Welcome learners, to this comprehensive tutorial on the global keyword in Python! Understanding ‘global’ is fundamental to mastering Python, so we’re really excited that you’ve chosen to take this journey with us.
Table of contents
What exactly is ‘global’ in Python?
Python ‘global’ is a keyword used inside a function to declare that a variable is a global variable. Anything you do to the variable that you declared as global, you’re doing to the global variable.
‘Global’ is most commonly used when we want to perform operations on a variable in a function especially when the same variable is also defined in the global scope.
If you aim to enhance your Python skills, then learning about ‘global’ will make your coding journey far smoother. It will grant you more control and flexibility over your Python script. Whether you’re programming a simple game or a sophisticated algorithm, ‘global’ can help you navigate the intricacies of functions, synchronizing gameplay, and so much more!
It’s not just about functionality, but also about cleaner code. Using ‘global’ wisely can contribute to making your code more readable and maintainable.
Defining the Basics: Examples of Global Keyword
To help you understand the ‘global’ keyword better, let’s take a look at some practical examples:
Example 1: Global Variable in Function
Let’s start by declaring a simple global variable. After that, we’ll define a function that will update the value of this variable.
x = 10 def update(): global x x = 20 print(x) update() print(x)
Now the output will be:
10 20
This happens because we’ve declared ‘x’ as a global variable using the ‘global’ keyword inside the update() function.
Example 2: Multiple Global Declarations
You may encounter instances where you will have to use more than one global variable. This is how to handle this:
x = 10 y = 30 def update(): global x, y x = 20 y = 40 print(x, y) update() print(x, y)
And here’s the output:
10 30 20 40
We can declare multiple variables as global by separating them with commas.
Advanced Examples of Global Keyword
Example 3: Global Variables in Nested Functions
Now, let’s check how to use the same global variables in the case of nested functions:
x = 10 def outer(): global x x = 20 def inner(): global x x = 30 inner() print("Inside outer:", x) outer() print("In main:", x)
The output is:
Inside outer: 30 In main: 30
From the above example, you can see that the global variable ‘x’ is accessible across both the functions and its value gets modified successfully.
Example 4: Global Keyword with List
Global variables aren’t just limited to integer or string types. We can easily use lists as global variables:
lst = [1, 2, 3] def update_list(): global lst lst.append(4) print(lst) update_list() print(lst)
This will produce the following output:
[1, 2, 3] [1, 2, 3, 4]
This shows that our ‘lst’ global variable got updated correctly inside the function as well!
More Intricate Examples of ‘Global’ Keyword Usage
Example 5: Global in If-Else Statements
Now, let’s delve into the world of conditional statements. Let’s see how to use a global variable in an if-else block:
x = 10 def check(): global x if x == 10: print("x is 10") else: print("x is not 10") check()
The above code will output:
x is 10
This simple example shows how we can use a global variable within an if-else statement.
Example 6: Global Keyword in While Loops
Let’s see how the ‘global’ keyword works inside a while loop:
n = 5 def countdown(): global n while n > 0: print(n) n -= 1 countdown()
The output will be:
5 4 3 2 1
This reveals how a ‘global’ variable maintains its value through each iteration of the while loop.
Example 7: Global Keyword in For Loops
We can also iterate through a set of values using a global variable in a for loop:
x = [] def fill_list(): global x for i in range(5): x.append(i) fill_list() print(x)
The output will be:
[0, 1, 2, 3, 4]
We used the ‘global’ keyword to insert the numbers into the global array ‘x’ within the function.
Example 8: Global Arrays in Nested Functions
For our final exercise, let’s have a look at how to use global arrays in functions nested within other functions:
x = [] def fun_outer(): global x x.append(1) def fun_inner(): global x x.append(2) fun_inner() print("Inside outer function:", x) fun_outer() print("In main function:", x)
The output is:
Inside outer function: [1, 2] In main function: [1, 2]
In this exercise, the global array ‘x’ remains accessible within both the outer and inner functions.
As these examples demonstrate, the ‘global’ keyword is a versatile and essential part of Python, particularly when working with functions and variables. By understanding and mastering ‘global’, you’re well on your way to becoming a Python pro!
Where to Go Next?
Congratulations on reaching this point in mastering Python’s ‘global’ keyword! Mastering Python is a rewarding voyage of exploration and our Python Mini-Degree is the perfect next step in your Python journey.
The Python Mini-Degree, offered by Zenva Academy, is a comprehensive collection of courses that take you deep into the world of Python programming. What will you learn in the Python Mini-Degree?
- Coding basics with Python
- Algorithms using Python
- Object-Oriented Programming (OOP) with Python
- Game development with Python
- Practical application development with Python
The comprehensive courses are designed for everyone, from beginners to experienced coders, fostering an engaging learning environment through step-by-step projects. You’ll gain the opportunity to create your own games, develop efficient algorithms, and build real-world apps.
We offer instruction from experienced instructors, interactive lessons, coding challenges, and course completion certificates. Plus, Zenva Academy gives you the flexibility to access your courses anytime and learn at your own pace.
To widen your Python horizons, make sure to check out our more extensive Python course collection.
With Zenva, Go From Beginner To Professional
At Zenva, we endeavor to make coding, game creation, and AI education accessible to everyone. We offer a diverse selection of over 250 courses to help you boost your career no matter where you’re starting from. Do you aim to learn to code, create amazing games, or leverage the power of AI? We’ve got you covered!
We provide both beginner and professional courses that can help you go from zero to hero in your coding journey. So whether you’re an absolute novice or someone looking to deepen your existing knowledge, count on Zenva to guide you.
Go ahead and start exploring the possibilities with us today!
Conclusion
Arming yourself with deep knowledge of Python’s ‘global’ keyword will truly boost your coding skills, giving you more control over your scripts and leading to cleaner and more performant code. As you continue upskilling with us, remember these fundamentals will lay a solid foundation for your future coding projects. Whether you’re forecasting data trends or developing an exciting game, understand that every ‘global’ keyword has its place in your journey.
Ready to take your Python mastery to the next level? Elevate your learning and jump into the real-world projects with our comprehensive Python Mini-Degree. We look forward to helping you write your coding success story, one line of code at a time.
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
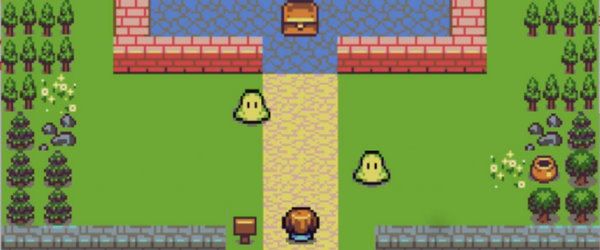
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.