Welcome to this exciting tutorial on Python Functional Programming. In the world of programming, Python is a language that offers flexibility and power, with its functional programming capabilities being a particularly strong feature. Whether you are an experienced coder looking to enhance your skills or you’ve just started your coding journey, this tutorial will prove informative and engaging.
Table of contents
What is Functional Programming?
Functional Programming is a coding paradigm focused on the evaluation of mathematical functions and avoids changing state and mutable data. It’s a style of programming that treats computation as the evaluation of mathematical functions and avoids side-effects and changing state. This fundamental concept can open up new possibilities in how you approach coding tasks.
Why should you learn Functional Programming in Python?
Learning Functional Programming in Python offers many benefits. Functional codes tend to be more concise, predictable, and easier to test than traditional imperative or procedural code. This methodology helps you to write better organized, more reusable, and more reliable code.
Now that we’ve explored the ‘What is’ and ‘Why should I learn it’ of Python Functional Programming, let’s move on to some code!
Functional Programming Basics in Python
Let’s delve into the basics of Functional Programming in Python. We will mainly focus on three fundamental concepts: purity, higher-order functions, and list comprehensions.
Purity
In Functional Programming, a function is considered pure if its output only depends on the input and it does not produce side-effects. Let’s have a look at a simple example:
def pure_function(x, y): return x + y print(pure_function(5, 7))
In this example, the function pure_function only returns the sum of the inputs. Its output is entirely determined by its input, so it’s pure. It does not change any global variables or affect anything outside of its scope.
Higher-Order Functions
A higher-order function either takes one or more functions as parameters or returns a function as an output, just as in the following example:
def higher_order(func, value): return func(value) def double(x): return x * 2 print(higher_order(double, 5)) # Output: 10
The function higher_order is taking another function as a parameter and a value to operate on. It then applies the function to the value.
List Comprehensions
Now, let’s check out how we can use list comprehensions to write more functional-style code.
numbers = [1, 2, 3, 4, 5] squares = [n**2 for n in numbers] print(squares) # Output: [1, 4, 9, 16, 25]
Here, we have created a new list, squares, from our original list of numbers using a list comprehension, which is a clear and concise way of creating new lists.
This part should give you a decent understanding of purity, higher-order functions, and list comprehensions in Python’s Functional Programming. Let’s move to the next part for further exploration.
More Functional Programming Concepts in Python
Building on what we’ve explored so far, we will now delve into other more complex concepts integral to Python’s Functional Programming: lambda functions, map, filter and functools.
Lambda Functions
Lambda functions are small, anonymous functions that are defined with the lambda keyword, instead of the def keyword.
double = lambda x: x * 2 print(double(5)) # Output: 10
In the example above, we’ve created a lambda function that doubles the input.
Map
The Python map function applies a specific function to every item of an iterable (like a list) and returns a map object (which is also an iterable).
numbers = [1, 2, 3, 4, 5] squared = map(lambda x: x**2, numbers) print(list(squared)) # Output: [1, 4, 9, 16, 25]
The map function, paired with a lambda, provided a quick way to perform an operation for each item in our list.
Filter
Like map, the filter function also applies a specific function to every item of an iterable, but it returns only those items for which the function evaluates to True.
numbers = [1, 2, 3, 4, 5] evens = filter(lambda x: x % 2 == 0, numbers) print(list(evens)) # Output: [2, 4]
The filter function, when paired with a lambda function, is a powerful tool that quickly filters items in a list.
Functools
The functools module in Python is used for higher-order functions, that is, functions that act on or return other functions.
from functools import reduce numbers = [1, 2, 3, 4, 5] product = reduce((lambda x, y: x * y), numbers) print(product) # Output: 120
In the example above, we’ve used reduce and a lambda function to find the product of all numbers in a list.
Through these examples, you should have gained an understanding of how to apply functional programming concepts in Python, making your code more efficient and concise.
Keep Learning with Zenva
So, you’ve taken the first steps in your Functional Programming journey with Python. That’s fantastic! But what’s next? Well, the world of Python is vast and full of opportunities for further exploration and development of your skills. For those who want to delve further into this exciting world, we invite you to check out our Python Mini-Degree.
The Python Mini-Degree offered by us at Zenva Academy is a comprehensive collection of courses that cover Python programming from different angles. Starting from coding basics, moving through algorithms and object-oriented programming, and ending with game development and app development using Python libraries and frameworks like Pygame, Tkinter, and Kivy. Not just for beginners, these courses also suit experienced learners, offering flexible learning options and project-based assignments that are sure to challenge and inspire you.
Remember, Python is ranked as the world’s most preferred programming language. The demand for Python skills spans multiple industries, making Python not just a fun language to learn but also a major asset in the job market.
Interested in exploring Python more broadly? We also offer a variety of other Python courses, taking learners from the fundamentals all the way to advanced topics.
Conclusion
In conclusion, Functional Programming in Python offers a dynamic, versatile and efficient way to deal with coding challenges. By mastering its concepts, you can write more efficient, organized, and reusable code. Python, with its flexibility and power, is an ideal choice for anyone interested in expanding their coding skill set.
We hope this tutorial has been helpful and inspiring for you. We encourage you to explore even deeper into the world of Python with our Python Mini-Degree. Remember, continued learning is key in the ever-evolving tech world, and with Zenva, you can keep pace with it. Happy coding!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
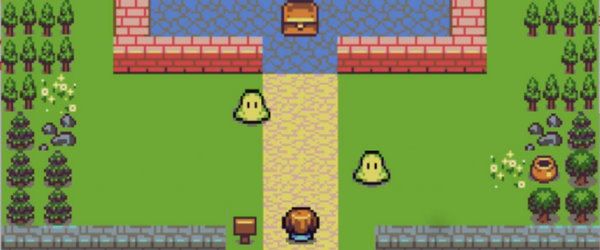
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.