Welcome to this comprehensive tutorial on Python’s hash function. Python’s hash is an intriguing tool that may seem baffling at first but provides immense value once mastered. In this tutorial, we aim to dismantle the complexity of Python’s hash function and show you the magic behind it. Through a series of engaging examples and friendly explanations, we want to ease your journey into mastering this vital function.
Table of contents
What is Python’s Hash Function?
Python’s hash is a built-in function in the Python programming language. Hash, in simple terms, is a way to convert data of arbitrary size to fixed-size data. It is used to create unique identifiers for large amounts of data. Doesn’t it sound intriguing already? Let’s dive deeper to answer the next big question.
What is the Function Used For?
If you’ve been wondering why you would use Python’s hash function, wonder no more! Here’s where its magic really stands out:
- Hashing is primarily used for table lookup or data comparison.
- It’s a common method used in database indexing to efficiently retrieve items.
- In gaming, hash functions can be used to save game states or create unique object identifiers.
Why Should I Learn It?
With Python being a popular language in various fields such as web development, data science, AI, and game development, understanding the hash function is like adding a powerful arrow in your programming quiver. It not only helps you design efficient algorithms but also boosts your problem-solving abilities. Trust us when we say, this is a tool you want to master, and here’s your chance!
Understanding Python’s Hash Function through Examples
Let’s start with the basics. To compute the hash value, we use the syntax hash(object). Now, let’s dive into examples.
Example 1: Hash for integer
# Computing hash for integer print(hash(181))
This will output a unique hash value for the integer 181.
Example 2: Hash for float
# Computing hash for decimal print(hash(181.23))
Similar to integers, Python’s hash function can generate hash values for float numbers too.
Example 3: Hash for string
# Computing hash for strings print(hash('Zenva'))
Strings can also be hashed to generate unique identifiers, which can be particularly useful in many applications.
Python’s Hash Function: Advanced Examples
Now that we have covered simple types of data, let’s see some advanced examples.
Example 4: Hash for tuple
# Computing hash for tuple print(hash(('Zenva', '
', 'Tutorial')))
In Python, we can also calculate the hash for tuples. This is handy in scenarios where we want to maintain sequence and need a unique identifier.
Example 5: Hash for objects
# Hash for custom object class Zenva: def __init__(self, title, course): self.title = title self.course = course obj = Zenva('
', 'Unity') print(hash(obj))
Here we have a simple Zenva class. When we try to hash its instance, it raises an error. Python doesn’t know how to hash the custom object. To solve this, we need to include the __hash__ method in our class.
Example 6: Hash for objects with a custom hash method
# Customizing hash for custom object class Zenva: def __init__(self, title, course): self.title = title self.course = course def __hash__(self): return hash((self.title, self.course)) obj = Zenva('Game Development', '
') print(hash(obj))
This time the hash is computed. We provided a custom hash computation by hashing a tuple of properties.
By now, you should have a good grasp of the basics of Python’s hash function and how it can be used in various data types. Remember, the key to mastering any concept is practice, so don’t forget to play around with these examples and try creating your own.
Digging Deeper into Python’s Hash Function
Now that you understand the basics, let’s dive deeper and explore some more intriguing aspects of Python’s hash function. We’ll provide more complex examples for you to experiment with and enhance your knowledge.
Example 7: Hash Immutability and hash collisions
# Immutable hash and Hash collision team_A = ('Zenva', 'Game Development', 2021) team_B = ('Zenva', 'Game Development', 2022) # Same hash for immutable data print(hash(team_A) == hash(team_A)) # Different hash for different but similar data print(hash(team_A) == hash(team_B))
In this example, we show that the hash of an unchanged (immutable) object remains the same. However, even if the data is very similar, the hash will be different unless all the elements are identical. This difference helps in efficiently searching or indexing data in large datasets.
Example 8: Hash for Lists?
# Trying to hash a list my_courses = ['Unity', 'Python', 'AI'] print(hash(my_courses))
When the above code is executed, Python throws a TypeError stating that lists are unhashable. This occurs because lists are mutable, meaning they can change over time. Therefore, their hash value is unstable and could change, violating the rule of hashing.
Example 9: Working around mutable objects
# Hashing the representation of a list my_courses = ['Unity', 'Python', 'AI'] print(hash(str(my_courses)))
If you must hash a mutable object, like a list, one workaround is to convert it to a string before calculating the hash. This should be used with caution, especially when working with large data sets, as it may lead to unintended collisions.
Example 10: Hashing and the None object
# Hash for None print(hash(None))
Unsurprisingly, Python’s built-in None object also has a hash value defined. This returns a constant value, similar to other built-in immutable data types.
Example 11: Hashing Boolean values
# Hash for Boolean print(hash(True)) print(hash(False))
Even Boolean values return their own unique hash values, with True and False always returning the same results each time.
Example 12: Dictionaries and Hashing
# Hash keys game_dict = {"name": "Zenva Battle", "platform": "PC", "publisher": "Zenva"} for key in game_dict: print(f"{key} - {hash(key)}")
Dictionaries in Python use the hash values of keys, providing us fast access to the associated values. This example shows us the hash values of each key in a dictionary.
Through these advanced examples, we hope you have journeyed a step further in understanding the utility and versatility of Python’s hash function. Continue experimenting and practising, and remember, mastery comes with time and perseverance. Don’t feel overwhelmed if you don’t understand something at first – that’s why we’re here!
Where to Go Next
We hope you’ve enjoyed the dive into Python’s hash function. If you’re raring for more, we at Zenva, your dedicated e-learning companions are here to help you continue your journey!
A great next step would be to start or continue with our Python Mini-Degree. The Mini-Degree includes a comprehensive range of courses that dive deep into Python programming. Python is highly regarded in the industry for its simplicity and versatility, making it popular for a range of applications, including game development and web development.
The Python Mini-Degree covers a wide array of topics like:
- Coding fundamentals
- Python algorithms
- Object-oriented programming
- Game development, and much more
Each tutorial is designed to cater to both beginners and experienced programmers with step-by-step projects that guide you in crafting games, algorithms and real-world apps.
What makes our mini-degree unique is the emphasis we place on building a portfolio of Python projects. Not only are you equipped with Python skills but you also end up with a presentable portfolio to showcase your abilities.
Our Python courses cater to all levels of Python enthusiasts. If you’ve sailed past the basics and are looking forward to more intermediate-level learning, we’ve got you covered.
Conclusion
Embarking on a journey of mastering Python’s hash function is a wonderful opportunity to enhance your programming skills. As we illustrated in this tutorial, the hash function is a powerful tool in your Python programming arsenal capable of boosting your ability to design efficient algorithms and problem-solving capabilities. The ability to hash various data types, whether an integer, float, string, or a custom object, provides endless potential for performance and efficiency improvements in your code.
At Zenva, our aim is to provide you with the tools, knowledge, and resources you need to succeed in learning to code. With our comprehensive Python Mini-Degree, you can continue bolstering your Python skills. So don’t stop here. Keep exploring, keep asking questions, and above all, keep coding. The world of Python awaits you! Happy Learning!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
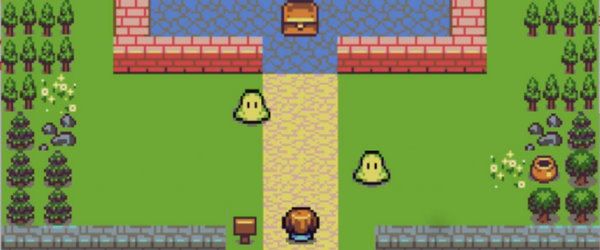
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.