Welcome to this tutorial on Lua encapsulation! This programming powerhouse may sound complex, but once you’ve dived in and discovered its potential, you’ll quickly see why it’s such a valuable tool in the coding world.
Table of contents
What is Lua Encapsulation?
Lua encapsulation is a significant aspect of object-oriented programming (OOP) in the Lua language. Encapsulation is a way to bundle the data (variables) and the methods (functions) together into single units called ‘objects.’
What is it Used For?
Encapsulation is used to hide the values or state of an object from any other code. This prevent the data from being directly modified and keeps it safe and secure. In game development, this can be incredibly useful in managing interactions between different elements within your game.
Why Should I Learn It?
Understanding Lua encapsulation allows you to design and build interactive elements that operate independently from each other, enhancing your game’s complexity and dynamism. This level of control is a key element to truly master as you continue your journey in Lua coding and game creation.
Whether you’re a novice coder or a seasoned developer looking to further enhance your skills, realizing the potential of Lua Encapsulation will add valuable tools to your coder’s toolkit. Let’s dive into some practical examples and fully understand the concept!
Creating a Basic Object in Lua
We’ll start with the basics of creating an object in Lua. Here’s how to create an object with properties.
Player = {name = "John", health = 100, speed = 10} print(Player.name) print(Player.health) print(Player.speed)
In the above code, we’ve created a Player object with the properties name, health, and speed. When we print these properties, it will output “John”, 100, and 10 respectively.
Adding Methods to Our Object
An object can also contain methods, which are functions that can access and modify the data. Here’s how you can add a method to an object in Lua.
Player = { name = "John", health = 100, speed = 10, takeDamage = function(self, damage) self.health = self.health - damage end } Player:takeDamage(20) print(Player.health)
In the above example, we added a takeDamage method that reduces the Player’s health by a given amount. After taking 20 damage, the Player’s health drops to 80.
Encapsulating Our Object
Encapsulation in Lua usually involves creating a table as a class with local variables and functions and returning the object. In Lua, the keyword local is used to declare a local variable. This local variable is only visible within the block where it is declared, protecting it from outside access.
local Player = {} Player.__index = Player function Player:new(name, health, speed) local player_instance = {name = name, health = health, speed = speed} setmetatable(player_instance, Player) return player_instance end player1 = Player:new("John", 100, 10) print(player1.name) print(player1.health) print(player1.speed)
In the above code, we have encapsulated our variables and our object creation process inside a function of our new class, Player. The function Player:new() returns a new object of the class type Player and sets the internal variable __index to the Player table which allows instances to look up methods not found in their own tables.
Adding Encapsulated Methods
Next, we will add encapsulated methods. This allows us to develop functions that operate securely within the object and can only be accessed in the context of the object.
function Player:takeDamage(damage) self.health = self.health - damage end player1:takeDamage(20) print(player1.health) -- prints 80
Here, we have added an encapsulated method, takeDamage, to our Player class, which modifies its instance’s health attribute.
Access Control with Encapsulation
By using the local keyword, we can also have control over the access to our attributes and methods, providing a finer level of detail in our encapsulation.
local Player = {} Player.__index = Player local function calculateActualDamage(damage) return damage - 0.1 * damage end function Player:new(name, health, speed) local player_instance = {name = name, health = health, speed = speed} setmetatable(player_instance, Player) return player_instance end function Player:takeDamage(damage) self.health = self.health - calculateActualDamage(damage) end player1 = Player:new("John", 100, 10) player1:takeDamage(20) print(player1.health) -- prints 82
Here, we have used a local function calculateActualDamage which is only accessible inside our Player class. This function modifies the damage amount and we use it inside the takeDamage function.
Inheritance in Encapsulation
Another powerful aspect of encapsulation is ‘Inheritance’, where one class can adopt the properties and methods of another. This is done by calling the superclass’s constructor within the derived class.
Enemy = Player:new("Enemy", 50, 5) print(Enemy.name) -- prints "Enemy" print(Enemy.health) -- prints 50 print(Enemy.speed) -- prints 5
Here, the Enemy class is a derived class inherited from the superclass Player. So it consists all the properties and methods of the Player class. The ‘:new()’ constructor is used to initialize a Enemy instance with the class’ name, health, and speed.
We hope you’ve found this deep dive into Lua encapsulation to be informative and useful on your coding journey. Remember, encapsulation is essential not just for code security, but also for effectively structuring your code and maintaining its integrity as you build complex game interactions. Happy coding!
Overriding Methods in the Derived Class
If needed, we can override inherited methods in derived classes too. Let’s override the takeDamage method in our Enemy class.
function Enemy:takeDamage(damage) self.health = self.health - damage print("An enemy took damage!") end Enemy:takeDamage(20) print(Enemy.health) -- prints 30
In this example, we have written a specialized takeDamage method for the Enemy class. Now, when we invoke the takeDamage method on an Enemy object, it will use this specialized function instead of the inherited one. Notably, it also prints “An enemy took damage!” when invoked.
Use of self in Lua Encapsulation
In encapsulation, ‘self’ is a special variable that refers to the instance that is executing the current method. This gives us a way to access the instance’s variables and is crucial for encapsulation in Lua.
function Player:getHealth() return self.health end print(player1:getHealth()) -- prints 82
This Player method, getHealth, when called on an instance, refers to the self (the instance) to return its health.
Special Class Methods
Lua also has special class methods like __tostring() that can be overridden for your classes. This could be very beneficial for debugging.
function Player:__tostring() return self.name.."'s current health: "..self.health end print(player1) -- prints "John's current health: 82"
Here, we overrode the default __tostring() method from Lua, so whenever we print an instance of Player, it will give information in a more human-readable format.
Encapsulation for Game Properties
Encapsulation is not just for players and enemies but also for game properties such as levels and score.
local Game = {level = 1, score = 0} function Game:nextLevel() self.level = self.level + 1 end function Game:addScore(points) self.score = self.score + points end Game:nextLevel() Game:addScore(100) print(Game.level) -- prints 2 print(Game.score) -- prints 100
In this last example, we created a Game object with encapsulated properties level and score, showing how encapsulation helps us manage game properties efficiently and securely.
With these detailed examples and concepts, you are now equipped with the knowledge to use encapsulation effectively in your Lua game projects. Always remember the fundamentals of encapsulation – it separates code implementation from code use, which not only secures our data but also makes our code more manageable and flexible. Happy gaming!
Where to go next
With the fundamentals of Lua encapsulation now under your belt, it’s time to continue expanding your knowledge and hone your skills. A fantastic way to do so is by diving into our extensive catalog of supportive materials and courses.
In particular, you might find our Roblox Game Development Mini-Degree to be a rewarding next step. These comprehensive courses cover game creation with Roblox Studio and Lua across various game genres. From level creation and scripting to diving deeper into multiplayer mechanics and leaderboards, this Mini-Degree helps you build foundational and advanced skills in game development. Turning your passion for gaming into a career might be closer than you think!
For more targeted learning, check out our encompassing selection of Roblox courses. Whether you are a beginner or an experienced developer, there’s always something new to discover and learn at Zenva Academy. Your coding adventure has just begun – let’s keep the momentum going!
Conclusion
The understanding and effective use of encapsulation in Lua can be a game-changer in your coding journey. It adds an extra layer of security and efficiency, making your game development more dynamic and interactive. This has been a beginner’s guide, and there’s so much more to unearth about Lua and game development.
We invite you to dive deeper into the ocean of coding with us at Zenva Academy. Choose from our wide variety of courses and step forward in your learning adventure today. With Zenva, every coder has the potential to become a game creator. Are you ready?
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
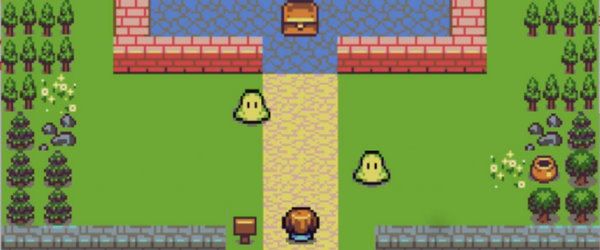
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.