Get ready to embark on a fantastic journey into the world of Lua and its application in object-oriented programming (OOP). Many of you might be familiar with other object-oriented programming languages like C++ or Python, but if you’re an aspiring game developer, Lua will be an essential tool in your kit.
Table of contents
What is Lua Object-Oriented Programming
Lua is a lightweight, high-level scripting language often used in the game development industry. Unlike traditional OOP languages where the object-oriented principles are hard-coded into the language’s design, Lua allows for much more flexibility by using “tables” to create objects, and “meta-tables” to create classes.
Why Should I Learn Lua OOP?
Lua is particularly popular in the field of game development, powering some of the world’s leading platforms like Roblox. The incorporation of robust and creative features makes it a favorite among game developers. The object-oriented nature of Lua gives developers more control over their code by allowing them to encapsulate data within objects, making for cleaner, more organized code.
Mastering Lua will not only add a new dimension to your coding skillset, but it will also open new opportunities in the thriving game development landscape. Let’s dive deep into this fascinating aspect of Lua programming!
Basic Syntax of Lua OOP
Our first order of business is understanding the basics of Lua’s Object-Oriented Programming. Unlike languages like C++ or Java, Lua does not have built-in syntax for classes and objects. Instead, we use tables and metatables to simulate this functionality.
-- defining a table myTable = {} myTable.x = 10 myTable.y = 20
In the above example, we define a table named ‘myTable’ and add properties ‘x’ and ‘y’ to it. This table can be thought of as an object in OOP, with ‘x’ and ‘y’ being its properties.
Creating Classes using Metatables in Lua
Next, we will create a ‘class’ in Lua. To do this, we will use metatables. Metatables allow us to attach ‘meta-methods’ to a table that define certain behaviors, such as what happens when you try to access a table’s non-existing field.
-- Creating a class Vector2 = {} Vector2.__index = Vector2 function Vector2.new(x, y) local self = setmetatable({}, Vector2) self.x = x self.y = y return self end
In this code, we are defining a ‘Vector2’ class. Through the setmetatable function, we are making it so that all instances of this ‘class’ will look towards the ‘Vector2’ table when they are attempting to access a field that they do not possess.
Methods in Lua OOP
Just like in any OOP language, our objects can have methods. Let’s add a method to our ‘Vector2’ class that will calculate the magnitude of the vector.
function Vector2:magnitude() return math.sqrt(self.x * self.x + self.y * self.y) end
We have now added a function that acts as a method of the ‘Vector2’ class. An important thing to note here is the use of the colon ‘:’ instead of the dot ‘.’ operator. This allows ‘self’ to be implicitly passed to the method.
Instantiation and Method Calling in Lua OOP
Now that we have created our class and added a method to it, we can create an instance of ‘Vector2’ and call its method.
-- Create an instance local vec = Vector2.new(3, 4) -- Call a method print(vec:magnitude()) --prints 5
This example illustrates how we create an instance of the ‘Vector2’ class and call its ‘magnitude’ method. The result of 5 (the hypotenuse of a 3-4-5 triangle) proves that our method is working perfectly.
By mastering these basics, you’ve taken your first step into the world of Lua Object-Oriented Programming. Stay tuned for the next part of this series where we’ll dive deeper into the intricacies of Lua OOP!</p
More Power with Functions in Lua OOP
Moving forward, let’s harness the power of Lua functions in OOP to execute more complex operations.
Adding and Overriding Methods in Lua
In Lua, we can add more methods to our classes even after their declaration. Similarly, we can just as easily override them whenever necessary.
-- Adding a new method function Vector2:add(vector) self.x = self.x + vector.x self.y = self.y + vector.y end -- Overriding the 'magnitude' method function Vector2:magnitude() return math.abs(self.x) + math.abs(self.y) end
In this example, we’ve added a new method ‘add’ to our Vector2 class that adds the x and y values of the calling vector to those of the passed vector. We’ve also overridden our ‘magnitude’ method to return the sum of the absolute values of ‘x’ and ‘y’ instead of their root.
local vec1 = Vector2.new(10, 20) local vec2 = Vector2.new(30, 40) vec1:add(vec2) print(vec1.x) --prints 40 print(vec1.y) --prints 60 print(vec1:magnitude()) --prints 100
We can see the influence of our new ‘add’ method and the overridden ‘magnitude’ in the above code.
Inheritance in Lua
Like any other OOP language, Lua supports inheritance, whereby we can create new classes based on existing ones. This can significantly speed up development and ensure a cleaner organization of code.
-- Creating a new class based on 'Vector2' Vector3 = setmetatable({}, Vector2) Vector3.__index = Vector3 function Vector3.new(x, y, z) local self = setmetatable(Vector2.new(x, y), Vector3) self.z = z or 0 return self end -- Overriding the 'magnitude' method for 'Vector3' function Vector3:magnitude() return math.sqrt(self.x * self.x + self.y * self.y + self.z * self.z) end
We have successfully established a new class ‘Vector3’, based on ‘Vector2’. Our new class also has an additional dimension ‘z’. Afterward, we override the ‘magnitude’ method for ‘Vector3’ to incorporate the ‘z’ value.
local vec3 = Vector3.new(3, 4, 12) print(vec3:magnitude()) --prints 13
We can ensure that our overridden ‘magnitude’ method is functioning correctly by making a ‘z’ value equal to 12 (forming a 5-12-13 Pythagorean triple).
Through this deep dive into Lua’s OOP syntax, we have illustrated the flexibility and power of Lua. It adapts to various OOP concepts effectively, providing a clean and organized framework for game development. Stay tuned for our next tutorials to learn more about this exciting language and enhance your coding skills!
Polymorphism and Metamethods in Lua
Polymorphism is a foundational element of OOP that allows a method to perform different things based on its input. Metamethods in Lua are a way to implement polymorphism. They allow us to define what happens when an operation is performed on a table.
-- Defining the '__add' metamethod on Vector2 Vector2.__add = function(v1, v2) return Vector2.new(v1.x + v2.x, v1.y + v2.y) end
Here, we have added the ‘__add’ metamethod to our ‘Vector2’ class. This metamethod gets called when we use the ‘+’ operator on instances of the class.
local vec1 = Vector2.new(10, 20) local vec2 = Vector2.new(30, 40) local sum = vec1 + vec2 print(sum.x) --prints 40 print(sum.y) --prints 60
The ‘__add’ metamethod is working correctly, as we can see from the sum of ‘vec1’ and ‘vec2’.
Modifying Existing Metamethods
We can further modify our ‘__add’ metamethod to accept both ‘Vector2’ and ‘Vector3’ instances, and return a ‘Vector3’ instance in the latter case.
Vector2.__add = function(v1, v2) if (getmetatable(v2) == Vector3) then return Vector3.new(v1.x + v2.x, v1.y + v2.y, v2.z) else return Vector2.new(v1.x + v2.x, v1.y + v2.y) end end
This code checks the metatable of ‘v2’ and returns a ‘Vector3’ instance if it matches the ‘Vector3’ metatable. Otherwise, it returns a ‘Vector2’ instance.
local vec1 = Vector2.new(10, 20) local vec3 = Vector3.new(30, 40, 50) local sum = vec1 + vec3 print(sum.x) --prints 40 print(sum.y) --prints 60 print(sum.z) --prints 50
The modified ‘__add’ metamethod successfully returns a ‘Vector3’ instance when adding a ‘Vector2’ instance to a ‘Vector3’ instance.
Conclusion
Throughout these examples, we’ve showcased the power, flexibility, and potential of Lua’s object-oriented features. By leveraging tables, metatables, and their associated metaprogramming methods, we can design and implement complex object-oriented patterns that are clear, extensible, and efficient. By honing your Lua skills with us, you are well on your way to becoming proficient in game development, scripting and more. Stay tuned for advanced topics and keep coding!
Keep Learning and Enhance Your Skills
Now you’ve gotten started and dipped your toes into the vast sea of Lua Object-Oriented programming, the next logical question is – where to go next?
The answer is simple – continue to sharpen your knowledge and skills with us here at Zenva. As the leading online learning platform for programming, game development, and AI, we offer a catalogue of over 250 courses suitable for everyone, from beginners to professionals. Our courses help you learn coding, create games, and earn certificates. This means that even after you’ve mastered the basics, Zenva has more advanced content to fuel your learning journey.
For those who are keen on applying their Lua OOP learning to game development, our comprehensive Roblox Game Development Mini-Degree is the perfect next step. This collection of guided online courses will allow you to create different genres of games using Roblox Studio and Lua, including obstacle courses, melee combat games, and FPS games. By learning game development with Roblox, you can tap into the multi-billion-dollar game market. Alternatively, explore more offerings in our Roblox collection to find topics of your interest.
Regardless of where your learning journey takes you, always remember that each step you take brings you closer to mastery. The world of game development and coding awaits you. Happy learning!
Conclusion
Your journey into the world of Lua Object-Oriented Programming has just begun, and there is so much more to explore and learn. Remember, with each new concept you understand and implement, you’re not only enhancing your coding skills, but also opening yourself to a universe of opportunities in the world of game development, scripting, and beyond.
Learning is a never-ending journey, and we at Zenva are committed to walking this path with you. From basics to advanced concepts, our comprehensive courses have everything covered. TheRoblox Game Development Mini-Degree is a perfect learning path that can take your Lua programming to the next level. Keep moving forward in your journey and continue creating, exploring, and pushing the limits of what’s possible. Happy coding!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
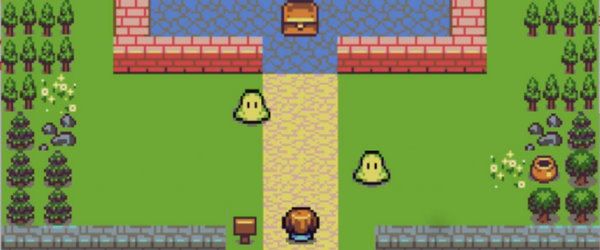
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.