Have you ever been stuck with a Python script running for what feels like an eternity? Wondered how you could make your code execute faster? Today’s tutorial aims to shed light on Python optimization, a topic often overlooked by beginners and sometimes even by seasoned coders. Learning how to optimize your Python code can significantly enhance the efficiency, speed, and overall performance of your work. Join us as we dive deep into the nuts and bolts of Python Optimization!
Table of contents
What is Python Optimization?
Python Optimization refers to the process of improving your code in terms of efficiency and speed. This usually involves minimizing the computational complexity of your code, reducing memory usage, or speeding up the execution time.
Why Should You Learn It?
Optimization is an integral part of programming, irrespective of which language you are using. For Python developers, it is even more crucial because Python, being an interpreted language, can sometimes be slower than its compiled counterparts like C++ or Java.
By learning how to optimize Python, you are enhancing the performance of your code, and this can be extremely beneficial, especially when you work with large data sets or complex algorithms. You’d be surprised at how much time and resources you could save with some simple optimization techniques!
Better yet, Python Optimization doesn’t just make you a better coder—it makes you stand out from the crowd. This niche skill can be a selling point in job interviews, a key to advancing your career, or even just a way to impress your codemaking peers at your next hackathon.
Unlock the full potential of Python by mastering Python Optimization!
The Basics of Python Optimization
Let’s kick off this part of our tutorial by walking through some essential strategies to optimize your Python code:
Using Built-in Functions
Python’s built-in functions are optimized down to the C level, hence they are generally faster than writing your custom functions. Let’s look at an example:
# Custom function to multiply all elements in a list. def custom_multiply(input_list): result = 1 for num in input_list: result *= num return result # Using Python's built-in function for multiplication. import functools def built_in_multiply(input_list): return functools.reduce(lambda x, y: x * y, input_list)
By utilizing Python’s built-in functions, you can achieve the same results with fewer lines of code and less execution time.
Avoiding Unnecessary Loops
Loops in Python can be costly, and their misuse can lead to significant increases in execution time. Let’s check out an example:
# Avoid this new_list = [] for i in old_list: if i not in new_list: new_list.append(i) # Optimize it this way new_list = list(set(old_list))
In the optimized version, we’re using Python’s built-in set function to remove duplicates from the list, eliminating the need for a loop.
Using List Comprehension
List comprehension allows you to replace many for and while blocks with a simple, readable and more efficient single line of code. Here’s an example:
# Avoid this new_list = [] for i in num_list: new_list.append(i * 2) # Optimize it this way new_list = [i * 2 for i in num_list]
Using Local Variables
Making use of local variables over global variables can help speed things up, especially in large applications. Let’s observe this in action:
# Avoid this global_var = 5 def test_func(n): for i in range(n): print(global_var) # Optimize it this way def test_func(n): local_var = 5 for i in range(n): print(local_var)
The optimized version of the function uses a local variable, which will run faster than the version with a global variable.
These simple but effective optimization techniques can make a big difference in the efficiency of your Python code. Stay tuned for more advanced methods in our next section!
Advanced Python Optimization Techniques
Ready to kick it up a notch? Let’s plunge into some advanced Python optimization strategies:
Using ‘in’ with Sets and Dictionaries
The ‘in’ operator is more efficient when used with sets and dictionaries compared to lists and tuples, especially for large data sizes.
# Avoid this if value in my_list: # Optimize it this way if value in my_set:
Concatenating Strings
For string concatenations, it’s more efficient to use Python’s .join() function rather than the ‘+’ operator:
# Avoid this s = "" for substring in list_of_strings: s += substring # Optimize it this way s = "".join(list_of_strings)
Factorizing Constant Expressions
Constant expressions can be computed once and stored, instead of being computed in every loop.
# Avoid this for i in range(len(my_list)): # Optimize it this way list_length = len(my_list) for i in range(list_length):
Using Generators
Generators are a great Python feature that generate values on the fly, consuming less memory than lists or tuples.
# Avoid this def square_numbers(nums): result = [] for i in nums: result.append(i * i) return result # Optimize it this way def square_numbers(nums): for i in nums: yield (i * i)
Profiling Your Code
Python’s built-in cProfile module can help identify bottlenecks in your code and guide you on where to focus your optimization efforts.
# Example usage import cProfile cProfile.run('YourFunction()')
Using NumPy Arrays
If you are working with numerical data, using NumPy arrays can drastically increase your program’s speed. They are more efficient and provide a great set of library functions.
# Avoid this list1 = [1, 2, 3] list2 = [4, 5, 6] product_list = [a*b for a, b in zip(list1, list2)] # Optimize it this way import numpy as np array1 = np.array([1, 2, 3]) array2 = np.array([4, 5, 6]) product_array = array1 * array2
Armed with these advanced optimization techniques, your Python code is set to run faster and more efficiently. Remember, optimized Python code not only reduces execution time but also enhances readability, making your code more understandable.
Continuing Your Python Optimization Journey
Now that we have outlined Python optimization basics and advanced techniques, the question arises: What’s next? The learning doesn’t stop here!
For those determined to master Python in-depth and accelerate their career, we highly recommend checking out our Python Mini-Degree. This comprehensive collection of courses teaches Python programming, covering everything from coding basics to algorithms, object-oriented programming, game development, and app development.
With the Python Mini-Degree, not only will you learn Python, one of the most popular programming languages in the world, you’ll also be given the opportunity to apply these skills in real-world scenarios. You will be creating your own games, designing algorithms, and even developing apps.
Boost your portfolio and prepare for the job market with this flexible, 24/7 accessible program. Python is a sought-after competency, especially in fields like data science. So why wait to become a Python pro?
What really sets our learning platform apart is the community. At Zenva, we pride ourselves on bringing together over a million learners and developers. Our students have used their skills to create their own games and websites, secure jobs, and even start businesses. With our qualified and experienced instructors, you’re always in good hands.
If you’re looking for a more specific Python skill set or want to delve into a different area, don’t worry. We’ve got you covered with our broad collection of Python courses.
Conclusion
Python optimization is a crucial skill every developer needs to master. It can make the difference between a program that takes minutes to run and one that takes seconds, empowering you with the ability to tackle bigger, more complex projects. Further, it sets you apart and gives you an edge in the ever-competitive realm of tech and programming.
We invite you to join the ranks of our many successful students. Whether you’re a beginner just getting your feet wet, or a seasoned programmer looking to broaden your knowledge, dive into the world of Python with our comprehensive Python Mini-Degree. Let’s embark on this journey to enhance your Python skills and career prospects, together!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
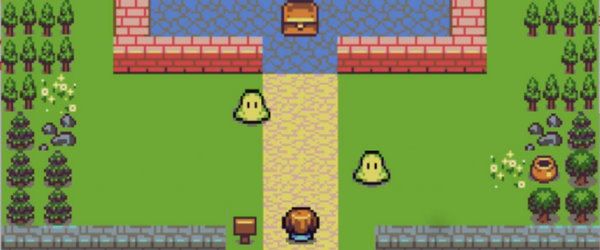
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.