In the ever-expanding universe of Python, the pygame library offers a fun, robust way to create video games and multimedia applications. It’s a remarkable toolkit, particularly attractive for those looking to delve into game development. A significant aspect of pygame, and the focus of this article, is using the mouse module for game functionality.
Table of contents
What is Pygame’s Mouse Module?
The mouse module in pygame allows us to interact with the mouse input in a game setting – it’s our bridge between the player and the game itself. Through this module, we can detect mouse’s position, its button clicks, and even hide or show the mouse altogether.
What is Pygame’s Mouse Module Used For?
Pygame’s mouse module is predominantly used for integrating mouse-driven interactions in a game or multimedia application. This can involve moving game characters, choosing options, drag-and-drop functionality, and so on. It breathes life into static elements, allowing users to interact with them in a real, tangible way.
Why Should You Learn the Pygame’s Mouse Module?
Understanding the workings of pygame’s mouse module is significant for a number of reasons:
- This module is a fundamental part of creating interactive and user-friendly games and apps in pygame. Thus, learning it boosts your game development skills.
- The concepts learned here can be applied to other graphical interfaces and game development platforms.
- It opens up room for more complexity in your programs, providing opportunities for unique mechanics and increased user engagement.
In this tutorial, we will delve into the mouse module, learn how to catch mouse events, and explore how to utilize these inputs in a game scenario. We are going to have an engaging experience creating simple yet fun game mechanics using the mouse module. So buckle up, and let’s jump right into it!
Getting the Mouse Position
First, let’s understand how to get the current position of the mouse.
import pygame # Initialize pygame pygame.init() screen = pygame.display.set_mode((500,500)) running = True while running: for event in pygame.event.get(): if event.type == pygame.QUIT: running = False # Get the mouse position mouse_pos = pygame.mouse.get_pos() print(mouse_pos) pygame.quit()
In the code above, pygame.mouse.get_pos() is used to get the current mouse position, which is printed out in the console.
Detecting Mouse Clicks
Next, we’ll learn how to detect mouse clicks using the pygame library.
import pygame # Initialize pygame pygame.init() screen = pygame.display.set_mode((500,500)) running = True while running: for event in pygame.event.get(): if event.type == pygame.QUIT: running = False elif event.type == pygame.MOUSEBUTTONDOWN: print('Mouse Button Pressed!') pygame.quit()
In the above snippet, we use the pygame.MOUSEBUTTONDOWN event to detect whenever a mouse button is clicked. Here, we simply print a message, but you could potentially develop this functionality to trigger some action in your game.
Handling Different Mouse Buttons
In pygame, each mouse button (left, right, middle) is considered separately.
import pygame # Initialize pygame pygame.init() screen = pygame.display.set_mode((500,500)) running = True while running: for event in pygame.event.get(): if event.type == pygame.QUIT: running = False elif event.type == pygame.MOUSEBUTTONDOWN: if event.button == 1: print('Left Mouse Button Pressed!') elif event.button == 2: print('Middle Mouse Button Pressed!') elif event.button == 3: print('Right Mouse Button Pressed!') pygame.quit()
In the above code, the number attached to the ‘button’ attribute of the event object denotes which mouse button was pressed: 1 for left, 2 for middle and 3 for right.
Showing and Hiding the Mouse Cursor
Lastly, in certain scenarios, you might want to hide the mouse cursor to improve the game’s aesthetics or functionality. Here’s how to do that:
import pygame # Initialize pygame pygame.init() screen = pygame.display.set_mode((500,500)) pygame.mouse.set_visible(False) # to hide the mouse running = True while running: for event in pygame.event.get(): if event.type == pygame.QUIT: pygame.mouse.set_visible(True) # show the mouse before quitting running = False pygame.quit()
Here, we utilize the pygame’s set_visible() method. When set_visible(False) is called, the mouse cursor gets hidden, and set_visible(True) brings it back when the game quits.
Changing the Mouse Cursor
Apart from hiding and showing, pygame also lets us change the appearance of the mouse cursor. We can change the mouse cursor to different system cursors:
import pygame # Initialize pygame pygame.init() screen = pygame.display.set_mode((500, 500)) # Change the cursor to a hand icon pygame.mouse.set_cursor(*pygame.cursors.broken_x) running=True while running: for event in pygame.event.get(): if event.type == pygame.QUIT: running = False pygame.quit()
In the above code, pygame.mouse.set_cursor() function changes the cursor to a given system cursor. Pygame offers built-in system cursors like ‘broken_x’, ‘diamond’, ‘tri_left’, etc.
Drag and Drop Functionality
Pygame’s mouse module can also be used to implement drag-and-drop functionality, a common feature in many games and software. Here’s a basic example:
import pygame # Initialize pygame pygame.init() screen = pygame.display.set_mode((500,500)) rect = pygame.Rect(50, 50, 60, 60) # rectangle that we will move dragging = False # track whether we are dragging the rectangle running = True while running: for event in pygame.event.get(): if event.type == pygame.QUIT: running = False elif event.type == pygame.MOUSEBUTTONDOWN: if event.button == 1: # Left mouse button if rect.collidepoint(event.pos): # Check if clicked on the rectangle dragging = True elif event.type == pygame.MOUSEBUTTONUP: if event.button == 1: # Left mouse button dragging = False elif event.type == pygame.MOUSEMOTION: if dragging: mouse_x, mouse_y = event.pos rect.center = (mouse_x, mouse_y) screen.fill((0,0,0)) pygame.draw.rect(screen, (255,0,0), rect) # Draw our rectangle pygame.display.flip() pygame.quit()
This snippet creates a simple drag-and-drop functionality where we can click and drag a red rectangle around with the mouse.
The pygame’s mouse module is an incredible tool in game development. Understanding its functionality allows you to enhance your game’s interactivity and engagement level. Be creative and experiment with the skills and codes you’ve learned here. Happy coding!
Customizing Mouse Cursors
You can create custom mouse cursors by passing a bitmap string or a list with integers to the cursor setting function. Here’s an example of setting a larger than usual arrow cursor:
import pygame # Initialize pygame pygame.init() screen = pygame.display.set_mode((500,500)) # Create custom cursor cursor = ( " ", " ", " .. ", " .... ", " ...... ", " ......... ", " .......... ", " ........... ", " ...... #### ", " ....... ### ", " .. #### ### ", " ### ### ", " ## ### ", " ### ### ", " ", " ", " ") pygame.mouse.set_cursor(*pygame.cursors.compile(cursor)) running = True while running: for event in pygame.event.get(): if event.type == pygame.QUIT: running = False pygame.quit()
In this code, we first create a tuple cursor that defines the image of the cursor in plain text characters. The dot . represents the transparent area, and the hash # represents the opaque area. Then we use pygame.cursors.compile(cursor) function to compile the custom cursor.
Using Mouse to Draw on Screen
Let’s create an application where you can draw on the screen using the mouse.
import pygame # Initialize pygame pygame.init() screen = pygame.display.set_mode((500,500)) drawing = False color = (255,255,255) running = True while running: for event in pygame.event.get(): if event.type == pygame.QUIT: running = False elif event.type == pygame.MOUSEBUTTONDOWN: drawing = True elif event.type == pygame.MOUSEBUTTONUP: drawing = False elif event.type == pygame.MOUSEMOTION: if drawing: pygame.draw.circle(screen, color, event.pos, 5) pygame.display.flip() pygame.quit()
In the above code, we set a boolean drawing to True when the mouse button is down and false when it’s up. If the mouse is moving and drawing is True, we draw a small circle at the location of the mouse.
Tracking Mouse Movements
Finally, let’s consider a scenario where we need to follow real-time mouse movement.
import pygame # Initialize pygame pygame.init() screen = pygame.display.set_mode((500,500)) running = True while running: for event in pygame.event.get(): if event.type == pygame.QUIT: running = False elif event.type == pygame.MOUSEMOTION: x,y = event.pos print(f"Mouse at ({x}, {y})") pygame.quit()
In the above snippet, we use the pygame.MOUSEMOTION event to track the movement of the mouse. We print the current position of the mouse whenever it moves.
Understanding the mouse module in pygame and its use cases is one more milestone in your game development journey. The ability to detect and respond to mouse clicks, get the mouse position, perform mouse-related actions, and more, can help you create more interactive and engaging games. Keep learning and coding!Congratulations on making it this far! Your journey into the world of Python game development using pygame is only just beginning. There is much more to explore, such as more sophisticated game mechanics, artificial intelligence, and even 3D games! Remember, practice and application are the best ways to reinforce and advance your skills.
If you’re wondering where to go next, we’d like to recommend Zenva’s Python Mini-Degree. It’s a comprehensive collection of Python courses that covers everything from the basics of coding to Algorithms, Object-Oriented Programming, and even game and app development. The courses are flexible, allowing you to learn at your own pace, and finish with a portfolio of your own Python projects and a completion certificate.
For a wider range of Python courses, feel free to check out our Python courses page. At Zenva, we provide courses that cater to every skill level – whether you’re a beginner or a seasoned programmer looking to sharpen your skills. We offer engaging learning experiences with over 250 supported courses spanning programming, game development, and AI. Keep exploring, keep coding, and most importantly, keep making games! Happy learning!
Conclusion
Congratulations on taking this essential step in your Python game development journey! As you continue to discoveries with pygame and its incredibly flexible mouse module, remember that every new module and function you master adds another tool to your ever-expanding toolkit. In the world of game development, this spells greater creativity, increased interactivity, and higher user engagement.
Whether you are a newcomer or a seasoned developer, our Python courses at Zenva cater to all skill levels with engaging, flexible, and tailor-made learning experiences. We invite you to explore further, keep practicing, and continue innovating. After all, every great game began with a single line of code – the possibilities are truly endless. Happy coding!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
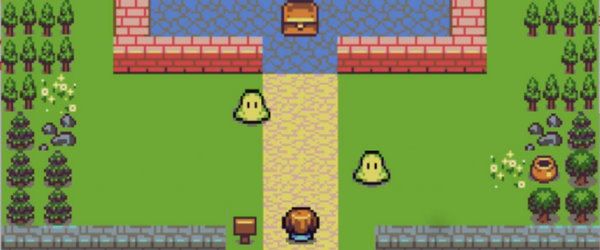
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.