Welcome, coding explorers! In this fascinating dive into Python, we will explore the world of the Python OS module. This often-underutilized tool within the Python ecosystem carries immense power and can significantly streamline your coding efforts if appropriately leveraged.
Table of contents
What is Python OS Module?
The Python OS module (OS standing for Operating System) is a built-in Python library that provides a portable way of using OS-dependent functionality. It acts as an interface between Python and your computer’s operating system, enabling you to interact with underlying system functionalities directly from your Python script.
With the Python OS module, you can perform various file and directory operations, manipulate paths, read and set environmental variables, and even handle child processes.
Why Should You Learn It?
Learning to use the Python OS module can greatly enhance your productivity as a programmer. It’s not only about expanding your Python knowledge but also about acquiring skills to interact directly with your OS, giving you the power to automate tasks, manage files efficiently and enhance your program’s capabilities and efficiency.
Imagine you are creating a simple game. Using the Python OS Module, you could create a game save system, automatically creating, reading, and writing files for each game session. Or suppose you are working on a larger project. In that case, you can employ this module to handle directories dynamically, making your code cleaner, more efficient and easily maintainable.
No matter what your coding journey looks like, having the Python OS module in your toolkit will surely open new pathways to more exciting, efficient and streamline coding adventures.
Python OS Module Basics
Let’s start by importing the OS module. This can be achieved as follows:
import os
Once imported, you can use various functions to interact with your operating system. Let’s explore some of the basic functionalities.
OS Module Directory and File Operations
The OS module provides a bunch of methods to manage and manipulate files and directories. Here are some simple yet powerful examples:
# Creating a new directory os.mkdir("new_directory") # Listing content of a directory os.listdir() # Renaming a directory os.rename("new_directory", "renamed_directory") # Removing a directory os.rmdir("renamed_directory")
Python OS Module Path Manipulation
The OS module is also very good at handling path-related tasks. This is a skill every programmer should master well!
# Joining two or more pathname components os.path.join("/home/zenva", "tutorials") # Splitting a pathname. Returns tuple "(head, tail)" where "tail" is the part after the last slash. os.path.split("/home/zenva/tutorials") # Getting the current working directory os.getcwd() # Checking if a path exists os.path.exists("/home/zenva/tutorials")
That covers some of the most commonly used functionalities of the Python OS module. In the next section, we’ll dive into more advanced functionalities.
Advanced Functionalities with the Python OS Module
Let’s now move on to the more advanced capabilities of the Python OS module that will truly help heighten your productivity as a programmer.
Environment Variables
Using the Python OS module, you can directly interact with environment variables. This can be really handy when you’re dealing with various deployment environments or secret API keys.
# List all environment variables os.environ # Get value of a specific environment variable os.getenv('HOME') # Set value of an environment variable (available for the duration of your script) os.environ['API_KEY'] = 'your-secret-api-key'
Executing a Shell Command
Another great functionality that is frequently useful involves executing Shell commands directly from Python.
# Execute a shell command os.system('ls')
Working With Files
The OS module is not only about interacting with directories. It can also do wonders when working with files!
# Get the size of a file os.path.getsize("/path/to/your/file") # Get the time of the last access os.path.getatime("/path/to/your/file") # Get the time of the last modification os.path.getmtime("/path/to/your/file")
Process Handling
Lastly, the OS module can be used for creating and managing processes, contributing to optimizing the overall performance of your programs.
# Creating a new process new_process = os.fork() # Checking process id os.getpid() # Waiting for process completion os.waitpid(-1, 0)
These built-in functionalities are just the tip of the iceberg when it comes to what’s possible with Python’s OS module. We encourage you to delve into the official Python documentation for a detailed look into the complete feature set of the module. Coding with Python has a lot of potential, so keep exploring and learning and you won’t be disappointed with the world of possibilities that open up for you!
Where to go next?
Now that you’ve got a grasp of the Python OS module, you might be wondering – what’s next? How do I continue my journey and keep learning?
One of the best routes to keep progressing in Python is to check out our Python Mini-Degree. This comprehensive collection of courses delves deep into Python programming, covering not only the basic syntax and algorithms, but also object-oriented programming, game development, and app creation. With projects such as building games, medical diagnosis bots, and real-world applications, you can elevate your Python knowledge to new heights.
Our courses are designed to be accessible and flexible, enabling learners to study at their own pace, from the comforts of their homes. With video lessons, quizzes, and completion certificates included, the Python Mini-Degree is an all-inclusive path for learners of all levels, from beginners just starting out to more advanced programmers looking to broaden their Python skills.
Additionally, we invite you to explore our broader collection of Python courses. Delve into more focused topics, refine specific skills, or just broaden your understanding of what is possible with Python.
Conclusion
Delving into the Python OS module has opened up a whole new gamut of possibilities in your programming journey. Like any good explorer, you’ve taken the time to understand and appreciate one more cornerstone in the magnificent structure of Python programming.
Now that you are equipped with the power of the OS module, don’t stop here. It’s time to apply this newfound knowledge, build upon it, and create something remarkable! Check out our Python Mini-Degree program and continue this thrilling development adventure. We at Zenva are always here to guide you and help you hone your skills. The world of programming is your playground – let’s explore it together!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
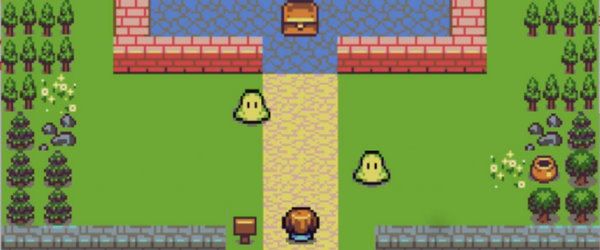
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.