We’re venturing today into an exciting realm of game development – “Roblox vehicle scripting”. This article demystifies this fascinating topic with a hands-on approach, engaging examples, and simple analogies. Whether you’re a beginner just entering the coding world, or an experienced programmer looking to learn something new, this tutorial will serve you well. Buckle up and get ready to fuel your coding skills!
Table of contents
Understanding Roblox Vehicle Scripting
At its core, Roblox vehicle scripting is all about coding vehicles in games on Roblox. It’s what gives life to cars, planes, boats, or even imaginary vehicles in Roblox games.
The Power of Roblox Vehicle Scripting
Harnessing the power of vehicle scripting enhances your game’s realism and engagement. Imagine creating a racing game with realistic vehicle controls, or an adventure game with flying cars – all possible with Roblox vehicle scripting.
Why Learn Roblox Vehicle Scripting?
Roblox vehicle scripting is a sought-after skill in the Roblox developer community. It opens up a plethora of opportunities, from making your games more enjoyable to potentially earning Robux, Roblox’s in-game currency. Plus, it’s a fun and creative way to improve your coding skills.
It’s now time to shift gear and roll into the exciting world of coding vehicles in Roblox. Ready? Let’s ride!
Creating a Basic Vehicle
The first step to scripting a vehicle in Roblox is to create the vehicle model itself. Using Part object in Roblox, we can create a simple vehicle:
local vehicle = Instance.new("Part") vehicle.Name = "MyFirstVehicle" vehicle.Size = Vector3.new(1,1,3) vehicle.BrickColor = BrickColor.new("Bright red") vehicle.Parent = workspace
In the code above, a Part is created, given the name ‘MyFirstVehicle’, a size, a color, and finally added to the workspace.
To give the vehicle a simple movement functionality:
local vehicle = Instance.new("VehicleSeat") vehicle.Name = "MyFirstVehicle" vehicle.Size = Vector3.new(1,1,3) vehicle.BrickColor = BrickColor.new("Bright red") vehicle.Parent = workspace
Here, this time we created a ‘VehicleSeat’ instead of a ‘Part’. This ‘VehicleSeat’ part has built-in vehicle mechanics.
Adding Wheels
A vehicle wouldn’t be much of a vehicle without wheels. We can create wheels for our vehicle using a similar method:
local wheel = Instance.new("Part") wheel.Name = "Wheel" wheel.Shape = "Cylinder" wheel.Size = Vector3.new(0.8,-1,0.8) wheel.BrickColor = BrickColor.new("Medium stone gray") wheel.Parent = workspace
The ‘Shape’ property will ensure that the part created is cylindrical, resembling a wheel.
Let’s attach this wheel to our vehicle. For this, we’ll need a HingeConstraint:
local hinge = Instance.new("HingeConstraint") hinge.Attachment0 = vehicle:FindFirstChild("Attachment") hinge.Attachment1 = wheel:FindFirstChild("Attachment") hinge.Parent = workspace
A HingeConstraint connects two parts and allows them to rotate about a common axis. ‘Attachment0’ and ‘Attachment1’ are the two parts we wish to connect – in this case, our vehicle and wheel.
Making the Vehicle Move
To make a vehicle move, we can use the ‘Throttle’ property of the VehicleSeat part:
vehicle.Throttle = 1
Setting the ‘Throttle’ property to 1 would make the vehicle move forward. You can set ‘Throttle’ to -1 to reverse the vehicle direction.
Stay tuned for the next part where we’ll explore turning mechanisms and car customization!
Steering Mechanism
Providing steering capabilities to your vehicle makes it more interactive and fun to use. For our basic car, we can leverage the `Steer` property of the VehicleSeat part:
vehicle.Steer = 1
Setting ‘Steer’ to 1 makes the vehicle steer right, -1 for left and 0 to make it go straight.
Adding More Wheels
To balance our vehicle and make it more practical, let’s add more wheels. Like we did earlier, create three more wheel parts and attach them to the vehicle:
-- Create more wheels local wheel2 = wheel:Clone() wheel2.Parent = workspace local wheel3 = wheel:Clone() wheel3.Parent = workspace local wheel4 = wheel:Clone() wheel4.Parent = workspace -- Add HingeConstraints local hinge2 = hinge:Clone() hinge2.Attachment1 = wheel2:FindFirstChild("Attachment") hinge2.Parent = workspace local hinge3 = hinge:Clone() hinge3.Attachment1 = wheel3:FindFirstChild("Attachment") hinge3.Parent = workspace local hinge4 = hinge:Clone() hinge4.Attachment1 = wheel4:FindFirstChild("Attachment") hinge4.Parent = workspace
In this code, we’ve cloned our original wheel three times and created corresponding HingeConstraints for the cloned wheels.
Customizing Your Vehicle
Roblox scripting isn’t just about creating functional objects – customization is a big part of the fun! Let’s look at how to customize your vehicle:
– Changing the color: We can change the color of our vehicle by simply modifying the BrickColor property:
vehicle.BrickColor = BrickColor.new("Bright yellow")
– Changing the size: We can change the size of our vehicle using the Size property:
vehicle.Size = Vector3.new(2,1,6) -- makes the vehicle twice as long
– Adding decals: We can add images or designs (decals) on our vehicle for further customization:
local decal = Instance.new("Decal") decal.Texture = "http://www.roblox.com/asset/?id=2532201" -- substitute with any texture URL decal.Face = Enum.NormalId.Top decal.Parent = vehicle
These are just the basics. Once you understand these fundamentals, you can create a variety of unique vehicles in your Roblox games. Dive in and start experimenting!
Creating a User Interface for Controls
Now that we’ve learned how to make our vehicle move, let’s look at how we can control our vehicle through a user interface.
The first step in creating any User Interface (UI) in Roblox is to create a ScreenGui. ScreenGui acts as a container for other GUI objects.
local screenGui = Instance.new("ScreenGui") screenGui.Parent = game.Players.LocalPlayer:WaitForChild("PlayerGui")
In the code above, we’ve created a ScreenGui and parented it to the PlayerGui, allowing it to be visible on the player’s screen.
Next, let’s create two buttons for controlling the vehicle. Each button will be a TextButton object.
local button1 = Instance.new("TextButton") button1.Text = "Move Forward" button1.Size = UDim2.new(0.2, 0, 0.1, 0) button1.Position = UDim2.new(0.4, 0, 0.8, 0) button1.Parent = screenGui local button2 = button1:Clone() button2.Text = "Move Backward" button2.Position = UDim2.new(0.6, 0, 0.8, 0) button2.Parent = screenGui
The code sets the Text property to “Move Forward” and “Move Backward”. It also sets the Size and Position properties to position the buttons on the screen, and parented them to the ScreenGui.
The next step is crucial – we need to make these buttons work. We can do this by creating a function for each button that changes the VehicleSeat’s Throttle property.
button1.MouseButton1Click:Connect(function() vehicle.Throttle = 1 end) button2.MouseButton1Click:Connect(function() vehicle.Throttle = -1 end)
In the above code, we’ve connected the MouseButton1Click event of each button to a function. When the button is clicked, the function changes the Throttle of the VehicleSeat, causing it to move forward or backward.
Making a vehicle stop when the button is released requires another function to be bound to MouseButton1Up event of each button.
button1.MouseButton1Up:Connect(function() vehicle.Throttle = 0 end) button2.MouseButton1Up:Connect(function() vehicle.Throttle = 0 end)
With these final pieces of code, you now have a working UI that can control a vehicle! Go on and drive your freshly coded vehicle around your Roblox world. Remember that all these functionalities are customizable, so feel free to experiment and make your vehicle truly unique!
Matching Your Aspirations
At Zenva, we believe in nurturing coding creativity, game development prowess and learning new technologies. We hope this tutorial has given you a strong starting point for your Roblox vehicle scripting journey. Don’t stop here; keep learning, keep building, and continue wielding the power of code. Stay creative, stay curious, and happy coding!
Accelerating Your Learning Journey
If you’ve found your journey into Roblox vehicle scripting thrilling and wish to further amplify your learning experience, we at Zenva offer numerous routes to march ahead. Our wide-ranging programming, game development, and AI courses, for beginners and professionals alike, strive to accelerate your skill growth, creating a path to improve your career prospects, or simply boost your confidence and satisfaction in personal projects.
A notable next-step for any Roblox enthusiasts is our trump card – the Roblox Game Development Mini-Degree. This comprehensive course bundle includes a gamut of genres, teaching you various aspects of game creation using Roblox Studio and Lua. It’s perfect for individuals at any skill level – whether you’re making your first foray into coding or a seasoned programmer looking for new challenges. Taught by experienced gamers and certified coders, this Mini-Degree covers exploration of Roblox Studio foundations, 3D level development, platforming mechanics, multiplayer combat, leaderboards implementation, and more.
For a broader collection of Roblox related courses, feel free to explore our Roblox courses. At Zenva, we firmly believe that continual learning is the key to growth. Once you embark on this journey, you’ll discover that every new line of code, every small mistake corrected, and every creative solution to a problem, all adds up to develop you into a better coder, and ultimately, a problem solver. We invite you to continue your journey with us and step into the exciting world of game development.
Conclusion
There you have it, a fun-filled, comprehensive journey into the world of Roblox vehicle scripting. We trust you found the tutorial enlightening and enjoyed shaping your own virtual ride. Remember, learning new skills should be an enjoyable journey and not a race. Keep coding, keep exploring, and keep fuelling your passion.
At Zenva, we’re here to facilitate and nurture your coding journey, from the first baby steps to the mastering leaps. Whether you wish to deepen your understanding of Roblox, advance towards another programming language, experiment with artificial intelligence, or delve deeper into game development, our courses cater to a wide spectrum of interests. Each tutorial, each course, and each Mini-Degree is a stepping stone towards achieving your coding dreams. With Zenva, you’re both the driver and the creator of your vehicle. Drive on!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
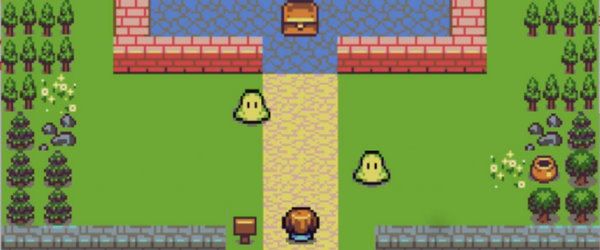
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.