Welcome to an exciting journey into the world of Roblox game pass scripting! This versatile coding skill can open up an array of opportunities for both hobbyists and professionals alike. Whether you’re a coding novice or a seasoned scripter, this tutorial will guide you on how to harness the power of game pass scripting to enhance your Roblox games.
Table of contents
Unlocking Roblox Game Pass Scripting
Before diving into the code, it’s important to have a good understanding of what Roblox game pass scripting is and why it’s worth your time.
What is Roblox Game Pass Scripting?
In a nutshell, game pass scripting in Roblox involves the use of scripts, which are coded instructions, to provide unique experiences for players who’ve purchased a game pass. These could range from character upgrades, special abilities, exclusive areas, and more. The beauty of learning game pass scripting is that it enables you to create immersive and tailored experiences for your players, adding layers of fun and engagement to your games.
What is it for?
Game pass scripting in Roblox significantly contributes to the player’s overall gaming experience. With the right script, you can offer enhanced features, special in-game goods, and exclusive benefits. It’s an awesome way to reward devoted players and also a creative approach to monetize your games.
Why Should I Learn It?
Learning game pass scripting goes beyond just enhancing your games – it allows you to better understand how monetization works within a game design context. Plus, scripting is a fundamental skill in any programmer’s toolkit, making it an invaluable add-on to your coding journey. The multi-faceted benefits of this skill are what make it a worthwhile learning endeavor. Stay tuned and let’s unravel the world of game pass scripting together!
Creating a Game Pass
Before you start scripting, the first step is to create a game pass on the Roblox platform. Once you’ve designed your game pass, you can then start integrating it with your game using scripts.
Setting Up Your Script
Firstly, let’s set up a basic script to check if a player owns a certain game pass. Every script in Roblox starts with referencing the game service, so let’s set it up:
local gamePassID = 12345 -- replace with your own Game Pass ID local MarketplaceService = game:GetService("MarketplaceService") -- function to check game pass ownership function checkGamePassOwnership(player) return MarketplaceService:UserOwnsGamePassAsync(player.UserId, gamePassID) end
In the above code example, replace 12345 with your game pass ID. The MarketplaceService:UserOwnsGamePassAsync() function takes two parameters – the user’s ID and the ID of the game pass – to check if the player owns a specific game pass.
Activating the Game Pass
Once we have the script to check for game pass ownership, we need to write another function which is triggered when the player enters the game. This function will check if the player has the game pass and then grant them the game pass features, if they do.
-- function to activate game pass features function activateGamePassFeatures(player) if checkGamePassOwnership(player) then -- add your game pass feature activation code here.. end end -- event that runs when a player enters the game game.Players.PlayerAdded:connect(activateGamePassFeatures)
Available Game Pass Features
There is a wealth of features you can offer to your game pass owners. Some examples might include:
- Enhanced player speed
- Special abilities or powers
- Access to exclusive areas
- Character upgrades or costumes
These unique features can be coded into the ‘activateGamePassFeatures’ function depending on your game design. This will lead us to the next part of this tutorial, where we’ll delve into specific examples of what you can do with your game pass.
Examples of Game Pass Features
Let’s dive into the exciting part where we turn these game pass scripting concepts into real-world scripts. Below you’ll find multiple examples which you can tweak and use in your own projects.
1. Enhancing Player Speed
You can enhance the walking speed of a player when they own a specific game pass. The code snippet below shows exactly how to achieve this:
function activateGamePassFeatures(player) if checkGamePassOwnership(player) then -- set the player's WalkSpeed to 50 player.Character.Humanoid.WalkSpeed = 50 end end
In this code, we simply check if the player owns the game pass. If true, we amplify their character’s walking speed.
2. Adding Special Abilities
Next up, let’s see how we can grant our game pass owners a special ability. Suppose we wish to endow our players with the ability to fly:
function activateGamePassFeatures(player) if checkGamePassOwnership(player) then -- give the player ability to fly player.Character.Humanoid.FlySpeed = 50 player.Character.Humanoid.JumpPower = 100 end end
Once again, the script checks for game pass ownership, and if true, empowers the player’s character to fly and jump higher.
3. Granting Access to Exclusive Areas
Offering game pass owners access to exclusive areas provides a sense of privilege and excitement. The implications behind featuring exclusive areas are vast, encompassing secret rooms, hidden challenges or even VIP lounges.
function activateGamePassFeatures(player) if checkGamePassOwnership(player) then -- teleport the player to an exclusive area player.Character.HumanoidRootPart.CFrame = CFrame.new(10, 10, 10) end end
This script teleports the player to a specific location (10, 10, 10) in the game, indicating the coordinates of an exclusive area.
4. Offering Character Upgrades or Costumes
Finally, granting cosmetic upgrades or costumes is a classic way to reward your game pass holders. Here’s how you might achieve this:
function activateGamePassFeatures(player) if checkGamePassOwnership(player) then -- change the character's shirt player.Character.Shirt.ShirtTemplate = "rbxassetid://unique_shirt_id" end end
The ‘unique_shirt_id’ should be replaced with your designed shirt’s asset id. Once the player is found to own the game pass, the script changes their character’s shirt to the unique one you’ve created.
With these concepts and examples, you should have a good springboard to start your adventure in game pass scripting. Remember, practice makes perfect when it comes to programming. So go ahead, get creative and enhance your Roblox games with the unique features game pass scripting can endow!
Diving Deeper into Game Pass Features
By now, you’ve familiarized yourself with the basic concept of implementing game pass features in your Roblox games. Let’s further our understanding by exploring additional advanced scripts to make your game pass even more exciting!
Adding Immunities and Strength Buffs
One of the popular gameplay enhancements is the attribute buffs, making players more resilient or stronger in the game. This could easily be accomplished through a game pass. In the following example, we’re granting an immunity bonus to the player, making them invincible to any harm:
function activateGamePassFeatures(player) if checkGamePassOwnership(player) then -- make the player invincible player.Character.Humanoid.MaxHealth = math.huge player.Character.Humanoid.Health = player.Character.Humanoid.MaxHealth end end
In this script, ‘math.huge’ represents an infinitely large number, essentially making the player invincible. This can drastically enhance the gameplay, especially in action-packed or adventure games.
Offering Exclusive In-Game Items
Your game pass can grant exclusive in-game assets to the players. If in your game you have a special weapon or equipment, you can ensure that only game pass owners have access to it.
function activateGamePassFeatures(player) if checkGamePassOwnership(player) then -- grant exclusive asset to the player game.ReplicatedStorage.ExclusiveAsset:Clone().Parent = player.Backpack end end
The example above illustrates how to clone an exclusive item from Roblox’s ‘ReplicatedStorage’ service and store it in the player’s backpack.
Increasing In-Game Currency
If your game has an in-game currency system, you can offer bonus credits to the game pass holders. This is a common feature in many games to encourage more interactive gameplay.
function activateGamePassFeatures(player) if checkGamePassOwnership(player) then -- increase in-game currency for the player player.leaderstats.Money.Value = player.leaderstats.Money.Value + 1000 end end
This script increments the in-game currency of the player by 1000 units, promoting further game interactions and explorations.
Assigning Unique Roles
Finally, one of the advanced ways to reward game pass owners is by assigning them unique roles or titles. Check the example below:
function activateGamePassFeatures(player) if checkGamePassOwnership(player) then -- assign a unique role to the player game:GetService("GroupService"):SetRank(GroupId, player.UserId, RankId) end end
Here, replace ‘GroupId’ and ‘RankId’ with your group’s ID and the ID of the role you want to assign respectively. This script assigns a unique role to the game pass holder within a certain group.
These examples should provide a robust understanding of the potential enhancements you can provide using game pass scripts. Don’t limit yourself to these scripts but use them as a base to get creative and explore myriad possibilities with Roblox game pass scripting. Remember, the joy of game development lies in experimenting, learning, and continually challenging yourself to create amazing gaming experiences!
Continuing Your Roblox Journey
Having mastered the essentials of Roblox game pass scripting, you might be wondering where to head next on your Roblox coding journey. At Zenva, we’ve always believed in the power of continual learning, and we’re here to offer just that with our extensive collection of courses.
Check out our comprehensive Roblox Game Development Mini-Degree where you’ll learn from creating a range of different game genres, getting your hands dirty with Lua scripting, and implementing multiplayer functionality and leaderboards. From obstacle courses to FPS games, there’s a lot to discover and learn! Beginners as well as experienced developers will find these courses very useful.
Furthermore, explore our unique set of Roblox courses to broaden your skills. No matter the level you’re at, we’re sure you’ll find value and build upon your knowledge. As Zenva learners always say – there’s no end to evolving, keep coding and keep creating!
Conclusion
Stepping into Roblox game pass scripting might seem daunting at first, but once you’ve got the hang of it, the opportunities to craft unique gaming experiences are endless. As we’ve shown, this scripting skill can add value to both your games and your personal development as a programmer.
At Zenva, we’re dedicated to providing comprehensive courses that empower you to enhance your coding journey and unlock exciting opportunities. Head over to our Roblox Game Development Mini-Degree and get started on creating captivating gaming experiences today. Let’s step into the inspiring realm of game development together, as one coding community!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
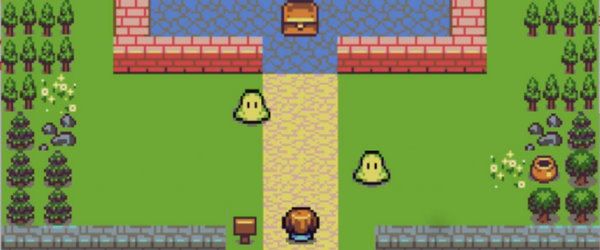
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.