Welcome to this comprehensive tutorial on Lua coding. Over the next few sections, we will delve into the fundamental mechanics of Lua, a powerful yet straightforward programming language widely used in game development and web applications. Our tutorial aims to engage you with multiple hands-on code examples and analogies tied around popular game mechanics, creating a wonderful learning experience. For seasoned coders looking for a refresher and beginners eager to dive into the world of coding, this tutorial is the gateway to enhancing your coding skills.
Table of contents
What is Lua?
Lua is a high-level, multitasking scripting language used to extend applications. It’s largely known for its simplicity, efficiency, portability, flexibility, and free availability, making it a popular choice among game developers and app programmers alike.
What is it Used For?
Lua serves a variety of purposes due to its expansive functionality, however, it is especially prominent in the realm of game development and design, where game mechanics can be controlled and altered programmatically using Lua. Other applications include web scripting, Image processing and artificial intelligence.
Why Should I Learn Lua?
Learning Lua offers a multitude of advantages. Lua is highly portable and runs on just about any platform you can think of, providing a wide scope for practical application. Its integration with C means you can use Lua for high-level programming while relying on C for low-level functions. The rise of cloud computing also means that Lua is growing in demand, so knowing Lua can prove advantageous for your career aspirations in the tech domain. Rest assured, by investing time to learn Lua, you are setting yourself up for a future filled with opportunities in the rapidly evolving tech world.
Getting Started with Lua
Before diving into the coding examples, it’s necessary to have Lua installed in your system. It’s a straightforward task and can be accomplished by visiting the official Lua website and following the installation instructions.
Now let’s tap into the world of Lua with some basic coding examples.
1. Hello World in Lua
In Lua, printing a phrase or sentence is as simple as utilizing the print
function. Here’s how you can display the classic “Hello, World!” in Lua:
print("Hello, World!")
The following code displays the string “Hello, World!” when run.
2. Variables and Data Types in Lua
In Lua, there are eight basic types: nil, boolean, number, string, userdata, function, thread, and table. Let’s take a look at a few examples:
local name = "John" -- This is a string local age = 30 -- This is a number local isAdult = true -- This is a boolean print("Hello " .. name .. ", age " .. age) print("Is John an adult?", isAdult)
The “..” operator is used to concatenate strings in Lua.
3. Working with Tables in Lua
Tables are the only complex data structures in Lua. They function like arrays and are capable of storing multiple value types.
-- Create a new table local fruits = {"Apple", "Banana", "Cherry"} -- Add a new element to the table table.insert(fruits, "Orange") -- Remove the first element table.remove(fruits, 1)
The table.insert method is used to add a new element while table.remove helps in removing an existing element.
4. Conditional Statements in Lua
Lua, like other languages, use conditional statements to perform different computations or actions depending upon whether a programmer-specified condition evaluates to true or false.
local age = 20 if age >= 18 then print("You are an adult.") else print("You are a minor.") end
The if-else statement checks whether the age is more than or equal to 18. If it’s true, “You are an adult” gets printed, otherwise “You are a minor” is printed.
Working With Functions
Functions are a core part of Lua’s functionality. A function is a group of instructions that perform a particular task. You can define your own functions in Lua.
function greet(name) print("Hello " .. name) end greet("John")
In the given example, we define a function named ‘greet’ which takes one argument ‘name’. We then call this function with the argument “John”.
More Complex Lua Functions
Lua supports first-class functions, meaning that functions can be stored in variables, tables, and can be passed as arguments to other functions.
Let’s deep dive further into Lua’s function versatility.
First, how about a function performing addition?
function add(a, b) return a + b end print(add(2,4)) -- Prints 6
Next, let’s look at a function that calculates the factorial of a number.
function factorial(x) if (x == 0) then return 1 else return x * factorial(x - 1) end end print(factorial(5)) -- Prints 120
In this example, the factorial of a number is calculated using recursion, i.e., when a function calls itself.
Table iterations in Lua
Finally, let’s examine how Lua can iterate over tables, similar to other popular languages and arrays.
local fruits = {"Apple", "Banana", "Cherry"} for k, v in pairs (fruits) do print("I love " .. v) end
The for-loop uses a built-in function, pairs() which returns a next function, the table t, and nil. The next function, when called with nil, returns the first index and the associated value.
As you can see, Lua is an integral part of the coding and game development realm. It offers a great balance between ease of use for beginners and the power needed for advanced coders.
Now it’s your turn to put the knowledge gained in this tutorial into practice. Challenge yourself with some Lua-based exercises, and very soon, you’ll be creating games and applications with the best in the industry.
Looping in Lua
The loop statements in Lua are used when a block of code needs to be executed several times. Lua provides several types of loops to handle looping requirements, including ‘for‘, ‘while’, and ‘repeat’ loop.
For Loop:
for i = 1, 10, 1 do print(i) end
The above code snippet illustrates a ‘for’ loop. The loop will iterate 10 times, and with each iteration, it prints the current value of ‘i’.
While Loop:
local i = 1 while(i <= 10) do print(i) i = i + 1 end
This is an example of a while loop in Lua. We initialize ‘i’ with 1 and then, the while loop continues until ‘i’ is less than or equal to 10.
Error Handling in Lua
Lua provides a mechanism to handle errors that arise in a program. This is done using the pcall (protected call) function.
function divide(num1, num2) if (num2 == 0) then error("Can't divide by zero") else return num1/num2 end end status, value = pcall(function() divide(10, 0) end) if (status) then print("Result is " .. value) else print("Error: " .. value) end
In this example, pcall is used to handle the error caused by attempting to divide by zero. If the division is valid, it prints the result; if an error is detected, it outputs the error message.
Lua Tables as Associative Arrays
While Lua’s table can work as an array, it’s also functional as an associative array. Associative arrays are an abstract data type composed of a collection of key-value pairs, such that each possible key appears just once in the collection.
local person = { name = "John", age = 30, isAdult = true } print(person.name .. " is " .. person.age .. " years old.")
In this particular snippet, a table named ‘person’ has multiple entries. Each key is associated with a value which describes the ‘person’.
Where to Go Next
After grasping the basics of Lua from this tutorial, why not put your newfound skills to the test? Your Lua journey has only begun. At Zenva, we believe that you can do anything, provided you are equipped with the right knowledge and know-how.
We highly encourage checking out our Roblox Game Development Mini-Degree. This comprehensive collection of courses on creating games with Roblox Studio and Lua could be the perfect next step. Regardless of your experience level, you’ll find opportunities in the multi-billion-dollar gaming market.
You could also explore our broader collection of courses on Roblox, crafted to cater to anyone who has already learned the basics of Lua. With Zenva, a beginner’s fascination can be transformed into a professional’s arsenal. So why wait? Let’s start building your coding expertise today.
Conclusion
Wow, look at how far you’ve come! You’ve taken your first steps into the vastly interesting realm of Lua, a language that holds much promise and potential in today’s ever-evolving technological landscape. Whether it’s for game development or web applications, Lua’s simplicity backed by its powerful capabilities can propel you to create works of originality and impressed functionality.
So, ready to kickstart your Lua journey with us? Let’s master Lua together with our comprehensive Roblox Game Development Mini-Degree. Remember, every great coder starts by taking that first tiny step. With Zenva, that first step has the potential to become a giant leap. Embark on your coding journey today – we promise, you won’t look back!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
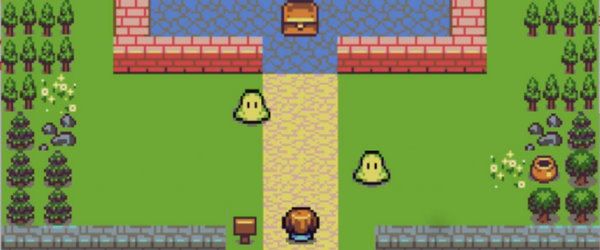
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.