Welcome to our comprehensive guide on Pygame Timer! This wonderful Python library tool contains many features, one of which is the timer functionality. The timer helps add an extra dimension of interaction and engagement to your Python projects. Whether you are just starting your coding journey or a seasoned professional, this tutorial will help you understand and implement Pygame Timer with ease.
Table of contents
What is Pygame Timer?
Pygame Timer is a simple yet effective feature within the larger Pygame library in Python. Simply put, it allows you to introduce a timing mechanism into your projects, running certain code blocks after a specified amount of time or at regular intervals.
What is it For?
Imagine you are developing a game where a certain event needs to happen every 5 seconds, or you want to track the elapsed time since the game started, or maybe you need to add a countdown mechanism. That’s where Pygame Timer comes into play! It provides a reliable and easy-to-implement way to add these time-related functionalities into your projects.
Why Should I Learn It?
Adding a timer to gameplay can significantly enhance the overall gaming experience. It introduces new levels of challenge and engagement for players. As a developer, understanding how to implement these timing mechanisms in Pygame will enable you to create more dynamic and interactive gaming experiences. Plus, it’s a valuable skill set to add to your coding knowledge as it’s not only useful in game development but also in other time-critical applications. Set your coding journey on fire with this fantastic tool today!
Getting Started with Pygame Timer
Before we get into coding, make sure you have the Pygame library installed in your Python environment. If not, you can install it using pip in your terminal:
pip install pygame
Now, let’s dig into it!
Creating a Simple Pygame Window
In Pygame, you begin with a simple window, which is the playground for all your animations, interactions, and timings. Let’s write the basic code to set up a window:
import pygame pygame.init() win = pygame.display.set_mode((500, 500)) while True: pygame.time.delay(100) # 100ms delay for event in pygame.event.get(): if event.type == pygame.QUIT: pygame.quit()
Understanding Pygame Events and the QUIT Event
In the above code, `pygame.event.get()` returns a list of all the events that occured since it was last called. The `QUIT` event is triggered when you click the close button on your Pygame window.
Pygame Clock and Time Delay
Notice `pygame.time.delay(100)`. This statement causes a delay of 100 milliseconds in our program execution. It’s a simple way to make sure our code doesn’t run too quickly.
Setting Up a Timer Event
Now, let’s get to the interesting part: the Timer event. For this, we first need to create a new event type (since Pygame doesn’t provide a built-in timer event). This can be done as follows:
TIMEREVENT = pygame.USEREVENT + 1 pygame.time.set_timer(TIMEREVENT, 1000) # 1 second = 1000 milliseconds
In the above piece of code, `pygame.USEREVENT` returns the first available user event id and we’ve added 1 to it to create a unique event id for our Timer event. The command `pygame.time.set_timer(TIMEREVENT, 1000)` sets up a timer event that will be added to the event queue every 1000 milliseconds.
Working with the Timer Event
From here, we can incorporate this new timer event into our main game loop. This way, we can specify what happens every time the timer event is fired. Let’s add a bit of code to display a message to the console every time the timer event occurs:
while True: pygame.time.delay(100) for event in pygame.event.get(): if event.type == pygame.QUIT: pygame.quit() elif event.type == TIMEREVENT: print('Timer Event Fired!')
Whenever the timer event (which we’ve set to fire every second) fires, “Timer Event Fired!” is printed on the console.
Applying Timer Event in Game Development
A practical game-related application of a timer would be for a character to perform an action at regular intervals. For example, let’s assume we want to change the color of our game window every second. We can do this by using our TIMEREVENT:
currentColor = 0 colors = [(255, 0, 0), (0, 255, 0), (0, 0, 255)] # RGB color values for red, green, blue while True: pygame.time.delay(100) for event in pygame.event.get(): if event.type == pygame.QUIT: pygame.quit() elif event.type == TIMEREVENT: currentColor = (currentColor + 1) % 3 # Cycle through the three colors win.fill(colors[currentColor]) pygame.display.update()
In this code, we’re changing the color of our game window between red, green, and blue every second.
Creating a Countdown Timer
It is also possible to use a timer to create a countdown. Let’s try to create a 10-second countdown timer that updates the window title every second:
countdown = 10 while True: pygame.time.delay(100) for event in pygame.event.get(): if event.type == pygame.QUIT: pygame.quit() elif event.type == TIMEREVENT: countdown -= 1 pygame.display.set_caption(f'Countdown: {countdown}') if countdown == 0: print('Time\'s up!') pygame.quit()
The window title will now count down from 10 to 0, at which point “Time’s up!” is printed to the console, and the Pygame window is closed.
These examples just scratch the surface of what you can accomplish with Pygame Timer. Its applications are as broad as your creativity!
Animating a Game Character
Let’s dive deeper and animate a game character using the Pygame timer. Assume that your character has different sprites for walking, and you have loaded them into the `sprites` list. Here’s how you can animate the character:
currentSprite = 0 sprites = [...] # List of character sprites while True: pygame.time.delay(100) for event in pygame.event.get(): if event.type == pygame.QUIT: pygame.quit() elif event.type == TIMEREVENT: currentSprite = (currentSprite + 1) % len(sprites) # Cycle through the sprites # Draw the current sprite to the window win.blit(sprites[currentSprite], (0,0)) pygame.display.update()
In this example, we use the timer to switch through different characters’ sprites, thus creating an animation effect.
Creating a Speed-Up Mechanism
In some games, you might want certain events or actions to speed up over time – for example, making a block fall faster and faster in a Tetris-like game. You can accomplish this with the pygame timer:
counter = 0 TIMEREVENTSPEEDUP = pygame.USEREVENT + 2 pygame.time.set_timer(TIMEREVENTSPEEDUP, 5000) # Speed up every 5 seconds fallSpeed = 500 # Initial fall speed TIMEREVENTFALL = pygame.USEREVENT + 3 pygame.time.set_timer(TIMEREVENTFALL, fallSpeed) while True: pygame.time.delay(100) for event in pygame.event.get(): if event.type == pygame.QUIT: pygame.quit() elif event.type == TIMEREVENTSPEEDUP: fallSpeed = max(fallSpeed // 2, 50) # Don't let it get too fast! pygame.time.set_timer(TIMEREVENTFALL, fallSpeed) # Reset the fall timer elif event.type == TIMEREVENTFALL: # Code to make the block fall
In this code, the block initially falls once every 500 milliseconds. But every 5 seconds (controlled by the TIMEREVENTSPEEDUP event), the time between falls is cut in half, up to a limit where it won’t fall more than once every 50 milliseconds.
Managing Multiple Timers
This simple example demonstrates that multiple timer events can coexist without any conflict. They run independently and trigger their events after the specified time, offering the ability to control different events or game mechanics independently.
Pygame Timer gives you control over how you manage time-related game mechanics. Whether it’s a countdown, timed events, or synchronization of activities, Pygame Timer is your go-to tool. Plus, as part of a rich library like Pygame, it has excellent compatibility with other game programming resources. Indeed, Pygame Timer sets the pulse for interesting game dynamics and should be a part of every game developer’s toolkit.
We at Zenva believe in sharing knowledge that helps you bring your ideas to life. We hope this tutorial has given you valuable insight into the effective use of Pygame Timer. Now it’s time for you to start implementing these concepts into your own game projects! Happy coding!
Where to Go Next?
Having explored the intricacies of Pygame Timer, an essential tool in Python game development, you might be wondering, “Where do I go from here?” It’s time to explore more diverse and powerful aspects of Python and game development.
If you’re looking for a comprehensive learning program, we encourage you to check out our Python Mini-Degree. This pathway covers not only the fundamentals of Python programming but also guides you deeper into the realm of game development, algorithms, object-oriented programming, and app development. As you work through step-by-step projects, you will build a portfolio of Python applications and gain valuable skills that employers are actively seeking. It’s not just about learning; it’s about applying that knowledge in a practical way.
For more variety and personalized learning opportunities, explore our collection of Python courses where you can pick the ones that suit your interests and learning goals the best. Remember, learning is a continuous journey, and every step you take gets you closer to your dreams. With Zenva, you’re not just learning how to code; you’re preparing for a promising career in the world of technology. Happy exploring!
Conclusion
In this tutorial, we’ve illuminated the workings of Pygame Timer, bringing you one step closer to mastering game development in Python. This versatile tool, when used effectively, can immensely elevate your game projects, making them more dynamic and interactive. With the newly acquired knowledge, it’s time to unfold your creativity and inject new life into your games.
We hope you continue this thrilling journey of learning with us. Take a step further and dive deeper into Python game development with our Python Mini-Degree. Equip yourself with a wide array of useful Python skills and set the path for a promising future in the tech world. At Zenva, we are thrilled to be your guide on your coding odyssey. So, let’s get coding!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
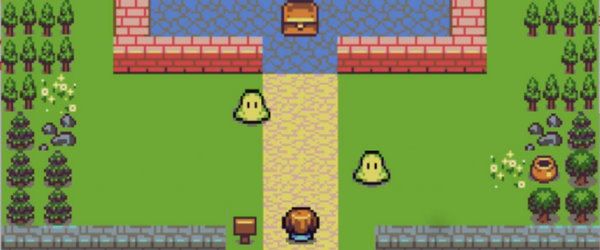
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.