In today’s digital world, the relevance of coding and programming is more prevalent than ever. Whether you’re new to the world of programming, an experienced coder looking to add another language to your arsenal, or a game enthusiast eager to create your own game dynamics, C++ is a language worth exploring.
Table of contents
What is C++?
C++ is a general-purpose programming language that was developed as an extension of the C language. Known for its efficiency and flexibility, C++ allows programmers to manage memory and system resources directly, making it an excellent choice for game development.
Why Learn C++?
Learning C++ opens doors to numerous opportunities. Here’s why you should consider learning C++:
- One of the most powerful languages: C++ provides a high degree of control over system resources and memory, a critical requirement for games or resource-intensive apps.
- Sought-after skillset: From video game design to system/application software, the versatility of C++ makes it highly sought-after in the job market.
- Strong foundation: Learning C++ provides a solid understanding of programming at a lower level, making it easier to learn other languages later.
Now, let’s dive deeper into coding with C++.
Getting Started with C++
The first step is always installing the right tools. For C++, you will need an Integrated Development Environment (IDE) like Code::Blocks or Visual Studio. Once installed, you can start writing your first C++ program.
#include using namespace std; int main() { cout << "Hello, World!"; return 0; }
This is a simple C++ code that will output ‘Hello, World!’ onto your screen.
Variables
Variables in C++ are easy to declare, they are containers for storing data values.
int myVar = 5; double myDoubleVar = 5.99; char myLetter = 'D'; string myText = "Hello World"; bool myBoolean = true;
This code snippet demonstrates five distinct variable types: integer numbers, double (fractional) numbers, characters, text strings, and boolean values.
Operators
As in other programming languages, we can perform basic arithmetic operations in C++.
int x = 5; int y = 10; int sum = x + y; cout << sum;
This code initiates two integer variables, x and y, and adds them together. The sum is then displayed.
If…Else
We can use If…Else statements in C++ to control program flow based on certain conditions.
int time = 22; if (time < 10) { cout << "Good morning."; } else if (time < 20) { cout << "Good day."; } else { cout << "Good evening."; }
The above snippet checks if the variable ‘time’ value is less than 10, between 10 and 20, or greater than 20, and outputs a corresponding message.
It’s important to remember that practice is key to mastering any programming language, C++ included. Regular coding exercises will not only help you understand the language better but also improve your problem-solving skills.
Loops in C++
Loops are crucial elements in any programming language, allowing you to execute a block of code multiple times. C++ offers several loop types, let’s explore a few.
While Loop continues until a specified condition is met:
int i = 0; while (i < 5) { cout << i << "\n"; i++; }
For Loop, commonly used for iterating over a range:
for (int i = 0; i < 5; i++) { cout << i << "\n"; }
Do/While Loop, similar to the while loop, but the condition is tested after executing the statements, guaranteeing the loop gets executed at least once:
int i = 0; do { cout << i << "\n"; i++; } while (i < 5);
Functions in C++
Functions are blocks of code designed to perform a particular task. A C++ function is defined like this:
void myFunction() { cout << "Hello, World!"; } int main() { myFunction(); return 0; }
Here, “void” means the function doesn’t return any value. Functions can also return values and take parameters.
Arrays in C++
An array in C++ is used to store multiple values in one single variable. The array can be declared as follows:
int myArray[3] = {10, 20, 30};
You can access the elements of an array using indexes:
cout << myArray[0]; // Outputs 10
Object-Oriented Programming (OOP) in C++
C++ supports object-oriented programming, including classes and objects, which makes it an excellent language for game development. Here’s an example:
class Car { public: string brand; string model; int year; }; int main() { Car myObj; myObj.brand = "Toyota"; myObj.model = "Corolla"; myObj.year = 2020; cout << myObj.brand; return 0; }
In the code snippet above, we defined a “Car” class with three properties and created a “myObj” object of the “Car” class. We accessed the properties using the dot ‘.’ operator.
Continued practice and learning will make C++ an excellent asset for your programming journey. Happy coding!
Pointers in C++
A pointer in C++ is a type of variable that stores the memory address of another variable. Pointers are often used for efficiency in certain programming routines, particularly those involving arrays and data structures.
int var = 5; int *p; p = &var; cout << var << "\n"; // Output: 5 cout << p << "\n"; // Output: address of 'var' cout << *p << "\n"; // Output: 5
The `*` symbol is used to declare a pointer and the `&` symbol is used to denote an address in memory. In this example, `p` is a pointer to an integer and it is assigned the address of variable `var`. The line `*p` then retrieves the value stored at the address `p`, which is `5`.
C++ Strings
Strings are used for storing text. C++ has a special `string` class that makes working with strings more intuitive.
string myName = "John Doe"; cout << "Hello, " << myName << endl;
String concatenation is done with the `+` operator:
string firstName = "John"; string lastName = "Doe"; string fullName = firstName + " " + lastName; cout << fullName;
Exception Handling in C++
Handling exceptions in C++ is done using three keywords: try, catch, and throw.
try { int age = 15; if (age > 18) { cout << "Access granted - you are old enough."; } else { throw (age); } } catch (int myNum) { cout << "Access denied - You must be at least 18 years old.\n"; cout << "Age is: " << myNum; }
In this example, if the age is lower than 18, an exception is thrown (the code inside the `throw` keyword). The exception is caught with a `catch` block.
File Handling in C++
C++ offers great flexibility when it comes to handling files. Here’s a way to write to a file:
#include using namespace std; int main() { ofstream myfile; myfile.open ("sample.txt"); myfile << "Writing this to a file.\n"; myfile.close(); return 0; }
This code snippet opens the file sample.txt (you may need to change the path depending on where you want to create this file), writes the text into the file, and then closes the file. File handling is crucial in saving data, reading data, and organizing data.
These wide range of features make C++ a versatile language and an excellent option for various coding tasks, particularly game development. Continued practice and engagement with each of these elements will lead to a mastery of this powerful language.
Where to Go Next?
The exciting journey of learning C++ is just beginning. As your aptitude for the language develops, continue to experiment, write code, refactor, and write again. Delve into the intricacies and capabilities of C++, looking to grow your understanding and ability to engage with complex problems.
At Zenva, we believe in the power of continued learning. We invite you to explore our comprehensive C++ Programming Academy, a graded learning hub that offers an in-depth study of C++ programming. From topics like programming flow to object-oriented programming, and even game mechanics, we provide a strategic stepping stone to sharpen your C++ skills. Also, take a moment to visit our broader collection of C++ Courses designed for a wider reach into C++ programming.
Zenva’s courses are hands-on, allowing you to integrate theory with practice as you work on actual projects. This prepares you for real-world coding challenges and helps you build a valuable portfolio. Remember, your learning journey with C++ is a marathon, not a sprint. Keep learning, keep coding and soon, you’ll be amazed at how far you’ve come.
Embrace the journey ahead. The joy of coding lies in constant learning and improvement. Happy programming!
Conclusion
In conclusion, there’s no denying the immense potential and wide-ranging application of C++. As you navigate the path to becoming a skilled C++ programmer, remember that the route may be challenging, but the reward is greatly fulfilling. With consistent practice and continual learning, you will soon be capable of building intricate programs, efficient algorithms, and even exciting game mechanics.
We, at Zenva, are committed to being your guiding light on this journey. Our C++ Programming Academy and other varied C++ Courses are designed to provide interactive, high-quality education that is both comprehensive and practical. Take the leap, dive deep into the world of C++, and embrace the coding adventure that awaits!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
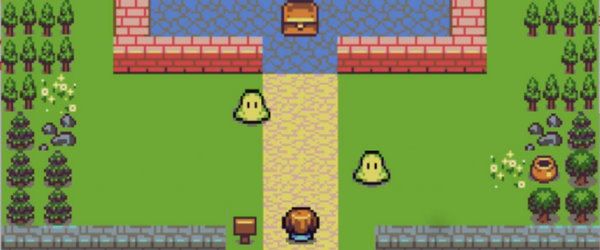
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.