Welcome to an exciting journey towards understanding Python Modules – a key concept that can greatly reinforce your programming foundation and enlarge your coding toolkit. The varied applications, ease of use, and immense functional value of Python Modules contribute generously to the success of Python as one of the most dominant programming languages.
Table of contents
What are Python Modules?
Python Modules are essentially code libraries – separate Python script files that contain various classes, functions, variables, and even other modules. These are reusable pieces of code designed to perform specific tasks, which you can include in your programs whenever needed, thereby promoting code reuse, modularity, and efficient project organization.
Why are Python Modules important?
Just as pieces of a jigsaw puzzle come together to create a bigger picture, Python Modules efficiently bind together smaller blocks of code into a cohesive and large Python program. They help organize code, make it cleaner and easier to read, and reduce the complexity of large programs.
Why Learn About Python Modules?
Python Modules add a significant edge to your coding arsenal. Here are a few reasons to learn them:
- The knowledge of Modules can aid you in writing more organized, maintainable, and scalable code.
- They can help you in reducing the repetition in your codebase, thereby following the DRY (Don’t Repeat Yourself) principle of coding.
- Mastering Modules complements your in-depth learning of Python, fostering comprehension of its coding best practices.
As we delve deeper into the world of Python Modules, we’ll realize their immense potential in creating thrilling gameplay mechanics. So are you ready to commence this captivating module-thon?
Creating and Using Python Modules
First things first, let’s understand how to create a basic Python module. For instance, you might have a file named ‘greetings.py’ with the following function:
def hello(name): return f"Hello, {name}!"
This ‘greetings.py’ becomes a Python Module named ‘greetings’, ready to be used in other programs.
To use a module, we need to import it. Understanding the different ways to import a module can give you flexibility in how you use them:
import greetings print(greetings.hello("Zenva"))
import’ allows us to access all functions, variables, classes etc. from the ‘greetings’ module. You can also import specific functions:
from greetings import hello print(hello("Zenva"))
Working with Standard Python Modules
Python comes with a robust selection of standard modules. Let’s exemplify this with a few standard Python modules:
The math module, used for mathematical operations:
import math print(math.sqrt(16))
The datetime module, used for dealing with dates and times:
from datetime import date print(date.today())
The random module can enable game developers to build unpredictability into their games:
import random print(random.randint(0, 10))
Exploring the OS Module
The OS module in Python provides a way of using operating system dependent functionality. This is a powerful tool with many uses, particularly in working with file and directory management.
Let’s see how you can use the os module to print your current working directory using os.getcwd()
:
import os print(os.getcwd())
The os.mkdir()
function allows you to create a directory:
os.mkdir('test')
To rename a file or a directory, you can use the os.rename()
function. Remember, you need the file or directory to exist for this operation to be possible:
os.rename('test', 'new_test')
The os.remove()
function allows you to delete a file:
os.remove('myfile.txt')
Deep Dive into Collections Module
Packed with high-performance container datatypes beyond lists, dict, and tuples, the collections module is a must-know for Python programmers.
You can use the Counter class to count the frequency of elements in a list:
from collections import Counter mylist = ['apple', 'banana', 'apple', 'banana', 'apple', 'kiwi'] counter = Counter(mylist) print(counter)
The OrderedDict class will remember the order in which items were inserted:
from collections import OrderedDict ordered_dict = OrderedDict() ordered_dict['a'] = 1 ordered_dict['b'] = 2 ordered_dict['c'] = 3 print(ordered_dict)
Exploring different modules and exploring their functions, classes, and variables helps you familiarise yourself with Python’s out-of-the-box abilities. Keep practising and trying out different modules – implementation is the best form of learning!
Where To Go Next? How to Keep Learning?
We’ve opened the doors to the world of Python modules for you. Armed with this knowledge, you have the power to embark on programming adventures. But, the learning journey has only just begun.
We highly recommend continuing this journey with our comprehensive Python Mini-Degree. This course collection offers a well-rounded dive into Python programming.
Our experts at Zenva have meticulously designed these courses to take learners beyond the basics. From coding fundamentals, algorithms, and object-oriented programming, to game development and app creation with popular libraries like Pygame, Tkinter, and Kivy, we’ve got you covered.
Python’s top position in the programming language charts and its widespread industrial demand makes this a valuable learning pursuit. The courses are regularly updated to keep pace with industry developments, ensuring your learnings remain relevant and impactful.
Whether you’re starting from scratch or have some prior programming experience, these courses adapt to your learning requirements. They offer flexible learning options, real-world project-based assignments, and helpful support from expert mentors.
We also offer intermediate-level Python courses for those looking to delve deeper into the fascinating world of Python. Continue enhancing your skills and slowly but steadily, you’ll evolve from a beginner to a professional in Python programming.
Conclusion
Python modules form an integral part of Python programming, transforming your code from basic snippets to scalable, efficient applications. This aspect of Python equips you with the ability to write more maintainable code, making it a go-to tool for any programmer.
In the vast universe of Python programming, every step, every new concept learned, takes you closer to mastery. With the Python Mini-Degree provided by Zenva, you’re not just learning – you’re evolving, you’re creating a brighter future in the domain of coding. Remember, every great developer was once a beginner who never gave up. So, keep learning, keep programming!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
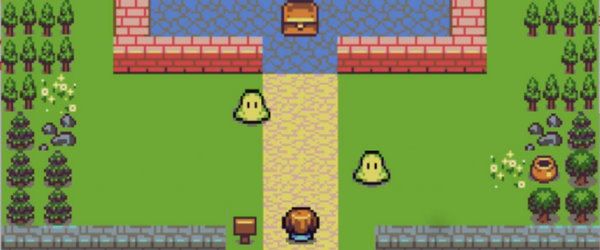
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.