If you’ve embarked on the journey to uncover the secrets of Python, you’ve probably seen or written code where variables of different types interact with each other. A number gets added to a string, a list interacts with a boolean, or a dictionary with an integer. But how does Python process such mixed interactions? The answer: Type Conversion.
Table of contents
What is Type Conversion?
Type Conversion, also known as Type Casting, is a method through which we can alter the data type of a variable. Python, being a dynamically-typed language, often implicitly converts one data type to another if needed. However, there are times when we need to perform this conversion manually to achieve the desired results in our code.
Understanding how to convert data types in Python can help us handle a plethora of potential issues ahead of time. It also aids in making our code cleaner, more readable, and more efficient. If you’re creating a game, for instance, where the player’s score is calculated or stored as a string, you’d need to convert that score to an integer if you want to perform any numerical operations on it. If not done correctly, this could cause unexpected bugs in your code.
What Can It Be Used For?
Python Type Conversion can be used to prevent type errors, which can often be a bane for programmers. For example, say you’re working on a game and you’re trying to calculate the total score. You’ve got the score as a string from user input and the bonus points as an integer. If you try to add them up without converting the score to an integer, Python will throw a TypeError. This is where the practice of Type Conversion comes in handy.
Also, Type Conversion can aid in data analysis and manipulation. Sometimes, raw data is obtained in one certain type while another data type might be needed for processing. This is commonly seen when working with APIs, data databases, or even user inputs.
In the next sections, we’re going to dive into the actual workings of Type Conversion in Python, featuring elementary code examples. We will also show you a way to keep advancing your Python skills with us at Zenva Academy. So, buckle up and get ready for an exciting ride into the world of Python Type Conversion!
Type Conversion in Python – Implicit and Explicit
As mentioned before, Python performs two types of Type Conversion – Implicit and Explicit. Let’s dive deeper, starting with Implicit Type Conversion.
Implicit Type Conversion
In Implicit Type Conversion, Python automatically converts one data type to another without the programmer’s involvement. Here’s an example:
x = 10 y = 1.5 z = x + y print(z) print(type(z))
In the code snippet above, Python automatically converts integer x to a float and adds it to y. The type() function shows that the result z is a float.
Explicit Type Conversion
Explicit Type Conversion, as the name suggests, requires the programmer to convert the data type. Python provides built-in functions for performing explicit type conversion, which include int(), float(), str(), etc. Let’s take a look at some examples:
# Converting float to int x = 10.5 y = int(x) print(y) print(type(y)) # Converting int to string x = 10 y = str(x) print(y) print(type(y)) # Converting string to float x = '10.6' y = float(x) print(y) print(type(y))
In each example, the respective function is used to convert one data type to another.
Error Handling in Type Conversion
Sometimes, Type Conversion may not work as expected. For instance, trying to convert a non-numeric string to an integer or a float would raise a ValueError. Hence, it’s critical to handle such possibilities.
# Trying to convert non-numeric string to int x = 'hello' try: y = int(x) print(y) except ValueError: print("Cannot convert non-numeric string to integer.")
The use of the try-except block in the above code ensures the error is caught and handled gracefully. The program doesn’t crash and the error message is meaningful.
Through these examples, you can see that Type Conversion in Python is a fundamental skill that every programmer should master for efficient coding. As we continue to explore the world of Python on our learning journey at Zenva Academy, we encourage you to practise and perfect your understanding of type conversion. It’s a small concept but vastly significant in the long run.
More on Explicit Type Conversion
Let’s delve deeper into explicit conversion and understand how to use additional built-in Python functions for type casting.
Converting to List
The list() function can convert certain types of data to a list.
# Converting string to list x = 'zenva' y = list(x) print(y) # Converting tuple to list x = ('coding', 'gaming', 'learning') y = list(x) print(y)
Converting to Tuple
The tuple() function can convert certain types of data to a tuple.
# Converting string to tuple x = 'academy' y = tuple(x) print(y) # Converting list to tuple x = ['python', 'java', 'c++'] y = tuple(x) print(y)
Converting to Set
The set() function can convert certain types of data to a set. A set is an unordered collection of unique elements.
# Converting string to set x = '
y = set(x) print(y) # Converting list to set x = ['coding', 'gaming', 'learning', 'coding'] y = set(x) print(y)
Converting to Dictionary
The dict() function can convert a sequence of tuples (each containing a key value pair) to a dictionary.
# Converting list of tuples to dictionary x = [('python', 1), ('java', 2), ('c++', 3)] y = dict(x) print(y)
As we can see, Python provides us with a suite of straightforward functions to convert between various data types. Understanding these functions is an essential step to becoming proficient in Python, and writing clean, efficient code. Here at Zenva Academy, we will continue to provide examples like these to help you deepen your understanding of Python.
Next Steps in Your Python Journey
Now that you have a good understanding of Type Conversion in Python and why it’s important, you might be wondering, what’s next?
Don’t worry, at Zenva Academy, we have got your back. We are committed to supporting learners on their journey from beginner to professional coder. Our Python Mini-Degree is a solid next step on your Python learning path.
Our Python Mini-Degree is a comprehensive collection of courses focused on Python—a language celebrated for its simplicity and versatility. It covers a wide range of topics from coding basics and algorithms, to object-oriented programming, game development, and app development. No matter your experience level, you will benefit from these courses. Beginners will gain a strong foundation, while more experienced coders will refine their Python skills and discover new approaches.
One of the highlights of our courses is that learning Python does not stay confined to theory. You will get hands-on experience by creating real-world apps, games, and algorithms. Each project can be added to your Python portfolio—proving to others (and yourself) that you know your stuff!
Apart from our Python Mini-Degree, we offer a broader collection of Python courses. By exploring our Python courses page, you can find more specialized topics to delve into, strengthening your Python acumen even further.
Conclusion
There you have it – a deep dive into the world of Type Conversion in Python. As we’ve seen, understanding this fundamental concept is crucial in writing clean, efficient, and error-free code. It’s a stepping stone towards mastering this versatile and in-demand language.
Your learning adventure mustn’t stop here. With our Python Mini-Degree, you can keep your Python skills evolving, always staying aligned with industry trends. Remember, in the world of coding, every piece of knowledge is a tool that empowers you. So, keep learning and keep coding, dear fellow coders, for a rewarding journey lies ahead with Zenva Academy.
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
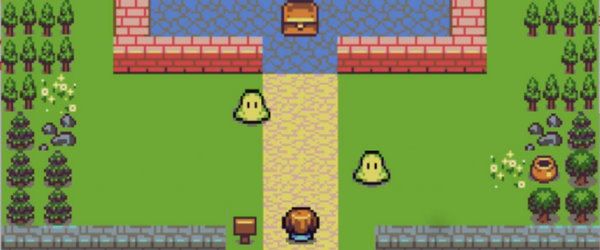
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.