Welcome to this tutorial where we will delve into the fascinating world of Python and Oracle Database connectivity. This subject holds the key to unlocking a whole new world of potential in your coding journey, and we are excited to guide you through it.
Table of contents
What is Python Oracle Database Connection?
Python Oracle Database Connection is a way of linking your Python application with an Oracle Database. This connection allows your application to interact with the database, performing operations such as selecting, inserting, updating or deleting data. It is a vital skill in the realm of database management and Python programming.
With a Python Oracle Database Connection, you can leverage Python’s powerful programming capabilities to manage and manipulate data stored in an Oracle Database. This adds speed, efficiency, and robustness to your application, whether it’s a small tool to manage your personal books collection or a big software handling thousands of records in a corporate setting.
As a coder, having a diverse toolkit is key to tackling various programming challenges. Learning how to connect Python with an Oracle Database not only allows you to interface with one of the most popular databases, it also opens doors to numerous career opportunities in fields like data analysis, backend development, and database administration. Plus, it’s a chance to see Python, a notably user-friendly programming language, interact with Oracle, offering a playground to test and build your skills.
Setting Up Your Environment
Before we dive into our examples, you need to ensure that your environment is properly set up for Python Oracle Database Connection. This involves installing Python, Oracle Database, and cx_Oracle which is a Python extension for Oracle Database access.
Installing cx_Oracle
To install cx_Oracle, simply use pip:
pip install cx_Oracle
Creating Your Database Connection
Once you have cx_Oracle installed, we can now proceed to write our first example – creating a connection to the Oracle Database with Python.
import cx_Oracle dsn_tns = cx_Oracle.makedsn('YourHost', 'YourPort', service_name='YourServiceName') conn = cx_Oracle.connect(user=r'YourUsername', password='YourPassword', dsn=dsn_tns) c = conn.cursor()
This code initializes a connection to the specified Oracle Database. Replace the respective fields with your actual database details.
Reading Data from Your Database
Now that we’ve connected Python with Oracle Database, let’s use Python to fetch data from the database. Here’s an example:
c.execute('SELECT * FROM YourTable') for row in c: print(row)
This script fetches all rows from “YourTable” and prints them.
Inserting Data into Your Database
You can also use Python to insert data into your Oracle database. Here’s an example:
c.execute("INSERT INTO YourTable VALUES (Value1, Value2, Value3)") conn.commit()
This script inserts a new row with Value1, Value2, and Value3 into “YourTable”. After performing an INSERT operation, remember to commit the transaction with ‘conn.commit()
‘.
Updating Data in Your Database
What if you need to update data in your database? Here’s how you can do it with Python:
c.execute("UPDATE YourTable SET Column1 = 'NewValue' WHERE Column2 = 'OldValue'") conn.commit()
This script updates “Column1” to ‘NewValue’ where “Column2” is ‘OldValue’ in “YourTable”. As with the INSERT operation, ensure you commit the transaction with ‘conn.commit()
‘ after an UPDATE operation.
Deleting Data from Your Database
Python is also used for deleting data from your Oracle Database. Here’s an example:
c.execute("DELETE FROM YourTable WHERE Column1 = 'Value'") conn.commit()
The above script deletes all rows where “Column1” is ‘Value’ from “YourTable”. After performing a DELETE operation, remember to commit the transaction with ‘conn.commit()
‘.
Executing Several Operations in a Transaction
Python Oracle database connectivity allows for executing several operations in a single transaction. Here is an example:
c.execute("INSERT INTO YourTable VALUES (Value1, Value2, Value3)") c.execute("UPDATE YourTable SET Column1 = 'NewValue' WHERE Column2 = 'OldValue'") c.execute("DELETE FROM YourTable WHERE Column1 = 'Value'") conn.commit()
The above script performs an INSERT, UPDATE, and DELETE operation in that order under one transaction which is then committed with ‘conn.commit()
‘.
Handling Errors in Your Database Operations
Python also allows for handling errors during the execution of database operations. Here’s how you can do it:
try: c.execute("UPDATE YourTable SET Column1 = 'NewValue' WHERE Column2 = 'OldValue'") conn.commit() except cx_Oracle.DatabaseError as e: print("There was an error: ", e)
This script attempts to perform an UPDATE operation and if it encounters an error, it prints the error message.
Using Parameterized Queries
Parameterized queries improve the efficiency and security of your database operations. Here’s how you can use them with Python:
params = ('NewValue', 'OldValue') c.execute("UPDATE YourTable SET Column1 = :1 WHERE Column2 = :2", params) conn.commit()
This script uses a parameterized query to perform an UPDATE operation. The parameters are passed as a tuple to the ‘c.execute()’ method.
Closing Your Database Connection
Once your database operations are complete, remember to close the connection:
conn.close()
Closing the connection releases the resources occupied during the connection.
Where to go next?
Now that you’ve learned how to use Python to interact with an Oracle Database, you might be wondering what’s next on your learning journey. This knowledge is a valuable stepping stone, but there are many other interesting and important topics in Python programming to explore.
We wholeheartedly recommend continuing your Python journey by checking out our Python Mini-Degree. This comprehensive course-of-courses covers a variety of Python programming topics beyond databases.
The Python Mini-Degree covers topics like coding basics, algorithms, object-oriented programming, game development, and even app development. You’ll learn in a hands-on manner by creating your own games, algorithms, and real-world apps, including a medical diagnosis bot, a to-do list app, and a quiz app.
Aside from the Mini-Degree, we also have a wide range of individual Python courses that cater to specific areas of interest in our Python Courses gallery. Browse through and discover the multitude of avenues you can venture into with your Python skills.
Conclusion
Python’s connectivity with the Oracle Database opens up a multitude of possibilities. It plunges you deeper into the powerful world of data handling and gives you an edge in the current data-driven ecosystem. Being equipped with this skill puts you in a position to create more dynamic and interactive Python applications and escalate your problem-solving capabilities.
We invite you to join us and explore these opportunities by virtually diving into our Python Mini-Degree. Further expand your Python development skills, embrace the challenge and let’s continue this exciting journey together with Zenva. We can’t wait to see what you’ll create!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
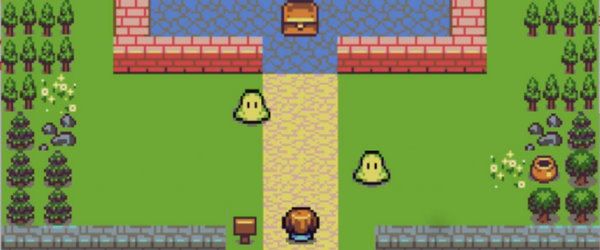
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.