We’re about to embark on an exciting journey of Python programming, where we explore a simple, yet miraculous feature of the language – variable swapping.
Table of contents
What is Python Variable Swapping?
Python variable swapping refers to the elegant ability Python gives us to exchange the values of two variables in a single line of code without the need for a temporary storage variable, a small but powerful trick that can simplify your coding process.
Python’s syntax for variable swapping is incredibly intuitive and beginner-friendly. It reduces clutter in your code and streamlines your operations, ensuring your programs are more readable and efficient. In games and complex algorithms, this feature is a real game-changer!
Interested in mastering Python and taking your programming skills to the next level? Why not explore our Python Mini-Degree program? At Zenva, we support learners at the start of their coding journey and beyond, offering high-quality content in programming, game creation, and AI.
Getting Started with Python Variable Swapping
Now, let’s dive into the coding part of Python variable swapping. Below is a simple example:
# Let's declare two variables player1 = "Alice" player2 = "Bob" # Python allows variable swapping like this player1, player2 = player2, player1 print(player1) # This will output: Bob print(player2) # This will output: Alice
In the block of code above, we initially assign two string values to “player1” and “player2”. Later in the code, we perform a variable swap operation. As a result, “player1” will now hold the name “Bob” and “player2” will hold the name “Alice”.
Understanding Python Variable Swapping
Variable swapping works for multiple variables too, not just two! We extend the concept of variable swapping from the last example for swapping three variables.
# Let's declare three variables player1 = "Alice" player2 = "Bob" player3 = "Charlie" # Python allows multiple variable swapping like this player1, player2, player3 = player3, player1, player2 print(player1) # This will output: Charlie print(player2) # This will output: Alice print(player3) # This will output: Bob
Part II – Diving Deeper into Python Variable Swapping
Now that we have a fair understanding of Python variable swapping, let’s broaden our horizon to fully comprehend the power of Python’s variable swapping mechanism.
Example 1: Swap Integers
Python’s variable swapping isn’t just restricted to strings. You can swap other data types as well.
# Declare two integers num1 = 10 num2 = 20 # Swap the variables num1, num2 = num2, num1 print(num1) # This will output: 20 print(num2) # This will output: 10
Example 2 – Swapping Lists
To further illustrate the versatility of Python’s variable swapping, here’s how to swap the values of two lists:
# Declare two lists list1 = ["Alice", "Charlie"] list2 = ["Bob", "Dianne"] # Swap the variables list1, list2 = list2, list1 print(list1) # This will output: ["Bob", "Dianne"] print(list2) # This will output: ["Alice", "Ethan"]
Example 3 – Swapping Nested Variables
Python’s variable swapping isn’t just for single variables. You can apply it to nested variables as well!
# Declare some nested variables player1 = ["Alice", 100] player2 = ["Bob", 200] # Python allows nested variable swapping like this player1[1], player2[1] = player2[1], player1[1] print(player1) # This will output: ["Alice", 200] print(player2) # This will output: ["Bob", 100]
Part III – Python Variable Swapping Advanced Concepts
Example 4: Swapping Values with Operators
In Python, you can swap variables using arithmetic operators. While it’s not as concise as the previous method, it’s good to know it can be done.
# Declare two variables x = 5 y = 10 # Swap using arithmetic operators x = x + y y = x - y x = x - y print(x) # This will output: 10 print(y) # This will output: 5
Even though Python already simplifies variable swapping, understanding how to swap variables using arithmetic operators is extremely useful, particularly if you come across a language without Python’s swapping capability.
Example 5: Swapping Values in a Dictionary
What if we have a dictionary and we want to swap values of two keys? Python’s got us covered!
# Declare a dictionary player_scores = {"Alice": 10, "Bob": 20} # Swap the scores of Alice and Bob player_scores["Alice"], player_scores["Bob"] = player_scores["Bob"], player_scores["Alice"] print(player_scores) # This will output: {"Alice": 20, "Bob": 10}
As you can see, Python brings variable swapping to another level by allowing value swapping even in data structures like dictionaries.
Part IV – Python-Variable Swapping in Data Storage and Algorithms
Let’s extend our journey to learn how Python variable swapping can be used in different areas. By featuring more code examples in this section, we will demonstrate how variable swapping is not just an interesting concept, but is practical and widely applicable.
Example 1 – Swapping Values in Tuples
Similar to other Python data types, swapping in tuples can also be achieved seamlessly.
# Declare a tuple players = ("Alice", "Bob") # Swap the players players = players[::-1] print(players) # This will output: ("Bob", "Alice")
Tuples are immutable, but by slicing the tuple in reverse order, we can achieve the variable swapping effect.
Example 2 – Swapping Values in Matrices
Not to be left behind, matrices – a crucial aspect in many fields like machine learning and game development – can also embrace the magic of swapping.
# Declare a matrix matrix = [[1, 2], [3, 4]] # Swap the values matrix[0][0], matrix[1][1] = matrix[1][1], matrix[0][0] print(matrix) # This will output: [[4, 2], [3, 1]]
This swapping capability can be a handy tool while working with large and complex matrices.
Example 3 – Improving Algorithms with Swap
One of the most classic examples of the utility of variable swapping is in sorting algorithms.
# Declare a list numbers = [5, 2, 1, 8, 4] # Perform bubble sort which constantly swaps values for i in range(len(numbers)): for j in range(len(numbers) - i - 1): if numbers[j] > numbers[j + 1]: # Swap! numbers[j], numbers[j + 1] = numbers[j + 1], numbers[j] print(numbers) # This will output: [1, 2, 4, 5, 8]
Swapping becomes particularly useful in algorithms such as bubble sort, where we continuously swap elements until we have a sorted list.
Example 4 – Reversing a String
Last but not least, Python’s swapping feature can be used for reversing a string in a creative way.
# Declare a string word = "Python" # Convert the word into a list word_list = list(word) # Swap characters to reverse the list for i in range(len(word_list) // 2): word_list[i], word_list[-i-1] = word_list[-i-1], word_list[i] # Convert the list back into a string reversed_word = "".join(word_list) print(reversed_word) # This will output: "nohtyP"
By turning a string into a list and applying swapping, you can reverse a string effortlessly.
Where to Go Next?
Python’s variable swapping feature might seem like a small component in the broad spectrum of Python programming, but it’s these little details that combine to form a powerful, versatile and highly sought-after language.
If you want to learn more Python, though, we highly recommend our Python Programming Mini-Degree program. This comprehensive program provides a wide variety of Python courses that walk through coding basics, explores advanced algorithms, introduces game and app development, and much more.
You might be wondering, why should I pursue the Python Programming Mini-Degree at Zenva?
- Learn from experienced and certified instructors: Our trainers master Python programming and deliver the courses effectively making your learning journey smooth.
- Engage in hands-on learning: With our coding challenges and project-based approach, you aren’t merely learning the language, you are also practicing it in real-world scenarios.
- Flexibility of learning: You can learn at your own pace and skip to the lessons that are most relevant to your current learning needs.
- Certificates of completion: Upon finishing the courses, you receive completion certificates that can enhance your job profile.
For learners who are interested in a wider variety of Python topics, we have a comprehensive collection of Python courses that cover a vast range of fundamental and advanced concepts.
Conclusion
In the world of programming, every detail counts and a feature as simple as Python’s variable swapping can be the difference between a good program and a great one. Hopefully, this journey through Python’s variable swapping has left you intrigued, awakened your curiosity and proved this rarely hailed feature’s brilliance.
At Zenva, we are dedicated to making your learning process engaging and enlightening. Whether you are a novice in the realm of coding or an experienced programmer seeking to learn more, we have got it all covered. Our Python Programming Mini-Degree program offers a comprehensive Python learning experience – from the basics to advanced topics. Our hands-on approach ensures you not just learn Python; you also practice, experiment, and empower yourself with it. Happy coding, everyone! Keep exploring, keep growing with Zenva. Your coding voyage just got more exciting!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
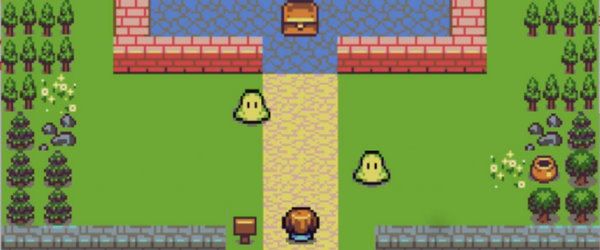
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.