Welcome to our comprehensive tutorial on Lua syntax! We aim to unlock the mysteries of Lua – a lightweight and efficient scripting language widely employed in game development. If you’ve been curious about Lua or are looking to enhance your game creation skills, you’re at the right place!
Table of contents
What is Lua?
Lua is a powerful, efficient, and lightweight scripting language. It is embeddable and has a simple procedural syntax, making it a favorite among game developers, particularly in areas of AI scripting and gameplay mechanics.
What is it for?
One of Lua’s primary advantages is its applicability in video game development. Games like World of Warcraft and Angry Birds have used Lua for its ability to handle intricate programming tasks using a simple syntax style.
Why should I learn Lua?
Learning Lua opens doors to creating and modifying game scripts, offering you the power to customize gameplay features and even build games from scratch. Plus, its streamlined syntax ensures that you spend more time creating and less time debugging!
Basic Lua Syntax
As we delve into the basics, you’ll soon realize Lua is as easy to learn as it is powerful. Let’s start by examining how to declare variables in Lua.
-- Number Variable local a = 5 -- String Variable local b = "Hello, Zenva" -- Boolean Variable local c = true
In the above code, we’ve declared three variables: a number, a string, and a boolean. In Lua, variables are declared using the local keyword. Also, Lua uses — for single-line comments.
Data Types in Lua
Lua has eight basic data types, all of which are easy to comprehend and use. Here are some examples:
-- Number local num = 10 -- String local str = "Learn at Zenva" -- Nil local n = nil -- Boolean local flag = true
In Lua, nil is a type that has one value: nil. It is typically used to represent the absence of a useful value.
Control Flow in Lua
Now, let’s check out Lua’s control flow syntax with examples of “if” and “for” statements.
-- If statement local num = 10 if num > 5 then print("The number is greater than 5") end -- For loop for i = 1, 10, 1 do print(i) end
The “if” statement is used to make decisions based upon different conditions. The “for” loop, on the other hand, executes a block of code for a certain number of times.
Functions in Lua
Functions in Lua are first class values, which means they can be stored in variables, passed as function parameters, and returned as function results. Here’s how to define and use a function in Lua:
-- Defining a function function add(a, b) return a + b end -- Using a function local sum = add(5, 10) print(sum) -- prints 15
In the above example, we define a function named add that takes two parameters, adds them, and returns the sum. We then call this function with the numbers 5 and 10 as arguments and print the result.
Lua Tables
Lua uses tables to help represent a wide variety of data structures. From standard arrays and dictionaries to more complex ones like graphs and trees, tables cater for them all.
Let’s start with a simple example of how to declare a table in Lua:
-- Defining a table local fruits = {"Apple", "Banana", "Cherry"}
Accessing table elements in Lua is just as straightforward:
-- Accessing an element print(fruits[1]) -- prints "Apple"
To add elements to our table, we can use the following method:
-- Adding an element table.insert(fruits, "Mango") print(fruits[4]) -- prints "Mango"
We can also use tables as maps/dictionaries. Here’s how:
-- Using a table as a dictionary local fruits = {Apple = 1, Banana = 2, Cherry = 3} print(fruits["Apple"]) -- prints 1
File I/O in Lua
In Lua, file input/output operations are very straightforward. Let’s see how to write content to a file:
-- Opening a file in write mode local file = io.open("test.txt", "w") -- Writing to the file file:write("Hello, Zenva!") -- Closing the file file:close()
Alternatively, we can read content from a file like so:
-- Opening a file in read mode local file = io.open("test.txt", "r") -- Reading the file content local content = file:read("*all") -- Printing the content print(content) -- prints "Hello, Zenva!" -- Closing the file file:close()
Great! Now that we’ve covered the basics, it’s time to use Lua to its fullest potential and build exciting game scripts and more at Zenva.
Lua Error Handling
Error handling is critical in programming to prevent common coding issues from causing serious problems in game scripts. In Lua, error handling is undertaken using the pcall command and the error function. Check it out:
-- Defining a function that can cause an error local function faulty_function() error("An error occurred") end -- Using pcall to catch the error local status, err = pcall(faulty_function) if not status then print(err) -- prints "An error occurred" end
In the above example, pcall (protected call) runs the function within a protected mode. This means it captures the error and continues executing the program instead of stopping it abruptly.
Working with Modules in Lua
Modules in Lua are like libraries: you can define functions in a module, then call them in other parts of your program. Here’s a simple example:
-- Defining a module local M = {} function M.add(a, b) return a + b end return M
In this example, we created a basic module M with a function named add. To utilize the module in different sections of your program, use the require function:
-- Using a module local mod = require("module") local sum = mod.add(5, 10) print(sum) -- prints 15
Object-Oriented Programming in Lua
Lua includes basic support for object-oriented programming (OOP). Though it lacks built-in classes, we can utilize tables and metatables to simulate them and create a powerful OOP infrastructure:
-- Defining a "class" local class = {value = 0} class.__index = class -- Defining a "method" function class:incr() self.value = self.value + 1 end -- Creating an "object" local obj = setmetatable({}, class) -- Using the method obj:incr() -- Prints the "object"'s value print(obj.value) -- prints 1
In the above, we’re following the typical object-oriented routine: defining a class, adding a method, creating an object of the class, and invoking the method on this object. It’s simple, efficient and fun!
By now, you should have a good grasp of Lua’s versatile programming landscape and its wide application in game development. So, power up your scripting engine and make the most of Lua at Zenva!
So you’ve grasped the basics of Lua and want to power on with your coding journey? Fantastic! Here at Zenva, we offer a multitude of beginner to professional courses in programming, game development, and AI. Whether you’re a newbie coder or an experienced developer aiming to boost your career, you’ll find over 250 supported courses designed to take you from beginner to professional.
For those of you who’ve fallen for the allure of Lua, why not venture further into game development with our comprehensive Roblox Game Development Mini-Degree? This collection of courses will teach you how to create games using Roblox Studio and Lua, covering everything from the basics of Roblox to scripting, melee battle games, and first-person shooter games. Roblox is a hugely popular gaming platform with a community of over 160 million monthly users, offering built-in multiplayer features and easy game publishing, making it a fun and practical way to delve deeper into game development.
Check out our wide range Roblox courses for a broader collection of offerings. Remember, all Zenva’s courses can be accessed 24/7 at your convenience, and you’ll earn completion certificates to showcase your newfound skills!
Conclusion
We hope you’ve enjoyed exploring Lua syntax and patterns with us. Lua is an engaging and practical tool for game development, and harnessing its power offers rewarding opportunities for coders of all skill levels. As you’ve discovered, mastering Lua opens up a world of potential, whether it’s tailoring astounding game scripts on Roblox, customizing gameplay, or even building your own games!
Embarking on this journey might seem daunting, but remember – we’re here to guide you every step of the way! Sharpen your skills with our Roblox Game Development Mini-Degree and get ready to turn your game development dreams into reality. It’s time to begin your coding adventure with Zenva!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
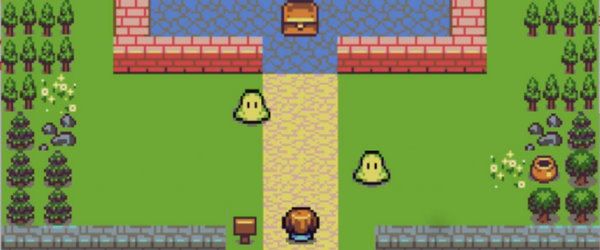
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.