Welcome to this exciting tutorial on Python Reflection in Object-Oriented Programming (OOP). Through this guide, we aim to simplify this concept and approach it through a gaming lens to make learning both interactive and fun. Brace yourself for an enthralling journey into Python coding.
Table of contents
What is Python Reflection in OOP?
Reflection refers to the ability of a program to inspect its own properties, particularly at runtime. In the world of Python, it allows us to delve deeper into object attributes and methods, exploring the structure and function of objects beyond what conventional coding permits.
Just as a mirror reflects reality, Python Reflection in OOP mirrors the program’s structure, enabling us to interact with the program dynamically. This introduces a level of flexibility unachievable with static coding.
Benefits of learning Python Reflection
Understanding Python Reflection puts you in a position to:
- Manipulate, inspect, and interact with Python objects in a dynamic manner
- Build more flexible and adaptable programs
- Debug your code more efficiently
Through the lens of gaming, these benefits translate into the ability to develop sophisticated game mechanics that adapt to player actions in real-time.
Buckle up as we dive into the coding section of this tutorial! We promise you an adventurous and enriching ride into the realm of Python Reflection in OOP.
Introductory Applications of Python Reflection
Let’s now walk through some fundamental code examples demonstrating Python Reflection in action. For starting our journey, we will use Python’s built-in functions such as type(), dir(), and getattr().
The type() Function
The type() function allows us to determine the type/class of an object.
class GameObject: pass player = GameObject() # Check the type of player print(type(player))
This program would output: <class ‘__main__.GameObject’>, reflecting the type of our player object.
The dir() Function
The dir() function allows us to access an object’s attributes and methods.
class GameObject: def __init__(self, name): self.name = name player = GameObject("Hero") # Access the attributes and methods of player print(dir(player))
This will output a list of attributes and methods, including the name attribute.
Advanced Applications of Python Reflection
Now that we’re comfortable with the basics, let’s move on to more advanced applications. We will introduce the getattr() and setattr() functions and show how they can be utilized to create more complex game mechanics.
The getattr() Function
The getattr() function can access the value of an object’s attribute using its name as a string.
class GameObject: def __init__(self, name): self.name = name player = GameObject("Hero") # Access player's name using getattr player_name = getattr(player, "name") print(player_name)
This will output “Hero”, reflecting the name of the player.
The setattr() Function
The setattr() function sets the value of an object’s attribute.
class GameObject: def __init__(self, name): self.name = name player = GameObject("Hero") # Use setattr to change player's name setattr(player, "name", "Super Hero") print(player.name)
The new name, “Super Hero”, will be printed as output, showing that Python Reflection can power dynamic changes to our game objects.
Delving Deeper with Python Reflection
Now that we have control over object attributes, let’s further our exploration by looking at instances, checks and built-in Python libraries.
Checking if an Attribute Exists
We can check if a class has a particular attribute using the hasattr() method.
class GameObject: def __init__(self, name): self.name = name player = GameObject("Hero") # Check if player has a 'name' attribute print(hasattr(player, "name"))
This will print ‘True’, reflecting the presence of the ‘name’ attribute for our player GameObject.
The isinstance() Function
We can use the isinstance() function to determine if an object is an instance of a particular class.
class GameObject: pass player = GameObject() # Check if player is an instance of GameObject print(isinstance(player, GameObject))
This outputs ‘True’, indicating that player is indeed an instance of the GameObject class.
Adding Methods Dynamically
In Python, we can add methods to instances dynamically, offering a high level of flexibility and control over our objects. Here’s an example:
class GameObject: pass player = GameObject() # Dynamically adding a method to our object def playerGreet(self): return "Hello, I am your player." player.greet = playerGreet print(player.greet(player))
This gives the output: ‘Hello, I am your player.’ Notice that the player object did not originally have a greet method; we added it dynamically using the reflection.
The inspect Module
Python offers a built-in library, inspect, that provides several functions to help get live information about live objects such as modules, classes, methods, functions, etc.
import inspect from some_module import SomeClass class ChildClass(SomeClass): pass print(inspect.getmro(ChildClass))
‘getmro’ function returns a tuple of classes that would be visited to look for methods in case of multiple inheritance. It’s a powerful tool which is part of Python Reflective capabilities.
Charting the Path Ahead: Your Future with Python
Having acquired a good understanding of Python Reflection in OOP, you might be asking, “Where do I go from here?” The world of Python programming is broad and dynamic, offering countless avenues for further learning and exploration.
The Python Mini-Degree offered by us at Zenva Academy is an elaborate and exhaustive bundle of courses centered on Python programming. It affords aspiring Python maestros an opportunity to get their hands dirty with the intricacies of Python, covering everything from the fundamentals of coding and algorithms to the nuances of object-oriented programming, game development, and real-world app creation.
Why opt for our Python Mini-Degree, you ask? Here are some compelling reasons:
- Navigates you step-by-step through exciting projects, letting you build games, algorithms, and apps that reflect real-world scenarios
- Equips you with a versatile skillset in Python, a language renowned for its simplicity and flexibility.
- Offers you the convenience to learn at your own pace, helping you juggle your learning schedule according to your needs.
- Reinforces learning through live coding lessons, quick challenges, quizzes, and interactive sessions
- Enables you to create a professional portfolio of Python projects, significantly bolstering your career prospects
Our team of instructors are certified by Unity Technologies and CompTIA. They are experienced coders, dedicated to assisting you in your journey from beginner to professional.
Apart from the Python Mini-Degree, we also offer a wide range of Python-focused courses. Feel free to explore these resources to deepen and diversify your Python knowledge further.
Conclusion
Python Reflection in OOP provides exceptional flexibility to programs, making it a valuable concept in modern programming. As you’ve seen, it’s instrumental in game development, enabling you to create dynamic game mechanics that respond to player actions in real-time.
Ready for the next level in your Python learning journey? Unlock the power of Python with Zenva’s Python Mini-Degree. Stay curious, keep exploring, and let’s code a brighter future together!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
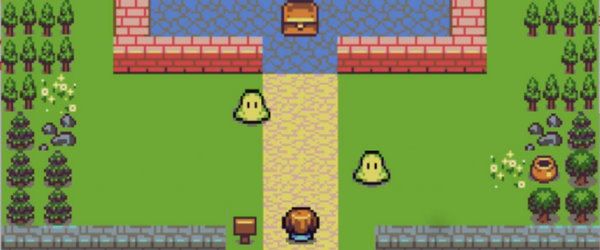
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.