Welcome everyone! In today’s coding tutorial, we’ll be delving into the enthralling world of Lua and the critical concept of error handling. Learning how to handle errors proficiently is a fundamental aspect of your journey into becoming an efficient and reliable Lua programmer, primarily if your focus is on creating engaging game mechanics.
Table of contents
What is Lua Error Handling?
Lua Error Handling is a set of techniques used by Lua programmers to identify, debug, and fix potential errors or exceptions in their code. Like in real-world scenarios where errors or unexpected events happen, Lua also expects and plans for the moment when things don’t go as planned, and that’s where error handling comes into play.
Why Should I Learn Lua Error Handling?
Lua Error Handling is essential for several reasons:
- It helps programmers understand what’s gone wrong in their code.
- It provides an orderly method of recovering from an error.
- It improves overall code reliability and robustness.
- Crucially, it fosters good coding practices by encouraging troubleshooting and debugging as part of regular coding routines.
So, if you are intent on developing games using Lua, mastering error handling is a must. Not only will this knowledge fine-tune your coding skills, it’ll also help in creating more resilient games.
What’s in it for me?
By learning Lua Error Handling, you won’t be left scratching your head when something unexpected happens in your code. Instead, you’ll have the tools necessary to identify and correct any issues swiftly and efficiently – a key trait of successful programmers. So, let’s jump right in, and soon you’ll be navigating through your set of codes with growing confidence and determination.
Getting Started with Lua Error Handling
Conceptually, error handling in Lua can be broken down into three key functions: error, pcall, and xpcall. Let’s dive into understanding these essential functions and demonstrate them through illustrative examples.
The error() Function
The error function is quite simple: it stops the execution of the current function and reports an error.
function divide(a, b) if b == 0 then error("Divide by zero error!") else return a / b end end print(divide(4, 0))
In the above code, attempting to divide by zero triggers our error, and Lua will helpfully output our error message: “Divide by zero error!” This halts the program at the point of error, allowing us to identify and rectify our mistake.
The pcall() Function
pcall (which stands for protected call) is a function that executes another function in protected mode. This means that it catches any errors that occur during execution and prevents them from crashing the program.
function faultyFunction() error("This function always errors!") end local status, err = pcall(faultyFunction) if not status then print("Caught an error: " .. err) end
In this example, pcall executes the faultyFunction, which promptly errors. However, instead of causing everything to collapse, pcall returns two values: a status (false if there was an error) and the error message (if there was an error).
The xpcall() Function
xpcall works similarly to pcall, but it has an additional feature: it accepts an error function that it calls if an error occurs. This can be handy for tracing errors, as shown in the example below:
function traceError(err) print("Error: " .. err) end function faultyFunction() error("This function always errors!") end status = xpcall(faultyFunction, traceError) if not status then print("An error occurred.") end
The traceError function is called when faultyFunction errors, allowing us to trace and print the error message accordingly.
These functions should form the foundation of your Lua error handling toolkit, and can be incredibly valuable in developing clean, error-free programs. We encourage you to play around with the code samples provided to familiarise yourself with these concepts.
Digging Deeper into Lua Error Handling
Now that we have built a solid foundational understanding of Lua’s basic error handling constructs, let’s delve into some more practical examples and scenarios where these techniques come prominently into play.
1. Safely Reading a File
One common place where you’d want to handle errors is file I/O operations. Consider a scenario where you’re attempting to read a file that doesn’t exist. In normal situations, this would crash your program. But with proper handling, the error can be caught and handled neatly:
function openFile(filename) local file, err = io.open(filename, "r") if not file then print("Cannot open file: " .. err) else -- perform file operations file:close() end end pcall(openFile, "non_existent_file.txt")
2. Error Handling in User-Defined Functions
Consider a function where we take a number as an argument and check if it is an even number:
function checkIfEven(n) if not n or type(n) ~= 'number' then error("Input is not a valid number!") elseif n % 2 ~= 0 then error("Input is not an even number!") else print("Given number is even.") end end local status, err = pcall(checkIfEven, "abc") if not status then print("Caught an error: " .. err) end
In this code, we’re handling two potential types of errors – invalid type and odd number, and print an appropriate error message for each.
3. Function Returning Error
You can also have a function return an error as part of its standard operation:
function safeDivide(a, b) if b == 0 then return false, "Divide by zero error!" else return true, a / b end end local status, result = safeDivide(4, 0) if not status then print("Caught an error: " .. result) end
In this sample, we’re again dividing numbers, but this time, instead of halting execution, we return boolean status alongside our result/error message.
4. Using xpcall() to Perform Cleanup Operations
Consider a situation where we’re working with resources that need to be released, like open files or network connections. Even when an error occurs, we want to ensure that these resources are cleaned up:
function cleanup(err) print("Error occurred: " .. err) -- Perform cleanup operations here like closing files, connection etc. print("Cleanup Done") end function errorProneFunc() error("Some random error") end xpcall(errorProneFunc, cleanup)
In this situation, the cleanup() function takes care of releasing the resources when an error happens, ensuring our program doesn’t leak resources.
These practical scenarios and code snippets should give you a good understanding of how error handling works in Lua and how important it is for writing robust, reliable code. Remember to experiment and iterate on these examples, as the best way to learn is through experience and practice!
Further Exploring Lua Error Handling
Now that we’ve had a look at a few practical examples, we’ll take a closer look at some of the more subtle parts of Lua’s error handling. We will walk through some additional situations where you might need to handle errors and how you might go about doing so.
5. Checking for nil values
One common place where errors can occur is when dealing with potentially nil values. Consider the following code:
function greetUser(username) if not username then error("Username is missing.") else return "Hello, " .. username end end status, msg = pcall(greetUser, nil) if not status then print("Error: " .. msg) else print(msg) end
Here, we’ve anticipated the case where the username might be nil and handled the error gracefully by detecting it.
6. Chain of Function Calls
In Lua, an error originating from one function can be caught by another function higher up the call chain. This can be illustrated by a sequence of function calls, where the functions allow their errors to bubble up to be handled by the initial calling function:
function thirdFunction() local r = io.open("non_existent_file.txt") end function secondFunction() thirdFunction() end function firstFunction() secondFunction() end status, err = pcall(firstFunction) if not status then print("Caught an error: " .. err) end
This type of error handling set-up can be extremely useful when you are modeling complex functions with a hierarchical structure.
7. Using Assertions
Lastly, in Lua, we can find a handy function called assert. This function tests if a certain condition is true, and if it’s not, it will throw an error message. This method is very effective in debugging:
function checkPositive(n) assert(n > 0, "Number is not positive!") print("Given number is positive.") end status, err = pcall(checkPositive, -5) if not status then print("Error: " .. err) end
Here, when checkPositive receives a non-positive number, the assert function triggers an error which gets caught in the pcall.
8. Using error(): Advanced
Finally, let’s delve a bit more into the working of the error function. It accepts two arguments, the error message, and a number which indicates the level in the call stack where the error happened:
function levelOne() levelTwo() end function levelTwo() error("Error at Level Two!", 2) end pcall(levelOne)
Here, when the error is thrown and is not caught in levelTwo(), it bubbles up to levelOne().
By practicing and mastering these different techniques for catching and handling errors in Lua, you can ensure that even when your code encounters unexpected inputs or conditions, it doesn’t just crash. It evaluates, reports and even recovers, leading to more robust and reliable code development. Let this be a part of your regular coding routine as it fosters good coding practices.
Next Steps in Your Coding Journey
Now that you’re equipped with the essentials of error handling in Lua, what’s your next step? We encourage you to continue with your fascinating journey into coding and game creation.
At Zenva, we have assembled a diverse range of comprehensive courses to support and extend your learning. Our curriculum is designed with the sole purpose of sharpening your skills and making you industry-ready, no matter where you are in your learning journey.
A fantastic next step could be to explore our Roblox Game Development Mini-Degree. This comprehensive collection of courses is focused on game creation using Roblox Studio and Lua. It covers various game genres and teaches critical skills such as adding multiplayer functionality and leaderboards.
If you want to delve into more specific areas within Roblox development, be sure to check out our broader collection of Roblox courses here.
Embrace the challenge, learn at your own pace, and create compelling games to showcase your hard-earned skills. With Zenva, every step brings you closer to becoming a seasoned professional. Happy coding!
Conclusion
Becoming fluent in Lua error handling is like gaining a superpower – the power to ensure your game or application behaves safely and predictably, even when the unexpected occurs. We’ve walked you through the fundamentals and provided practical application examples, and we truly believe that with practice and perseverance, you can master these techniques.
At Zenva, we’re committed to providing you with the resources and guidance you need to successfully navigate your coding journey. Learning doesn’t stop here. Get ahead with our Roblox Game Development Mini-Degree and dive deeper into the exciting world of game creation. Remember – “Every line of code is an achievement.” Here’s to you always achieving more!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
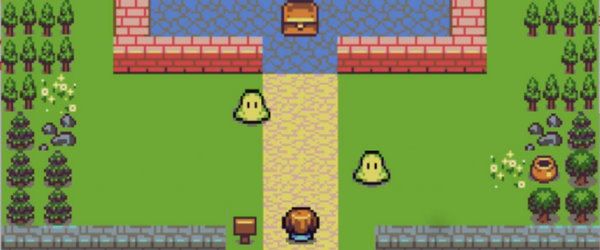
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.