In this enlightening journey, we’ll unravel the intriguing world of Python Object-Oriented Programming. Undeniably, acquiring understanding about this topic is an essential step in becoming a proficient Python programmer or game developer. Whether you are a beginner just starting off or an advanced coder seeking to hone your skills further, join us as we delve into the what, why, and how of these fundamental concepts.
Table of contents
What is Object-Oriented Programming?
Quite often referred to by its acronym OOP, Object-oriented programming is a programming paradigm that revolves around the concept of “objects. Objects are instances of classes, which can contain data in the form attributes (also many times called fields) and also associated behavior in the form of methods (functions defined inside a class).
What is it for?
OOP is majorly used for creating structured and reusable code. It enables developers to:
- Create and handle complex applications with more straightforward logic.
- Reuse code across projects by deploying classes as blueprints for objects.
- Enhance program modularity with OOP principles, leading to code that’s easier to debug, maintain, and develop.
Why You Should Learn Object-Oriented Programming in Python
If you’re learning Python or already have a few scripts under your belt, understanding OOP enhances your programming prowess in numerous ways:
- It is a universal concept: Major languages like Java, C++, and JavaScript use OOP. Thus, fellow developers and employers highly value this skill.
- Real-life representation: OOP concepts like classes and objects permit modeling real-world scenarios effectively in your code. This ability is crucial while developing complex game mechanics or software solutions.
- Pass the interview: OOP knowledge is often tested during coding interviews. Thus, understanding it raises your chances of acing job interviews and securing competitive roles.
In our following sections, we will dive deeper into Python’s object-oriented concepts through exciting code examples! If you’re excited as we are, then the real journey is about to commence.
Getting Started with OOP in Python
Defining a Class
In Python, we define a class using the keyword class. Here’s a simple class named Animal defined in Python:
class Animal: pass
Creating an Object (Instantiation)
Instances of classes (objects) can be created very simply. Below, we create an instance of the Animal class:
my_animal = Animal()
Adding Attributes and Methods to a Class
Attributes are akin to variables belonging to an object, and methods are functions that belong to an object. Let’s expand the above class to have an attribute and a method:
class Animal: sound = "Generic Animal Noise" def make_sound(self): print(self.sound)
Accessing Class Attributes and Methods
Class attributes and methods can be accessed using the dot notation after the instance:
my_animal = Animal() print(my_animal.sound) # Output: Generic Animal Noise my_animal.make_sound() # Output: Generic Animal Noise
Note: The self keyword represents the instance of the class and is used to access class attributes.
The Power of Inheritance
One of the principal advantages of Object-oriented programming is inheritance – where a new class can be derived from an existing class, inheriting its attributes and methods.
Defining a Child Class
Let’s create a Dog class that inherits from the Animal class:
class Dog(Animal): sound = "Woof"
Here, Dog is a child class, and Animal is the parent class.
Overriding Parent Methods
We can also override the parent class methods in the child class. Let’s modify the make_sound method in the Dog class:
class Dog(Animal): sound = "Woof" def make_sound(self): return f"Dogs say {self.sound}"
Now, creating a Dog object and calling make_sound will use the new implementation:
my_dog = Dog() print(my_dog.sound) # Output: Woof print(my_dog.make_sound()) # Output: Dogs say Woof
So far, we have covered the basic steps to get started with Object-oriented programming in Python. Continue to the next part for more advanced concepts.
Advanced Concepts in Python OOP
The flexibility of Python allows for more advanced object-oriented practices, including encapsulation, polymorphism, and special methods to customize class behaviors.
Encapsulation
Encapsulation, one of the fundamental principles of OOP, involves bundling of data (attributes) and the methods that manipulate this data under a single unit, called a class. It also refers to data hiding, where details are hidden from outside and can only be manipulated through methods present in the class.
class Account: def __init__(self, initial_balance): self._balance = initial_balance # private attribute def deposit(self, amount): self._balance += amount def withdraw(self, amount): if amount > self._balance: print("Insufficient balance!") return self._balance -= amount def get_balance(self): # getter method return self._balance
Polymorphism
Polymorphism allows us to use a single interface with different underlying forms. This can be accomplished in Python through method overriding and overloading, and operator overloading.
class Cat(Animal): sound = "Meow" def make_sound(self): return f"Cats say {self.sound}" class Parrot(Animal): sound = "Chirp" def make_sound(self): return f"Parrots say {self.sound}" # Polymorphism in action for animal in Cat(), Parrot(), Dog(): print(animal.make_sound())
Special Methods in Python
Python provides several special or magic methods, like __init__, __str__, and __add__, amongst others, that allow us to perform more sophisticated operations or follow conventions used widely in Python’s ecosystem.
class Vector: def __init__(self, x, y): # constructor method self.x = x self.y = y def __str__(self): # string representation return f"Vector({self.x}, {self.y})" def __add__(self, other): # addition operator overloading return Vector(self.x + other.x, self.y + other.y) v1 = Vector(2, 3) v2 = Vector(4, 5) print(v1 + v2) # Output: Vector(6, 8)
How to Continue Your Python OOP Journey
In this guide, we’ve merely scratched the surface of Object-oriented programming in Python. There exist numerous other concepts, techniques, and best practices for you to discover. However, how do you go about learning more and mastering OOP? We suggest that you keep building projects, create and manipulate classes, inherit from them, and make use of Python’s OOP features.
For structured learning and to really dive deep into Python Programming, we proudly recommend our Python Mini-Degree. This comprehensive collection of courses covers a wide range of essential Python programming concepts like algorithms, game and app development, and of course, the basics of coding with Python. Here you will learn not just theoretically but practically – by creating real-world projects, games, and apps which you can proudly showcase in your Python portfolio.
Our courses at Zenva are:
- Flexible: Accessible 24/7, study at your own pace based on your schedule.
- Comprehensive: Taught by experienced and certified instructors, course material includes video lessons, interactive lessons, coding challenges, quizzes, and printable materials.
- Rewarding: Completion certificates are issued at the end of each course, alongside skills that can help you land a job, publish a game, or even start your own business.
But that’s not all. For a broader collection, you can also explore our wider range of Python programming courses. Whether you are a beginner or an experienced programmer, our courses will bolster your programming prowess.
Conclusion
Unlock the endless opportunities that Python programming with a focus on OOP offers! Whether you aspire to become a game developer, software engineer, or want to delve into the world of algorithms, machine learning, or web development, mastering OOP is a stepping stone you can’t afford to miss. It’s a perfect blend of complex and logical that triggers thought, sparks curiosity, and ultimately leads to a profound understanding of Python.
Join us at Zenva today as we unravel the intriguing aspects of Python Object-Oriented Programming. Our comprehensive Python Mini-Degree offers the ultimate gateway to streamline your Python journey. Let your code speak volumes about your expertise. It’s time to let your ideas come alive with code!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
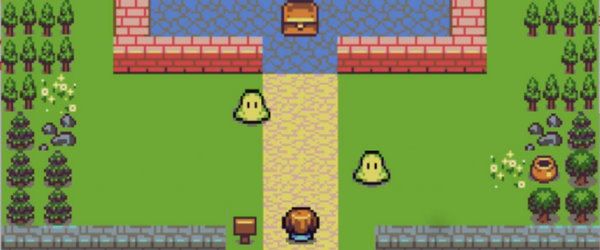
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.