Welcome to this comprehensive tutorial on Pygame keyboard press. Through practical examples of engaging game mechanics, we aim to make the concept of using keyboard inputs interactive and enjoyable while we uncover the broad capacities of the Pygame library.
Table of contents
What is Pygame?
Pygame is an open-source library designed to facilitate game creation in Python. It offers powerful features to manipulate graphics, sound, and user input which makes it an ideal choice for developing simple yet entertaining games.
Handling Keyboard Inputs in Pygame
One of the most fundamental concepts in Pygame is handling user inputs, especially from the keyboard. Keyboard input is crucial in most games as it allows the user to control game characters or actions.
Why Should I Learn Pygame Keyboard Press?
Understanding the Pygame keyboard press is essential to become proficient in game development with Pygame. Whether you’re building a simple arcade game or an ambitious platformer, having hands-on knowledge with keyboard inputs is vital.
Moreover, learning Pygame and particularly handling keyboard inputs, would strengthen your overall Python skills. Learning by doing tends to be the most effective and engaging approach, especially when it involves creating your own games!
Basic Setup for Pygame
Before we can handle keyboard inputs, we first need to setup a basic Pygame application. Here’s how you would create a basic window:
import pygame pygame.init() # Set size of the display window win_size = (800, 600) window = pygame.display.set_mode(win_size) # Game loop running = True while running: for event in pygame.event.get(): if event.type == pygame.QUIT: running = False pygame.quit()
Now we have a window for our game and a while loop to keep the program running until the user decides to close it.
Listening for Keyboard Input
Pygame uses events to handle inputs, and keyboard presses are no different. We can modify our game loop to listen for keyboard events as follows:
while running: for event in pygame.event.get(): if event.type == pygame.QUIT: running = False if event.type == pygame.KEYDOWN: if event.key == pygame.K_a: print('The A key was pressed.')
In the above example, Pygame will print a message when the user presses the ‘A’ key.
Handling Multiple Key Presses
Games usually require the user to press multiple keys simultaneously. Pygame events alone can’t handle this, but luckily Pygame provides a function that does.
keys = pygame.key.get_pressed() if keys[pygame.K_a]: print('The A key was pressed.') if keys[pygame.K_d]: print('The D key was pressed.')
This function returns a list of all keys that are currently being pressed down. This way, we can handle multiple key presses simultaneously.
Moving an Object with Keyboard Input
Now, let’s make our game a bit more visual. We’ll create a rectangle that moves when the user presses the arrow keys.
rectangle = pygame.Rect(50, 50, 50, 50) while running: for event in pygame.event.get(): if event.type == pygame.QUIT: running = False keys = pygame.key.get_pressed() if keys[pygame.K_LEFT]: rectangle.move_ip(-2, 0) if keys[pygame.K_RIGHT]: rectangle.move_ip(2, 0) if keys[pygame.K_UP]: rectangle.move_ip(0, -2) if keys[pygame.K_DOWN]: rectangle.move_ip(0, 2) window.fill((0, 0, 0)) pygame.draw.rect(window, (255, 255, 255), rectangle) pygame.display.flip()
In the example above, the rectangle will move left when the user presses the left arrow key, right when the right arrow key is pressed, and so on. With these examples, we’ve covered the basics of handling keyboard input, a key building block in game development using Pygame.
Adding Complexity with Pygame Key Press
As your Pygame skills evolve, you can make your games more complex. Let’s explore a few advanced ways to use keyboard inputs in Pygame.
Add Game Actions
In addition to controlling movements, keyboard inputs can be used to trigger game conditions or actions. Let’s setup our game so that pressing the spacebar will change the color of the rectangle:
color = (255, 255, 255) while running: for event in pygame.event.get(): if event.type == pygame.QUIT: running = False elif event.type == pygame.KEYDOWN: if event.key == pygame.K_SPACE: color = (255, 0, 0) keys = pygame.key.get_pressed() if keys[pygame.K_LEFT]: rectangle.move_ip(-2, 0) # .. other key press checks .. window.fill((0, 0, 0)) pygame.draw.rect(window, color, rectangle) pygame.display.flip()
When the user presses the spacebar, the color variable changes, therefore changing the color of the rectangle when it is drawn.
Character Animation
You can animate game elements using keyboard inputs. Consider a sprite sheet with various frames of a character walking. We can loop through these frames whenever the character moves to create an animation.
# Suppose character is a <a href="https://gamedevacademy.org/pygame-surface-tutorial-complete-guide/" title="Pygame Surface Tutorial – Complete Guide" target="_blank" rel="noopener">pygame surface</a> containing frames of a character walking character_frame = 0 while running: # .. event loop .. keys = pygame.key.get_pressed() if keys[pygame.K_LEFT]: rectangle.move_ip(-2, 0) character_frame += 1 # .. other key press checks .. window.fill((0, 0, 0)) pygame.draw.rect(window, (255, 255, 255), rectangle) window.blit(character, (50, 50), (character_frame % 4 * 50, 0, 50, 50)) pygame.display.flip()
We’re using the modulus operator to cycle back to the first frame after the last one. This creates an infinite looping animation.
Continuous & Discrete Actions
While some actions in a game can be continuous (e.g., movement), others need to be discrete (e.g., firing a weapon). To differentiate between continuous and discrete actions, we can use both methods of handling keyboard inputs.
while running: for event in pygame.event.get(): if event.type == pygame.QUIT: running = False elif event.type == pygame.KEYDOWN: if event.key == pygame.K_SPACE: print('BOOM! Fire in the hole!') keys = pygame.key.get_pressed() if keys[pygame.K_LEFT]: rectangle.move_ip(-2, 0) # .. other key press checks .. window.fill((0, 0, 0)) pygame.draw.rect(window, (255, 255, 255), rectangle) pygame.display.flip()
Here, the continuous action is the movement of the rectangle (LEFT key), while the discrete action is firing a weapon (SPACE key). The event loop method ensures the discrete action only occurs once per key press.
These examples show how combining keyboard inputs with other functions of the Pygame library can help create interactive and complex games. As with any coding project, the more you practice and experiment, the more you will learn and improve!
Adding Actions based on key hold duration
There could be situations in your game where you would want to perform an action based on the duration a key is held. For example, in a jump-n-run game, you might want the character to jump higher if the jump key is held longer. You can achieve this by storing the time when a key was pressed and calculate the hold duration in the KEYUP event.
key_down_time = 0 while running: for event in pygame.event.get(): if event.type == pygame.QUIT: running = False elif event.type == pygame.KEYDOWN: if event.key == pygame.K_SPACE: key_down_time = pygame.time.get_ticks() elif event.type == pygame.KEYUP: if event.key == pygame.K_SPACE: key_up_time = pygame.time.get_ticks() hold_duration = key_up_time - key_down_time jump_height = calculate_jump_height_based_on_hold_duration(hold_duration) character.jump(jump_height)
In this example, whenever the player presses the SPACE key, we store the current time in ‘key_down_time’. At the moment when the key is released, we calculate the hold duration and use it to calculate the jump height. Finally, we make the character jump using a hypothetical function.
Text Input
Sometimes you need the player to input text, perhaps to enter their name for a highscore list. Pygame doesn’t offer an inbuilt function for this, but you can build one using keyboard events.
text = '' while running: for event in pygame.event.get(): if event.type == pygame.QUIT: running = False elif event.type == pygame.KEYDOWN: if event.key == pygame.K_BACKSPACE: text = text[:-1] else: text += event.unicode window.fill((0, 0, 0)) font = pygame.font.Font(None, 32) text_surface = font.render(text, True, (255, 255, 255)) window.blit(text_surface, (50, 50)) pygame.display.flip()
In the above code, the user’s input is stored in a string called ‘text’. When the user presses a key, the corresponding character is added to the string. If the key is the backspace key, we remove the last character from the string. This is useful for implementing a name input feature in your game. The entered text will also be displayed on the screen.
Combining Mouse and Keyboard Input
In many games, you would want to combine mouse and keyboard inputs. Pygame allows you to handle mouse events and keyboard events separately. Here is an example of how you can move a player sprite towards the mouse position using WASD for movement:
player_rect = pygame.Rect(50, 50, 50, 50) while running: for event in pygame.event.get(): if event.type == pygame.QUIT: running = False keys = pygame.key.get_pressed() if keys[pygame.K_w]: player_rect.move_ip(0, -2) # .. other key press checks .. mouse_pos = pygame.mouse.get_pos() window.fill((0, 0, 0)) pygame.draw.rect(window, (255, 255, 255), player_rect) if pygame.mouse.get_pressed()[0]: target_pos = pygame.mouse.get_pos() direction = (target_pos[0] - player_rect.center[0], target_pos[1] - player_rect.center[1]) player_rect.move_ip(direction) pygame.display.flip()
In the above code, we first handle keyboard inputs to move the rectangle. We then check if the left mouse button is pressed. If it is, we move the rectangle towards the mouse pointer. Note that you could use the same approach to handle touch inputs if you’re developing a game for a touchscreen device.
Now that you’ve got a grasp on handling keyboard inputs in Pygame, where do you go from here? The answer is simple – keep experimenting, creating, and learning. Game development with Pygame isn’t limited to just moving rectangles around a screen. You can create complex systems, interesting mechanics, and thrilling games that provide endless hours of entertainment. With perseverance and practice, the only limit is your imagination.
We recommend continuing your educational journey by checking out our Python Mini-Degree. This comprehensive selection of courses covers a broad range of Python programming topics, from coding basics to app development. The curriculum includes engaging projects like constructing arcade games, fabricating a medical diagnosis bot, and building an escape room. Regardless if you’re a beginner or an experienced programmer, the Python Mini-Degree offers something for everyone. With versatile and practical content updated regularly, it’s a valuable tool to enhance your Python and Pygame abilities.
Additionally, you might want to explore our wider range of Python courses. At Zenva, we offer over 250 courses from beginner to professional level in programming, game development, and AI. Whether your aspirations lie in creating enjoyable games or mastering the art of coding, Zenva will help you achieve your ambitions.
Conclusion
Mastering keyboard inputs is a fundamental step in your Pygame journey, and an essential skill in rendering your game interactive and enjoyable. By getting a firm grip on this aspect, you’re laying strong foundations for your game development path.
We encourage you to keep exploring with our Python Mini-Degree or any of our comprehensive Python courses. With each line of code you write and each project you complete, you’re one step closer to becoming a proficient game developer! Let’s create, learn, and most importantly – have fun on this fascinating journey.
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
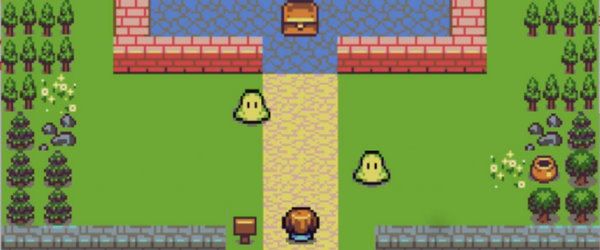
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.