Welcome to this interactive tutorial where we dive into the fundamental techniques of Python exception handling. Exception handling is a crucial part of any programming language, and Python is no exception. It ensures your code can elegantly handle errors, making your programs more robust and user-friendly. Do you want your applications to be more resilient and your development process to be more efficient? Then, mastering Python’s exception handling mechanism is key.
Table of contents
Understanding Python’s Exception Handling
An “exception” is an event that occurs during the execution of a program, disrupting the normal flow of the script. In simpler terms, think of it as a hurdle in the racecourse of your application’s execution. Without handling, this hurdle can cause your program to trip and collapse. Understanding exceptions is crucial for any developer, beginner or experienced, intent on crafting reliable and efficient applications.
Why Should You Learn Python Exception Handling?
Exception handling in Python plays an essential role in improving your code’s resilience. A well-designed system for exception handling can:
- Improve the reliability of your applications, ensuring they keep running smoothly even when an error occurs.
- Enhance the user experience by presenting meaningful error messages instead of abruptly crashing.
- Facilitate the debugging process, helping to quickly and efficiently identify the root cause of errors.
Even if you are at the start of your coding journey, or you’ve been coding for a while, mastering Python exception handling now will undoubtedly give you a good foundation and a head-start on your programming path. This tutorial will equip you with the indispensable techniques to handle exceptions in your Python applications. Stay with us, and let’s dive into some code!
Basic Exception Handling
In Python, we handle exceptions using the try/except block. A try block contains the code that could potentially raise an exception, while an except block is used to catch and handle that exception. Let’s look at an example:
try: print(5/0) # This will raise a ZeroDivisionError except: print("An error occurred!")
In this specific case, when Python executes the operation 5/0 and encounters a ZeroDivisionError, the program doesn’t crash. Instead, it moves to the except block and prints, “An error occurred!”
Handling Specific Exceptions
Python allows us to catch specific exceptions. This can be extremely useful when you anticipate different types of errors and want to handle them differently.
try: print(5/0) # This will raise a ZeroDivisionError except ZeroDivisionError: print("You can't divide by zero!")
Here, when a ZeroDivisionError is caught, we print a more meaningful message, “You can’t divide by zero!”
Using the else Clause
In Python’s exception handling, the else clause is executed if no exception is raised in the try block. Here’s how it works:
try: print(5/2) # This operation won't raise an error except ZeroDivisionError: print("You can't divide by zero!") else: print("The operation was successful.")
In the above snippet, since no exception is raised in the try block, the else block is executed, printing “The operation was successful.”
The finally Clause
The finally clause is executed regardless of whether an exception was raised or not, making it great for releasing external resources.
try: print(5/0) # This will raise a ZeroDivisionError except ZeroDivisionError: print("You can't divide by zero!") finally: print("This will always run.")
Even though a ZeroDivisionError was raised, the finally block still runs, printing “This will always run.” This is because finally is executed no matter what happens in the preceding blocks.
Raising Exceptions
Beyond handling exceptions, Python allows us to manually trigger, or “raise,” exceptions using the raise statement.
try: raise ValueError("This is a custom error message!") except ValueError as e: print("A ValueError occurred: ", e)
In this example, we are raising a ValueError exception with a custom error message, which is then caught and displayed.
Chaining Exceptions
Python 3 has given us the capability to chain exceptions, allowing an exception to raise another exception while maintaining the stack trace of the first exception.
try: 5/0 except ZeroDivisionError as e: raise RuntimeError("A runtime error occurred") from e
When a ZeroDivisionError is thrown, we then raise a RuntimeError with our custom message, indicating that “A runtime error occurred”.
Assert Statement for Exception Checking
Python has a built-in assert statement that we can use to trigger exceptions when a specified condition is not met.
x = 5 assert x < 5, "x should be less than 5"
If we run the above code, an AssertionError will be thrown with the message, “x should be less than 5”.
Using Exceptions with User-Defined Functions
Let’s look at how we can incorporate exception handling in a user-defined function, adding robustness to our code.
def divide_numbers(a, b): try: result = a / b except ZeroDivisionError: print("You can't divide by zero!") result = None finally: if result is None: print("Operation failed.") else: print("Operation successful.") return result result = divide_numbers(5,0)
Here, we have built a safer division operation function that provides meaningful feedback to the user and ensures the integrity of the application. Always remember that catching and handling exceptions in your Python code will help you build more stable and user-friendly applications.
Next Steps in Your Python Journey
After exploring Python exception handling, it’s important to note that learning is an ongoing process. Becoming a proficient coder requires constant practice, exploration, and curiosity. So what’s next? We encourage you to delve deeper into the world of Python programming by exploring our extensive course collections.
At Zenva, we offer an impressive Python Mini-Degree, an extensive compilation of courses designed to transform you from a Python novice into a confident programmer. Our mini-degree covers a full spectrum of Python-related subjects, ranging from coding basics to sophisticated subjects like algorithms, object-oriented programming, and even game and app development.
Through the course, you will embark on actionable, project-based learning that will see you creating a medical diagnosis bot, a to-do list app, and numerous engaging pieces of software.
You’ll find our instructors incredibly experienced and certified, with a knack for bringing complex Python concepts to life. Our courses feature interactive lessons, practical quizzes, and hands-on coding challenges designed to solidify your understanding and immerse you in a real-world coding environment.
With us, you can study at your own pace, anytime and anywhere, on any device. At the end of the journey, you’ll receive a certificate recognising your hard-earned skills and a plethora of projects to showcase in your portfolio.
For those desiring a more wide-ranging scope, we boast an abundance of Python courses covering diverse topics. Python is increasingly becoming a must-have skill in the job market, with its applications extending beyond traditional development to fields like data science, machine learning, and AI.
Conclusion
Python exception handling not only equips you with the ability to write robust, error-resistant code, but also supercharges your path to becoming a proficient Python developer. This skill is but one of the many tools you’ll leverage as you navigate the expansive landscape of Python programming. At Zenva, we’re fully committed to supporting your learning curve and ensuring you acquire the most rewarding coding skills in an engaging, flexible, and efficient manner.
Join our Python Mini-Degree or any other course from our extensive collection to further strengthen your Python programming abilities. Your ideal coding career, be it in web development, data science, artificial intelligence, or game development, is just a few confident strides away. Let’s embark on this exciting journey together and conquer the tech world with Python!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
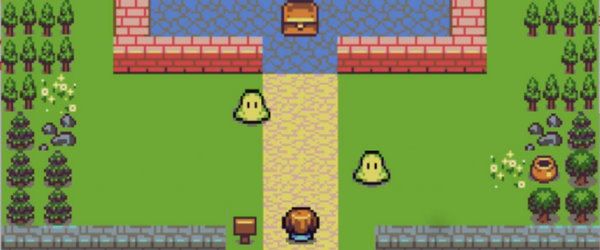
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.