In this tutorial, we’re diving into the realm of Python programming, specifically focusing on Python class and instance attributes. Whether you’re an aspiring game developer, an AI enthusiast or a beginner setting out on your coding journey, grasping these Python concepts can act as a foundational stepping stone in your learning ride.
Table of contents
Understanding Python Class and Instance Attributes
Python, as an object-oriented programming language, thrives on the creation and manipulation of objects. This feature introduces us to classes, the blueprints for these objects, and their attributes. The beauty of Python is that it allows us to define both class and instance attributes. But what separates the two?
Class Attributes vs Instance Attributes: The Basics
A class attribute is a value shared across all instances of a class, meaning every object created from that class will access the same attribute value. On the other hand, an instance attribute is uniquely tied to each object created from a class. Each instance can have a different value for that attribute, giving each object its uniqueness.
Why Learn About Python Class and Instance Attributes?
Hence, understanding the dynamic between class and instance attributes is crucial to controlling object behavior. By mastering these, you can create class blueprints that are flexible, reusable and efficient – opening up diverse game mechanics and further expanding the functionality of your Python projects. Whether you’re a beginner or an experienced coder, these skills will uplift your programming prowess.
Up next, we will be exploring some code examples to better illustrate the concepts we’ve discussed. So, if you’re excited to see Python class and instance attributes in action, read on!
Python Class Attribute: An Illustration
Let’s start simple with a basic class attribute example. We will create a class for a videogame character where all the instances of characters share a common attribute: the game they belong to.
class VideoGameCharacter: game = "Vision Quest" character1 = VideoGameCharacter() character2 = VideoGameCharacter() print(character1.game) #Output: Vision Quest print(character2.game) #Output: Vision Quest
As you can see, both objects or instances we created (character1, character2) inherit the attribute ‘game’ from the class and have the same value.
Python Instance Attribute: An Illustration
Now, let’s look at instance attributes by creating a new attribute for our characters – name. Each character should have a unique name.
class VideoGameCharacter: game = "Vision Quest" def __init__(self, name): self.name = name character1 = VideoGameCharacter("Lara") character2 = VideoGameCharacter("Samus") print(character1.name) #Output: Lara print(character2.name) #Output: Samus
Each created instance(character1, character2) now has its unique ‘name’ attribute, highlighting the essence of instance attributes.
Overriding Class Attributes with Instance Attributes
In Python, you can override a class attribute with an instance attribute. For instance, if a certain character doesn’t belong to the game “Vision Quest”, we can specify a different game for that character:
class VideoGameCharacter: game = "Vision Quest" def __init__(self, name, game=None): self.name = name if game: # if game parameter is provided self.game = game character1 = VideoGameCharacter("Lara", "Raiders Legacy") print(character1.game) #Output: Raiders Legacy
Though the class attribute ‘game’ was set to “Vision Quest”, we were able to override it for character1 by specifying a different game when creating the instance.
Modifying Class Attributes
Remember also that modifying a class attribute will change its value for all instances, unless it’s been overridden by an instance attribute. Let’s modify our class attribute ‘game’ after creating some instances:
class VideoGameCharacter: game = "Vision Quest" def __init__(self, name): self.name = name character1 = VideoGameCharacter("Lara") character2 = VideoGameCharacter("Samus") VideoGameCharacter.game = "Adventurers Guild" print(character1.game) #Output: Adventurers Guild print(character2.game) #Output: Adventurers Guild
See how the change in class ‘game’ attribute reflects on all instances, altering ‘game’ attribute value of both character1 and character2.
Creating Dynamic Class Attributes
In Python, you’re not confined to defining class attributes at the beginning of the class. You can create them dynamically at any point during execution.
class VideoGameCharacter: def __init__(self, name, power): self.name = name self.power = power character = VideoGameCharacter("Raiden", "Electricity") VideoGameCharacter.game = "Mortal Kombat" print(character.game) #Output: Mortal Kombat
Here, we added a class attribute ‘game’ after creating an instance, yet the instance was able to access this attribute.
Instances with Multiple Class Attributes
A Python class can have multiple class attributes and they can all be accessed by instances.
class VideoGameCharacter: game = "Outworld Adventures"
= "NetherRealm Studios def __init__(self, name): self.name = name character = VideoGameCharacter("Raiden") print(character.game) #Output: Outworld Adventures print(character.developer) #Output: NetherRealm Studios
Updating Instance Attributes
You can also dynamically update an instance attribute for any object, which won’t affect other instances.
class VideoGameCharacter: def __init__(self, name, power): self.name = name self.power = power character1 = VideoGameCharacter("Raiden", "Electricity") character2 = VideoGameCharacter("Scorpion", "Fire") print(character1.power) #Output: Electricity print(character2.power) #Output: Fire character1.power = "Thunder" print(character1.power) #Output: Thunder print(character2.power) #Output: Fire
Unsuccessful Instance Attribute Access
If you try to access an instance attribute that has not been defined, Python will raise an AttributeError.
class VideoGameCharacter: game = "Outworld Adventures" def __init__(self, name): self.name = name character = VideoGameCharacter("Raiden") print(character.power) #Raises AttributeError: 'VideoGameCharacter' object has no attribute 'power'
In this example, ‘power’ was not defined as either a class or an instance attribute but we tried to access it, causing Python to raise an AttributeError.
Checking for Attribute Existence
Fortunately, Python allows us to check for an attribute’s existence using hasattr() function before trying to access it, avoiding runtime errors.
class VideoGameCharacter: game = "Outworld Adventures" def __init__(self, name): self.name = name character = VideoGameCharacter("Raiden") if hasattr(character, "power"): print(character.power) else: print("Attribute power does not exist")
This will print “Attribute power does not exist” instead of raising a runtime AttributeError. This kind of code allows us to create more robust and error-resistant applications.
Continue Your Python Journey with Zenva
Congratulations on making it this far! We hope you found this tutorial on Python Class and Instance Attributes informative and engaging. But, don’t stop here. Python is an expansive programming universe with endless opportunities from game development and AI to web and app creation.
We invite you to check out our Python courses catalog that caters to a spectrum of learning goals.
One of our highly recommended learning paths is the Python Mini-Degree. This is a comprehensive collection of courses that guide you through the ins and outs of Python. Whether you’re interested in exploring coding basics, dabbling with algorithms, venturing into object-oriented programming or setting foot in game and app development, this mini-degree will have something for you.
You’ll be learning through engaging hands-on projects, creating your own games, algorithms and apps. Not only does this reinforce your learning, but it also enables you to build a portfolio to showcase your Python prowess to potential employers. Our course content is regularly updated to keep you in sync with industry trends, ensuring you’re always at the top of your game.
So, no matter where you are in your Python journey, let Zenva be the guide that helps you reach new coding heights! Keep coding, continue exploring and happy learning!
Conclusion
We’ve had an exciting journey exploring Python class and instance attributes, uncovering their power and potential in making your coding endeavors more efficient and adaptable. Certainly, these tools, when mastered, can add subtlety and robustness to your creations – be it games, apps, AI solutions, and more.
At Zenva, we’d love to be part of your Python learning journey. We encourage you to delve deeper into this programming language, exploring its vast possibilities with our Python Mini-degree. Our engaging, hands-on courses can prove to be your companion in mastering Python, arming you with the prowess to turn your programming ideas into reality. Continue learning, and remember, the depth of Python’s potential is limited only by the breadth of your imagination!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
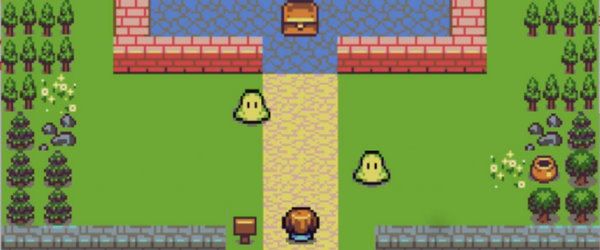
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.