Welcome to this comprehensive guide on Python exception handling. Whether you’re just beginning your coding journey or already have some experience under your belt, this interactive tutorial will equip you with the knowledge you need to write robust and error-resilient Python programs. So, let’s dive in and explore this fundamental Python concept!
Table of contents
What is Python Exception Handling?
Python exception handling is a method for managing and responding to errors that occur during program execution. These errors, also known as exceptions, can disrupt a program’s normal flow, causing it to abruptly terminate if not properly addressed.
Exception handling serves the important purpose of controlling how our programs behave when they encounter errors. It helps to ensure that our applications run smoothly and without interruption, regardless of unforeseen and erroneous program inputs or conditions. It’s like a safety net, catching errors before they cause our programs to crash and burn.
Without exception handling, a single error can crash your entire program, leading to a poor user experience. With exception handling, Python allows us to anticipate, detect, and respond to these errors, thus improving the resiliency of our programs and enhancing user experience. Let’s look at a simple example to cement our understanding of exception handling in Python.
Basics of Python Exception Handling
Python has several built-in exceptions which include `IOError`, `ValueError`, `ZeroDivisionError`, `ImportError`, `NameError`, `TypeError` and many more. We’ll start with a simple program without exception handling.
num1 = 10 num2 = 0 result = num1 / num2 print(result)
Running this code will result in a `ZeroDivisionError` because a number cannot be divided by zero. Let’s modify our program to include exception handling.
Using the Try/Except Block
The `try/except` block is the fundamental building block of Python exception handling.
num1 = 10 num2 = 0 try: result = num1 / num2 print(result) except ZeroDivisionError: print("Sorry, you can't divide by zero.")
Running this code will print “Sorry, you can’t divide by zero.” instead of throwing an error and halting the program.
Using the Else Clause
We can use the `else` clause in our `try/except` block to specify code that will only run if the `try` block does not generate an exception.
num1 = 10 num2 = 5 try: result = num1 / num2 except ZeroDivisionError: print("Sorry, you can't divide by zero.") else: print("The result is", result)
Running this code will print “The result is 2.0”.
Using the Finally Clause
We can use the `finally` clause in our `try/except` block to specify code that will always run, whether an exception is thrown or not.
num1 = 10 num2 = 0 try: result = num1 / num2 except ZeroDivisionError: print("Sorry, you can't divide by zero.") finally: print("This is the finally block.")
Running this code will print “Sorry, you can’t divide by zero.” then “This is the finally block.”
And with that, we have covered the necessary basics of Python exception handling. We’ll get into more advanced topics in the next section. Stay tuned!
Raising Exceptions
We can use the `raise` statement to throw an exception if a certain condition is met. This is particularly helpful in triggering exceptions on purpose to test our exception handling.
num = -5 if num < 0: raise ValueError("Negative numbers are not allowed!")
Running this code will throw a `ValueError` with the message “Negative numbers are not allowed!”.
Creating Custom Exceptions
Python also allows us to create and use custom exceptions. To do this, we simply define a new class that inherits from the `Exception` superclass.
class CustomError(Exception): pass raise CustomError("This is a custom exception")
Running this code will throw a `CustomError` with the message “This is a custom exception”.
Catching Multiple Exceptions
A `try` block can catch multiple exceptions using multiple `except` clauses.
try: num = int('Zenva') except ValueError: print("Value error!") except TypeError: print("Type error!")
This code will print “Value error!” since creating an int from a string that does not represent a number results in a `ValueError`.
Handling Exceptions with the same `except` Block
Numerous exceptions can also be caught by a single `except` block, by passing the errors in a tuple,
try: num = int('Zenva') except (ValueError, TypeError): print("There was an error!")
This code will print “There was an error!” since creating an int from a string that does not represent a number results in a `ValueError`.
Python’s exception handling mechanism is truly robust and flexible, giving us the ability to write resilient and error-proof programs. Remember, the best way to understand it is through practice. So, get to it, and happy coding!
What Next?
Now that we have demystified the fundamentals of Python exception handling, the path of continuous learning is wide open. To further enhance your knowledge and skills in Python, we at Zenva recommend checking out our Python Mini-Degree.
The Python Mini-Degree we offer is a comprehensive collection of Python programming courses, designed especially for both beginners and the more experience learners.
Here’s what it covers:
- Coding basics
- Algorithms
- Object-oriented programming
- Game development
- App development
These courses offer flexible, 24/7 access, step-by-step projects like creating games and real-world apps. You get to reinforce your learning through coding challenges and quizzes, build a portfolio of Python projects and earn completion certificates. We take pride in mentioning that our courses have helped numerous learners secure jobs, start businesses, and publish their own games and websites.
We understand that many of you are past the basics and are looking for intermediate and advanced learning options. That’s why we encourage those of you interested in extending your Python mastery to explore our intermediate-level Python courses.
Conclusion
Python exception handling is a critical and powerful tool in ensuring your programs run smoothly, predictably, and reliably. Its mastery can add substantial value to your programming skills and your career as a Python developer.
At Zenva, we’re steadfast in our commitment to helping you on your coding journey. With the Python Mini-Degree, you receive the dedicated guidance and resources necessary to not only understand Python exception handling but to also delve deeper into creating games, apps, AI systems and more using Python. Let’s continue learning and growing together in this exciting world of coding!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
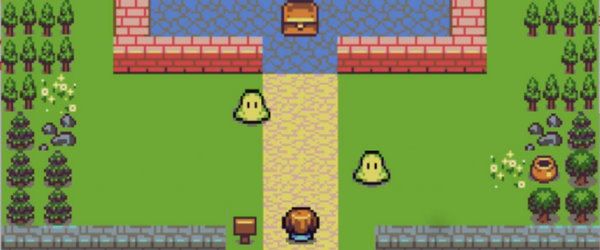
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.