Hello, Coders! As we embark on this exciting journey to explore Python bit functions on integers, you might wonder why this topic is important. Fear not, for by the end of this tutorial, these functions will seem less complex and more like a valuable tool in your Python arsenal.
Table of contents
What are Python Bit Functions?
Python bit functions are special functions that perform operations on integers at bit level. Sounds intimidating? It’s not! At the heart, these functions are just a way to manipulate binary data.
Bit functions are used extensively in network programming, graphics, and gaming where you need to optimize memory usage or perform calculations at the highest speed possible. They allow you to slice and dice integers, extract specific bits, and perform bitwise operations like and, or, not and xor.
Why Should I Learn Bit Functions?
As a Python programmer, learning to use bit functions takes you a step closer to getting the most out of this powerful language. Whether it’s creating a thrilling game, working on data analysis, or networking, bit operations can come to your rescue.
Despite their niche application, the foundational understanding of bit operations gives you insight into how computers work at a fundamental level. They provide a segue from high level programming to a middle ground, increasing your skill set and making you a more versatile coder.
Let’s get down to understanding these functions with some examples. We’ll start with simple operations and gradually move to more complex ones.
Understanding Bitwise AND (&) operation
The bitwise AND operation takes two integer values as input and performs an ‘and’ operation on every pair of corresponding bits of two integers. The operation returns 1 if both bits are 1, else it returns 0.
a = 12 # binary of 12 is 1100 b = 13 # binary of 13 is 1101 c = a & b # binary of c would be 1100 print(c) # result will be 12
Grasping Bitwise OR (|) operation
Similar to AND operation, the bitwise OR operation takes two bit patterns of equal length and performs the logical inclusive OR operation on each pair of corresponding bits. A bit is set (1) if one or both of the bits in the pair is 1. Else, it gets cleared (0).
a = 12 # binary of 12 is 1100 b = 13 # binary of 13 is 1101 c = a | b # binary of c would be 1101 print(c) # result will be 13
Comprehending Bitwise NOT (~) operation
The bitwise NOT operation is a unary operation that flips the bits of the number i.e., if the ith bit is 0, it will change it to 1 and vice versa. It is equivalent to the negative of the number minus one.
a = 12 # binary of 12 is 1100 c = ~a # binary of c would be 0011 print(c) # result will be -13
Deciphering Bitwise XOR (^) operation
The bitwise XOR (exclusive OR) operation compares each bit of its first operand to the corresponding bit of its second operand. If one bit is set (1) and the other bit is not set (0), the corresponding result bit is set to 1. Else, the corresponding result bit is set to 0.
a = 12 # binary of 12 is 1100 b = 13 # binary of 13 is 1101 c = a ^ b # binary of c would be 0001 print(c) # result will be 1
Unpacking Bitwise shift operators
The bitwise shift operators are the shift right operator (>>) and the shift left operator (<<). They move the bit value either to the right or the left.
Understanding the Bitwise Shift Right (>>) operation
This operator shifts the bits of the number to the right and fills the vacant bit on the left with a zero. The left operand specifies the value to be shifted and the right operand specifies the number of positions that the value is to be moved.
a = 12 # binary of 12 is 1100 c = a >> 2 # binary of c would be 0011 print(c) # result will be 3
Grasping the Bitwise Shift Left (<<) Operator
This operator shifts the bits of the number to the left and fills the space on the right with zeros. The left operand specifies the value to be shifted and the right operand specifies the number of positions that the value is to be moved.
a = 12 # binary of 12 is 1100 c = a << 2 # binary of c would be 110000 print(c) # result will be 48
Delving into the Bitwise Ones Complement Operator (~)
The unary bitwise complement operator (~) inverts all the bit pattern i.e., changes all 1s to 0s and all 0s to 1s.
a = 12 # binary of 12 is 1100 c = ~a # binary of c would be 0011 print(c) # result will be -13
Mastering Bitwise AND assignment operator (&=)
Used to combined AND operator with assignment operator. It performs a bitwise AND operation between the two operands and then assigns the result to the left operand.
a = 12 # binary of 12 is 1100 b = 13 # binary of 13 is 1101 a &= b # print(a) # result will be 12
Deciphering Bitwise OR assignment operator (|=)
This operator has similar function to AND assignment operator, but it does the bitwise OR operation.
a = 12 # binary of 12 is 1100 b = 13 # binary of 13 is 1101 a |= b # print(a) # result will be 13
Where to go next
Having unlocked the mysterious realm of Python bit functions, you might be eager to continue on your coding journey. The key to mastering any programming language, including Python, is consistency, practice, and continuous learning.
At Zenva Academy, we want to make that learning trek more accessible and engaging. We encourage you to explore our comprehensive Python Mini-Degree. This Mini-Degree offers an exhaustive array of Python courses spanning from programming basics, to algorithms, object-oriented programming, and even game and app development.
You will learn by creating your own games, writing efficient algorithms, and building real-world apps. The best part? These courses are designed for learners of all levels. Whether you are just starting out or you’re a seasoned coder looking to deepen your Python knowledge, we have got you covered.
What’s more, our courses do not stop at just Python programming. We recommend checking out our broader collection of Python courses to explore various areas of this versatile language.
Conclusion
In conclusion, Python bit functions do not have to be intimidating anymore. They are a powerful tool that, when understood and utilised, can lead to more efficient code and a deeper understanding of how your programs work under the hood.
Remember, the path to becoming a seasoned coder is paved with constant learning and practice. At Zenva, we are committed to making that journey easier and more engaging. With our extensive Python Mini-Degree, we’ve got you covered. Jump in today and harness the full power of Python!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
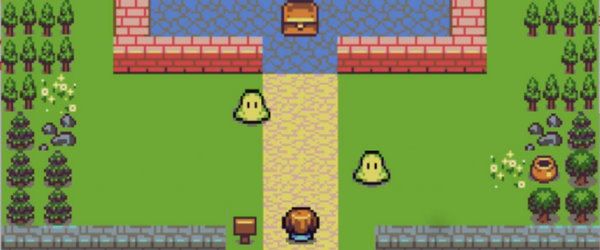
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.