If you’ve ever dreamed of creating your own video games, the world of Roblox offers an excellent platform to turn your dreams into reality. This popular gaming platform not only allows you to play games created by other users but also enables you to build and script your own games, significantly contributing to the expansive Roblox universe.
Table of contents
What is Roblox Scripting?
In the Roblox environment, scripting is the process of using a programming language called Lua to create interactive elements in your game. Lua is a lightweight, highly flexible scripting language which has been adapted to suit Roblox development requirements.
Why Learn Roblox Scripting?
Roblox scripting unlocks the realm of customization allowing you to create interactive games, immersive environments, and engaging gameplay mechanics. Beyond game creation, understanding Roblox scripting can:
- Enhance your problem-solving skills as scripting involves logical thinking and the ability to solve complex issues.
- Provide a solid foundation for learning other scripting languages, improving your future adaptability in the ever-changing field of game development.
Gracefully mastering Roblox scripting could even turn into an income stream, given the platform’s popularity and the growing demand for unique gaming experiences.
Stay with us as we delve deeper into the world of Roblox scripting. The journey is definitely worth it.
Basics of Roblox Scripting: Variables
In Roblox scripting, variables are utilized to store information. Here’s how you declare a variable in Lua:
local playerName = "John"
In this example, “playerName” is the variable, and it’s storing the string “John”.
We could also store numbers or Boolean values in variables:
local playerAge = 15 local isPlayerOnline = true
The word “local” defines the scope of the variable. A local variable can only be accessed within the chunk or the block where it is declared.
Conditionals in Roblox Scripting
Conditionals are used to perform different actions based on different conditions.
local playerScore = 100 if playerScore >=50 then print("You passed!") else print("You failed!") end
In the script above, if a player’s score is greater than or equal to 50, the console will print “You passed!” and if it’s less than 50, it will print “You failed!”.
Loops in Roblox Scripting
A loop statement allows us to execute a statement or group of statements multiple times.
for i=1, 5 do print("Hello, Roblox!") end
In the script above, the message “Hello, Roblox!” will be printed five times.
Functions in Roblox Scripting
Functions in Roblox scripting help us to structure code into manageable blocks. Below is a simple function to calculate the sum of two numbers.
function addNumbers(num1, num2) local sum = num1 + num2 return sum end print(addNumbers(5,3))
This script will add 5 and 3, and then print the result which is 8.
These examples cover some of the basics of Roblox scripting. Practice writing, running, and modifying these scripts on your own to better understand how each concept works.
Tables in Roblox Scripting
Tables are one of Lua’s key tools, and they are fundamentally a collection of key-value pairs.
local player = {name = "John", score = 100, level = 15} print(player.name) print(player.score) print(player.level)
In this script, a table named “player” is created with three key-value pairs. When we print the values associated with each key, Lua will output “John”, “100” and “15”.
Manipulating Tables
Once constructed, tables can also be manipulated. Let’s take a look at how to add, modify and remove elements from our table.
player.coins = 50 player.score = 150 player.level = nil print(player.name) print(player.score) print(player.level) print(player.coins)
In the script above, we added a new key-value pair (coins = 50), modified an existing one (score = 150), and removed another by setting its value to nil (level). Now when we print the values, Lua will output “John”, “150”, “nil” and “50”.
Event-driven Programming in Roblox Scripting
An important aspect of Roblox scripting is event-driven programming. Instead of following a linear process, your code waits until specific actions or “events” take place, then executes the corresponding code.
game.Players.PlayerAdded:Connect(function(player) print(player.Name .. " has joined the game") end)
This code snippet listens for the “PlayerAdded” event, and prints a message when a new player joins the game.
Accessing Roblox API
As part of its environment, Roblox provides a rich API for developers. Here’s an example of accessing it to manipulate an in-game object:
local p = game.Workspace.Part p.BrickColor = BrickColor.new("Bright red")
In this example, we’re changing the color of a brick part located in the game’s workspace to bright red. Taking full advantage of Roblox’s API is a significant step towards creating engaging and dynamic games.
These further examples provide a deeper understanding of Roblox scripting. Through practice and exploration, you’ll be able to leverage these concepts to create your own Roblox games!
Interacting With In-Game Objects
A key part of creating engaging games in Roblox is the ability to interact with in-game objects through scripting. You can change properties, attach scripts to objects, and create complex interconnections between different game elements.
Consider a simple Roblox brick. You can make the brick disappear using the following code snippet:
game.Workspace.Brick:remove()
On the other hand, if you want to increase the size of the brick, you could use:
game.Workspace.Brick.Size = Vector3.new(10, 10, 10)
Implementing physics-related changes is also possible. For instance, if you want a brick to bounce higher, you can adjust its Elasticity:
game.Workspace.Brick.Elasticity = 0.9
Managing Player Input in Roblox Scripting
One of the crucial aspects of game development is managing and reacting to player input. In Roblox, a built-in UserInputService allows you to capture input from players, whether they’re playing on desktop, mobile, or any other supported platform.
Here’s an example of how you can listen for a keyboard key press:
local InputService = game:GetService("UserInputService") InputService.InputBegan:Connect(function(input) if input.KeyCode == Enum.KeyCode.W then print("The W key was pressed") end end)
In the example above, if the player presses the ‘W’ key, the console will print “The W key was pressed”.
Utilising Roblox Services
Roblox offers more than 50 services that enable developers to add features ranging from messaging systems to pathfinding algorithms.
Take, for instance, the TweenService which can be used to animate properties of an object over time. Here’s an example showing how to change the position of a brick using a Tween:
local TweenService = game:GetService("TweenService") local Part = game.Workspace.Part local TweenInfo = TweenInfo.new(5) local Goals = {Position = Vector3.new(10,10,10)} local newTween = TweenService:Create(Part,TweenInfo,Goals) newTween:Play()
In this script, we first create a new TweenInfo object that describes the properties of the Tween. The Tween is then created and connected to a Part with the described properties. Finally, the tween is played, moving the Part to the new specified Position over 5 seconds.
Remember, mastering code takes time, patience and practice. We hope these code examples inspire you to dive deeper into the awesome opportunities that lie ahead in Roblox scripting!
Continuing Your Roblox Scripting Journey
So you’ve got the basics down and have dipped your toes into the world of Roblox scripting. Fantastic! The journey, however, doesn’t end here. In fact, you’ve just embarked on an exciting path that could truly transform your gaming and development experience.
We believe that learning continues beyond the first step. If you’re excited about taking your Roblox development skills further, we invite you to explore our Roblox Game Development Mini-Degree. This comprehensive program immerses you in the world of Roblox Studio and Lua, guiding you as you create a wide range of games, from obstacle courses to combat games, and even multiplayer titles with leaderboards.
If your interest extends beyond the mini-degree, we also offer a broader content selection in our Roblox catalog.
Remember, each step forward brings you closer to creating engaging Roblox games, developing a professional portfolio, and gaining marketable skills. With Zenva, you can truly go from beginner to professional.
We look forward to being part of your coding journey. So ready to level up your Roblox scripting? Let’s go! Your game is waiting to be built.
Conclusion
Whether it’s creating a narratively-rich adventure, an action-packed combat game, or a challenging obstacle course, Roblox scripting provides an accessible yet powerful platform for bringing your gaming ideas to life. At Zenva, we believe the possibilities are limitless, limited only by the reach of your imagination.
We’re thrilled to accompany you on this vibrant journey of discovery and creativity. So why not take the next step? Dive into our Roblox Game Development Mini-Degree and see where your creativity can lead. Remember, every great game developer started at the beginning, just like you. Embrace the process, and let’s build some fantastic games!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
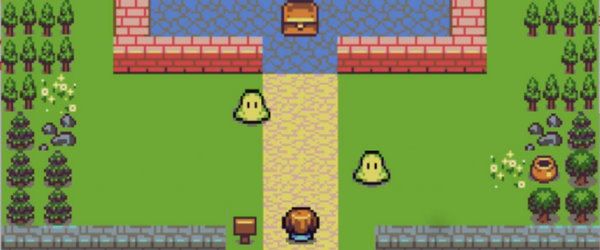
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.