Engaging, extraordinary, and bursting with creativity – that’s the world of Roblox coding. Our focus for today is a fundamental building block of successful Roblox gaming: microtransactions. As content developers in this exciting sandbox platform, understanding the art and design of microtransactions empowers you to build experiences that are not only enthralling but economically rewarding.
Table of contents
What Are Roblox Microtransactions?
Microtransactions, pervasive in modern gaming, are small, in-game purchases that users utilize to access additional content, features, or services. Almost an inseparable part of today’s gaming culture, they’re particularly impactful in platforms like Roblox, where customization and unique game mechanics are so highly valued.
Why Are Microtransactions Important In Roblox?
They contribute to the overall richness and engagement level of games by allowing players to personalize their experiences. More importantly, for developers like us, they create an avenue for monetizing our creative efforts. Roblox’s virtual currency, Robux, which players purchase with real-world money, further facilitates this economic exchange.
Having a clear understanding of microtransactions moves us towards creating better, more engaging games, and potentially, to financial rewards in the Roblox universe.
Setting Up Microtransactions in Roblox
To kick-start our journey in creating microtransactions in Roblox, we need to set the stage with Roblox Studio, Roblox’s development environment. If you don’t have it installed, simply go to the Roblox website and download the platform.
Creating Developer Products
Microtransactions in Roblox come in the form of Developer Products. These are the items that players can purchase multiple times – Let’s go through the process of creating Developer Products.
1. Open the 'View' tab in Roblox Studio and click on 'Developer Products'. 2. Click on the ‘+’ sign next to Developer Products in the explorer to create a new product. 3. Make sure you fill out the Name, Description, and Price (in Robux) fields accordingly.
Once set up, each developer product has a unique ID that you can use when scripting. This bridges the gap between your game logic and the actual purchase by the player.
Coding the Microtransaction: Buying the Product
Now that we have our developer products ready, we need to write the actual script that will process the purchases.
local MarketplaceService = game:GetService("MarketplaceService") function handleTransaction(player, productId) MarketplaceService:PromptProductPurchase(player, productId) end
The above snippet is a function that, when called with a player instance and product ID, prompts the player with a purchase request using the `PromptProductPurchase` function from `MarketplaceService`.
Validating the Purchase
Once the player commits to the purchase, we’ve got to handle their transaction and deliver the product. Thanks to Roblox’s cloud servers, we can use data persistence to save and remember player data even when they aren’t playing. This is done using Roblox’s ProcessReceipt function.
function MarketplaceService.ProcessReceipt(info) -- Get the player and product purchased local playerProduct = MarketplaceService:GetProductInfo(info.ProductId, Enum.InfoType.Product) if(info.PurchaseStatus == Enum.PurchaseStatus.Succeeded) then grantPremium(playerProduct) return Enum.ProductPurchaseDecision.PurchaseGranted else return Enum.ProductPurchaseDecision.NotProcessedYet end end
This function gets called whenever a player purchases a developer product. We then check the purchase status. If the purchase was successful, we grant the product to the player. If not, we keep checking until the status changes.
Granting the Purchase
In the previous snippet, we mentioned a function called `grantPremium`. This is the function that will handle giving our player the respective product, awarding them with the benefits of their purchase.
function grantPremium(playerProduct) -- Get the player who made the purchase local player = game.Players:GetPlayerByUserId(playerProduct.PlayerId) -- Add the benefits if player then player.leaderstats.Level.Value = player.leaderstats.Level.Value + 10 player.leaderstats.Robux.Value = player.leaderstats.Robux.Value + 100 end end
In this specific example, purchasing the developer product would increase the player’s level by 10 and give them an additional 100 Robux. Essentially, what benefits the player receives depends on how you implement this method according to your needs.
Listening for Player Purchases
Remember our `handleTransaction` function? Now we need to set it up so it’s called when the player prompts a purchase. One way to do this is by connecting it to an event, such as a button click in the game.
local purchaseButton = script.Parent -- assuming this script is a child of the button purchaseButton.Activated:Connect(function(player) handleTransaction(player, productId) end)
With this snippet, whenever the button (which is the parent of this script) is clicked by a player, the `handleTransaction` function is triggered, starting the purchase process for the provided productId.
Error Handling
Like any good developer, you should be prepared to handle some errors that may arise during the purchasing process. Roblox’s MarketplaceService provides a useful event for this: `PromptProductPurchaseFailed`.
MarketplaceService.PromptProductPurchaseFailed:Connect(function(player, productId, errorReason) print(errorReason) end)
Here, if the purchase fails, this event fires and provides you with the reason through the `errorReason` parameter, which you can use for debugging and improving your microtransaction process.
As you build your game, it is important to remember the player’s experience in the overall purchase process. User friendly and seamless transactions can greatly improve a player’s satisfaction within your game. Happy coding!
Adding In-Game Assets as Products
Often, you might want the player to be able to purchase in-game assets. Let’s see how we can implement that.
Firstly, setup a “product” table in your script, which contains a list of assets that can be purchased.
local product = { ["Speed"] = { id = 123456, Price = 200 }, ["Strength"] = { id = 789010, Price = 300 }, }
In this example, we have two products, Speed and Strength, each with its respective developer product IDs, and Price in Robux.
Now, let’s adjust our `handleTransaction` method to handle these assets.
function handleTransaction(player, product) MarketplaceService:PromptProductPurchase(player, product.id) end
This function now triggers a purchase request which will show the price set for that developer product in Roblox.
Applying the Purchased Asset
After a successful transaction, we need to apply our product to our player. Continuing from our earlier `grantPremium` function example, we could apply the speed asset gives the player a speed boost and the strength asset gives them extra power.
function grantPremium(playerProduct) local player = game.Players:GetPlayerByUserId(playerProduct.PlayerId) local asset = product[playerProduct.Name] if asset then applyAsset(player, asset) return Enum.ProductPurchaseDecision.PurchaseGranted else return Enum.ProductPurchaseDecision.NotProcessedYet end end function applyAsset(player, asset) -- Apply the asset to the player if asset.Name == "Speed" then player.Character.Humanoid.WalkSpeed = player.Character.Humanoid.WalkSpeed + 10 elseif asset.Name == "Strength" then player.Character.Humanoid.JumpPower = player.Character.Humanoid.JumpPower + 50 end end
These code snippets set up the developer product for two different assets. When a player purchases the “Speed” product, they are granted an additional 10 units to their WalkSpeed. Purchasing the “Strength” product, they receive an additional 50 units to their JumpPower.
Logging Transactions
It is important to keep track of transactions for auditing purposes. Implementing logging can be an excellent way to accomplish this.
function logTransaction(playerId, asset, status, info) print(os.date().." - Transaction: "..tostring(asset.Name)) print("Player: "..tostring(info.PlayerId)) print("Status: "..tostring(info.PurchaseStatus)) print("-------------") end -- And add the logTransaction function to our process receipt method function MarketplaceService.ProcessReceipt(info) local playerProduct = MarketplaceService:GetProductInfo(info.ProductId, Enum.InfoType.Product) local asset = product[playerProduct.Name] if info.PurchaseStatus == Enum.PurchaseStatus.Succeeded then applyAsset(playerProduct, asset) logTransaction(playerProduct.PlayerId, asset, "Success", info) return Enum.ProductPurchaseDecision.PurchaseGranted else logTransaction(playerProduct.PlayerId, asset, "Failed", info) return Enum.ProductPurchaseDecision.NotProcessedYet end end
This function logs the date of transaction, the name of the product, the player’s Id, and the status of the purchase. We then modified the `ProcessReceipt()` function to call this function after a purchase attempt.
Getting better at this requires practice. It’s crucial to continually expand and stabilize the in-game economic ecosystem, enhancing retention, and enjoyment of your games.
With the power of microtransaction implementation via Roblox coding in your hands, your journey into the thrilling world of game development is just beginning. You’ve seen the surface of game monetization, and we are certain you’re intrigued by the potential.
Zenva’s Roblox Game Development Mini-Degree is an excellent next step on your path to mastery. It provides an in-depth exploration of game creation using Roblox Studio and Lua, covering topics from basic level creation to scripting complex games like melee battles and first-person shooters. This comprehensive program caters to both beginners and experienced programmers, is project-based, and flexible, allowing you to learn at your pace and on any device.
For a broader learning experience, you can also embark on a variety of exciting projects covered in our Roblox category. At Zenva, our mission is to empower your journey from a beginner to a professional, while providing the most up-to-date content. Who knows? One day, you might be the creator of the next viral game in Roblox!
Conclusion
Understanding and implementing microtransactions effectively in your Roblox games could very well be the stepping stone that lifts your game from a hobby to a financially successful venture. Giving your players the opportunity to spend their Robux in your game provides a personalized experience and fuels your creativity to new heights.
Leap forward in your game programming journey with our comprehensive Roblox Game Development Mini-Degree. It’s time for you to write your own success story in the dynamic universe of game development. We can’t wait to see what you create!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
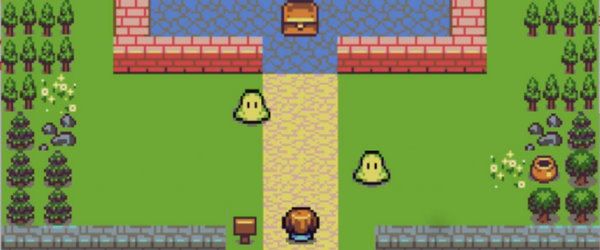
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.