In this tutorial, we will dive into the exciting world of Python variables. Variables are like containers in coding; they store data values and make our programs more efficient and flexible. If you’re looking to enhance your programming skills or kick-start your coding journey, understanding Python variables is key.
Table of contents
What are Python Variables?
In Python, variables allow you to store and manipulate information in your code. Think of them as labels for pieces of data that our program can recall or alter whenever it needs to.
Learning about variables is essential for anyone interested in coding, especially in Python, which is known for its simplicity and readability. They form the backbone of any coding task and are integral parts of functions, control flow, and data structures. By understanding variables, you can write more flexible, efficient, and powerful code to solve complex programming problems.
Now that we’ve understood the importance of Python variables, let’s explore them through some interesting examples.
Declaring Variables in Python
In Python, declaring a variable is as simple as giving it a name and assigning it a value. Here’s an example:
var = 10 print(var)
In the above code, we’ve declared a variable named ‘var’, assigned it the value 10, and then printed it out.
Python is a type-inferred language, meaning you don’t have to specify a variable’s data type; Python automatically determines it based on the value assigned. Here’s an example:
name = "Zenva" print(type(name))
In the code above, Python automatically identifies ‘name’ as a string variable.
Working with Multiple Variables
In Python, you can declare and assign values to multiple variables simultaneously. Let’s look at an example:
x, y, z = "GameDev", "Academy", "Zenva" print(x) print(y) print(z)
In the above code, we’ve simultaneously declared three variables and assigned values to them.
Global and Local Variables
In Python, variables can be divided into two basic types, global variables and local variables.
Global variables are declared outside a function and can be used anywhere in the program.
globalVar = "I am a global variable" def fun(): print(globalVar) fun()
In contrast, local variables are declared inside a function and can only be used within that function.
def fun(): localVar = "I am a local variable" print(localVar) fun()
In the next part of our tutorial, we will delve deeper into Python variables. So, keep reading!
Variable Reassignment in Python
One of Python’s great features is how easy it is to reassign values to variables. This gives your code extra flexibility, as shown in the following example:
var = 10 print(var) var = 20 print(var)
In the example above, we initially declared ‘var’ as 10. However, we later reassigned it as 20.
Mutability and Immutability in Python
Depending on the data type, variables in Python can be mutable (changeable) or immutable (unchangeable). Lists, for example, are mutable:
list_var = [1, 2, 3] list_var[0] = 4 print(list_var)
However, strings and tuples are examples of immutable types:
string_var = "hello" string_var[0] = "H"
Executing the above code will return a TypeError, because we’re trying to change an immutable object.
Copying Variables in Python
Python lets us copy one variable’s value into another variable, like this:
original_var = 10 copied_var = original_var print(copied_var)
In the above code, ‘copied_var’ has a copy of ‘original_var’s value.
However, keep in mind that when copying mutable variables like lists, any modifications to the copied variable will also affect the original one.
original_list = [1, 2, 3] copied_list = original_list copied_list[0] = 4 print(original_list)
The original list changed, even though we only modified the copied one. To avoid this, we use the ‘copy()’ function, like this:
original_list = [1, 2, 3] copied_list = original_list.copy() copied_list[0] = 4 print(original_list)
Now, modifying the copied list doesn’t affect the original one.
With these examples, we conclude our in-depth look into Python variables. This foundational knowledge will empower you to write more effective and efficient Python code. Happy coding!
Where to Go Next / How to Keep Learning?
Now that you’ve dipped your toes in Python variables, you might be wondering what the next step is. How do you continue learning and mastering Python? The answer is straightforward – through our comprehensive Python Mini-Degree!
Our Python Mini-Degree is a complete collection of Python programming courses. It journeys you through various topics, such as:
- Coding basics
- Algorithms
- Object-oriented programming
- Game development
- App development
By going through these courses, you can build your own games, apps, and real-world projects. All our courses are designed with flexibility in mind. Whether you’re a beginner or an experienced programmer looking to refine your skills, we have the course for you. Our sessions include interactive lessons, quizzes, and coding challenges to reinforce what you’ve learned and help you apply new skills.
Not only will you learn, but you’ll also develop a solid portfolio of Python projects. You even get to earn completion certificates, which are fantastic pieces of evidence for your programming prowess. Rest assured that our courses are regularly updated to align with industry trends and developments.
And for those seeking a broader collection, do check out our full range of Python courses.
Conclusion
As we wrap up this tutorial, we hope you’ve gained valuable insights into Python variables. This fundamental programming topic is essential in crafting efficient and powerful code, and gaining mastery over it will greatly aid in your coding journey.
No matter where you’re at in your coding journey, Zenva is instrumental in helping you build or enhance your programming skills. With our comprehensive and in-depth courses such as the Python Mini-Degree, you will be prepared to take on any programming challenge. So, why wait? Let’s dive in and start shaping your coding future today!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
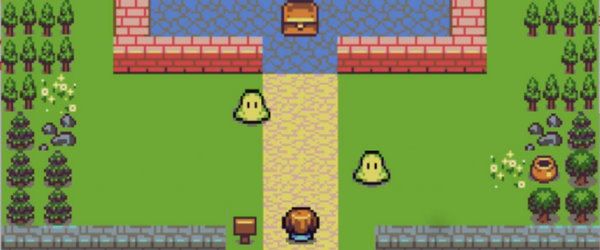
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.