over time.
Creating Multiple Closures within a Function
Next up, let’s get into creating multiple closures within a single function. This can be quite useful when used wisely, and it further emphasizes the power of closures in manipulating and persistent storage of data.
function outerFunction() local x = 0 return function() x = x + 1 return x end, function() x = x - 1 return x end end local increment, decrement = outerFunction() print(increment()) -- This will print 1 print(decrement()) -- This will print 0 print(increment()) -- This will print 1 print(increment()) -- This will print 2
Here, the outerFunction()
returns two closures. The first increases the value of x
and returns it, whereas the second decreases the value. Both these closures share the same local variable x
from their parent function, proving that multiple closures can exist within the same function and work on the same variable.
Taking Advantage of Closures
As we move forward, it’s time to look into more complex use-cases and truly harness the power of closures.
Creating Configurable Functions with Closures
One practical use of closures is to create configurable functions. Configurable functions are essentially functions that can be customized for performing specific operations. For instance, suppose you’re working on a game and you need to create different types of increase or decrease functions for various player statistics (like health, score, etc.). Here’s how you can do that using closures:
function createStepFunction(step) local x = 0 return function () x = x + step return x end end local increaseByTwo = createStepFunction(2) local decreaseByThree = createStepFunction(-3) print(increaseByTwo()) -- This will print 2 print(increaseByTwo()) -- This will print 4 print(decreaseByThree()) -- This will print -3 print(decreaseByThree()) -- This will print -6
In this example, createStepFunction()
returns a function that increases its local variable x
by the specified step. This allows us to create configurable increase and decrease functions that can be used throughout the game code.
Creating Private Variables with Closures
Closures can also be used to create private variables in Lua – a key concept for organizing code and preventing unwanted modifications. Since there’s no “private” keyword in Lua, you can emulate private variables using closures.
function createCounter() local count = 0 return function () count = count + 1 return count end end local counter = createCounter() print(counter()) -- This will print 1 print(counter()) -- This will print 2
In the above example, the closure can modify count
, but any code outside of createCounter()
has no access to it. Effectively, count
acts as a private variable.
Preserving State Across Calls with Closures
Closures have the unique ability to maintain states across different function calls. This property can be very useful to create iterators or generate a series of numbers. Let’s check it out:
function fibonacci() local a, b = 0, 1 return function() a, b = b, a + b return a end end local fib = fibonacci() for i = 1, 10 do print(fib()) end
This will print the first 10 numbers of the Fibonacci series. The fibonacci()
function generates a closure that preserves the state of the variables a
and b
across calls, allowing us to generate the Fibonacci series.
By now, we hope you have a deeper understanding of the power and flexibility that closures bring to the table. They certainly have a learning curve, but once mastered, they can significantly enhance your Lua coding skills and open up new possibilities for your game developments. So keep learning and practicing!
Using Closures for Asynchronous Programming
Another advanced use case of closures in Lua is to introduce asynchronous programming. While Lua natively supports coroutine-based multitasking, closures can be used to create continuations, a type of structure widely used in callback-based coding styles.
function asyncRead(file, callback) -- In a real system, this would be an asynchronous call local content = io.open(file):read("*a") callback(content) end function processFile(file) local closure = function (content) print("File " .. file .. " content: " .. content) end asyncRead(file, closure) end processFile('test.lua')
This example demonstrates a simplistic asynchronous file read. The closure in `asyncRead` function will not be called until the file is fully read.
Implementing Object-Oriented Techniques using Closures
While Lua supports object orientation through metatables, closures allow flexibility to create objects with behaviors in different styles. Let’s see an example:
function createStack() local stack = {} return { push = function (x) table.insert(stack, x) end, pop = function () local x = stack[#stack] stack[#stack] = nil return x end, isEmpty = function () return #stack == 0 end } end local s = createStack() s.push(1) s.push(2) s.push(3) while not s.isEmpty() do print(s.pop()) end
In this example, we’ve created a stack object with three closures as methods that manipulate the internal state of the object. All the closures share the same stack
object and can manipulate it independently.
Using Closures in Higher Order Functions
Closures are a foundational principle in functional programming, as they allow higher-order functions to encapsulate behavior dynamically, boosting the modularity and reusability of your code.
function map(func, list) local result = {} for i, v in ipairs(list) do result[i] = func(v) end return result end function square(x) return x * x end local list = {1, 2, 3, 4} local squares = map(square, list) for i, v in ipairs(squares) do print(v) end
This example defines a `map` function that applies a given function (`func`) to each element of a list. We then use this higher-order function with a simple `square` closure.
As you delve deeper into this type of programming, you’ll find that closures are a glue that holds higher-order functions together while offering excellent code reuse, abstraction, and encapsulation possibilities.
Conclusion
In this detailed exploration, we’ve seen closures in many shapes and use cases, from aids in the organization and encapsulation of our code, to powerful tools for asynchronous and functional programming styles.
Grasping these concepts solidly not only makes coding in Lua more enjoyable, but it is a pivotal instrument for game development, making it a must-have skill in any aspiring game developer’s toolbox.
At Zenva, we believe in the power of learning by doing. We hope this piece has helped demystify closures, and you’re now one step closer to becoming a proficient Lua programmer. Keep practicing!
Where to Go Next
Mastering Lua closures is just the beginning of your journey into the immense and exciting world of programming and game creation. We encourage you to keep exploring, trying new things and building on what you’ve learned so far.
The logical next step could be to put your Lua skills to practical use. Why not consider game development with Roblox? Our Roblox Game Development Mini-Degree is a comprehensive sequence of courses that covers basic level creation, Lua scripting, multiplayer combat, and more. Suitable for beginners with no prior coding experience, you’ll have the flexibility to select the learning materials that best suit your style and pace.
As you progress, feel free to explore our broader Roblox course collection. From beginner to professional, Zenva offers over 250 supported courses to boost your career. Who knows, your name could be the next in the list of those who’ve published their own games, landed job opportunities, or started businesses with skills learned from our courses! Keep going and happy learning.
Conclusion
Congratulations on diving into the intricacies of Lua closures and enhancing your scripting prowess. There’s endless power and potential in your hands now, ready to be harnessed in creating mind-blowing games and intricate coding projects.
Remember, mastery comes with practice, and at Zenva, we’re here to assist you at every step of the way. Keep broadening your horizons with our Roblox Game Development Mini-Degree and explore the exciting universe of game creation. Your journey has only just begun!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
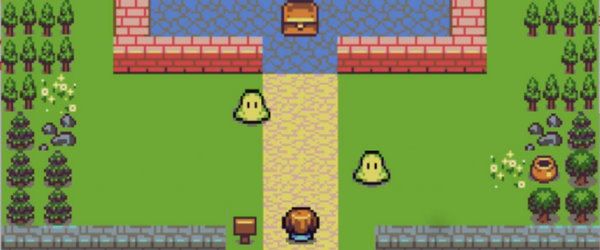
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.