Welcome! In today’s tutorial, we’ll be navigating the fascinating world of Python Byte Objects. As an integral part of Python programming language, understanding byte objects not only boosts your coding skills but also enhances your overall grasp of Python. Whether you are at the start of your coding journey or an experienced programmer, this tutorial is designed to be engaging, valuable, and most of all – accessible.
Table of contents
What Are Python Byte Objects?
In Python, Byte objects are sequences of Bytes – units of digital information that is built of 8 bits. Byte objects are immutable, which means they cannot be changed after they are created.
Byte objects are incredibly useful when dealing with binary data that needs to be stored or transferred over networks.
Understanding Python byte objects is paramount for several reasons:
- It increases your versatility and range as a Python programmer.
- Provides you the ability to deal with binary data, a must when working with files, images or networks.
- Opens up new avenues for understanding and handling data in Python.
So, without further ado, let’s dive into the world of Python Byte objects!
Creating Byte Objects
In Python, byte objects can be created in a few ways. We’ll kick things off with a simple one.
# Creating a byte object from a string with the default 'UTF-8' encoding data = bytes("Hello, Python!", 'UTF-8') print(data) # It should output: b'Hello, Python!'
We can also create bytes object with integers. Here’s how:
# Creating a byte object from a list of integers data = bytes([10, 20, 30, 40, 50]) print(data) # It should output: b'\n\x14\x1e(2'
Accessing Byte Objects
Here’s how to access elements within a byte object:
# Creating a byte object data = bytes("Hello, Python!", 'UTF-8') print(data[7]) # It should output: 80
Remember that byte objects are immutable. This means that you cannot change the value of any index.
# Attempting to change an element within a bytes object data[1] = 65 # It will return a TypeError: 'bytes' object does not support item assignment
Iterating Through Byte Objects
Byte objects can be iterated in a way similar to other iterable objects in Python.
# Creating a byte object data = bytes("Hello, Python!", 'UTF-8') # Iterating through the byte object for byte in data: print(byte)
On execution of the above code, you’ll see each byte in the object printed on a new line.
Bytearray Objects
An incredibly useful variant of byte objects is bytearray objects. They are similar to byte objects but are mutable – which means elements in bytearray objects can be changed.
Creating bytearray objects might seem familiar:
# Creating a bytearray object from a string input mutable_data = bytearray("Hello, Python!", 'UTF-8') print(mutable_data) # It should output: bytearray(b'Hello, Python!')
Modifying Bytearray Objects
Unlike bytes objects, elements of bytearray objects can be modified:
# Altering a value within a bytearray object mutable_data[1] = 65 print(mutable_data) # It should output: bytearray(b'HAllo, Python!')
Working with Files
Python byte objects are instrumental when dealing with binary file operations. Here’s an example:
# Writing binary data to a file with open("data.bin", "wb") as file: file.write(bytes([65, 66, 67, 68]))
This code creates a binary file named ‘data.bin’ and writes the binary representation of [65, 66, 67, 68].
# Reading binary data from a file with open("data.bin", "rb") as file: print(file.read())
The above code reads and prints the binary data from ‘data.bin’. It should output ‘b’ABCD”.
Converting Byte Objects
Here’s how to convert byte objects to a list of integers:
# Converting a bytes object to a list of integers data = bytes("Hello, Python!", 'UTF-8') print(list(data))
This will output a list of integers corresponding to the unicode of each character.
Where to Go Next?
As we’ve seen throughout this tutorial, Python’s byte and bytearray objects play a critical role in handling binary data. But this is just the tip of the iceberg. There’s a massive world of Python programming waiting to be explored, and now that you’ve got the hang of byte objects, you’re well on your way!
If you’re interested in going further with Python, we recommend checking out our Python Mini-Degree at Zenva Academy. This comprehensive collection of courses provides an excellent groundwork in Python programming, covering everything from coding basics, algorithms, and object-oriented programming, to game development and app development.
What makes our Python Mini-Degree an important asset for continuing learners? Let’s take a look:
- The courses include hands-on projects and quizzes to reinforce learning.
- Learners can build a portfolio of Python projects and earn completion certificates.
- The courses are taught by qualified instructors and can be accessed on any device.
- The content is regularly updated to stay current with industry practices.
We also invite you to browse our Python courses to find the right path for you.
Conclusion
Congratulations! You’ve just finished an essential chapter in your Python learning journey. Mastery over Python byte and bytearray objects empowers you to handle binary data like a pro, paving the way to explore complex Python functionalities. But remember, every end is a new beginning!
The world of Python is vast and exciting, waiting for you to dive deeper. Continue learning and exploring with us at Zenva by going further with our comprehensive Python Mini-Degree. Whether you’re aiming to build a career in programming or wish to elevate your existing skills, we’re here to guide you at each step. Let your Python learning journey grow beyond boundaries – stay inspired, keep coding, and shape the future the way you want!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
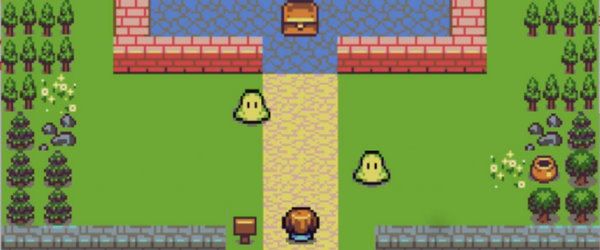
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.