Welcome to this comprehensive tutorial on mastering C++ practice! This guide will take a tour across various aspects of C++, a powerful and versatile programming language that holds a significant place in the world of coding. Whether you’re an absolute beginner or an experienced developer looking to refresh your skills, this tutorial offers engaging, valuable, and useful content tailored for you.
Table of contents
What is C++?
C++ is a statically typed, compiled, general-purpose, case-sensitive, free-form programming language that supports procedural, object-oriented, and generic programming. Intricately designed, it provides programmers a high level of control over system resources and memory.
Why learn C++?
The reach and utility of C++ are vast and multifaceted. Here’s why you should consider mastering it:
- Performance: C++ allows tight control over hardware resources which can optimize the performance of your code.
- Game Development: C++ is widely used in Game Development due to its high-speed execution and ability to extend and manipulate existing system components.
- Widely used in industry: C++ is used in a variety of applications from creating GUI applications to 3D graphics for games to real-time mathematical simulations.
Excited to dive in? Let’s get started with some exciting hands-on practice examples!
Basic C++ Syntax
Let’s start by understanding the basic syntax and structure of a C++ program:
#include int main() { std::cout << "Hello, Zenva!"; return 0; }
In this code snippet, we have included the iostream
library for Input/Output operations. The C++ journey begins with the main()
function. The cout
statement is used to print “Hello, Zenva!” on the screen.
Data Types and Variables
C++ has several different data types. Below we’ll declare variables with different data types and display their values:
#include int main() { int num = 10; double decimal = 3.14; char character = 'Z'; bool check = true; std::cout << "Integer: " << num << "\n"; std::cout << "Double: " << decimal << "\n"; std::cout << "Character: " << character << "\n"; std::cout << "Boolean: " << check << "\n"; return 0; }
In the above code, four different variable types: int
, double
, char
, and bool
have been declared and initialized. Then their values are displayed using the cout
statement.
Conditional Statements and Loops
Conditional statements like if, else paired with loops like while, for are powerful tools to make your program more intelligent. Let’s have a look at an example:
#include int main() { int number = 5; if (number > 0) { std::cout << "Positive number.\n"; } else { std::cout << "Non-positive number.\n"; } for (int i = 0; i < number; i++) { std::cout << i << "\n"; } return 0; }
In the above code, we check if a number is positive or not using the if...else
conditional statement. Then, we print all integers from 0 to 4 using the for
loop.
Functions
In C++, you can also write your own functions to perform certain actions. Observe this simple example:
#include void greet() { std::cout << "Welcome to Zenva!\n"; } int main() { greet(); return 0; }
Here, we have defined a function named greet
that prints a welcome message on the screen. This function is called within the main
function.
Arrays
Arrays are used to store multiple values of the same type under a single variable. Here’s how to declare, initialize and access an array:
#include int main() { int numbers[5] = {1, 2, 3, 4, 5}; for (int i = 0; i < 5; i++) { std::cout << numbers[i] << "\n"; } return 0; }
In this example, we declare an integer array named numbers
, then we access and print its elements one by one using a for
loop.
Pointers
Pointers in C++ are special variables used to store addresses of other variables. Here’s a simple usage of pointers:
#include int main() { int var = 20; int *pointerVar; pointerVar = &var; std::cout << "Value of var: " << var << "\n"; std::cout << "Address stored in pointerVar: " << pointerVar << "\n"; std::cout << "Value of *pointerVar: " << *pointerVar << "\n"; return 0; }
In this code snippet, we declare a pointer *pointerVar
and an integer var
. The memory address of var
is assigned to the pointer variable pointerVar
, as indicated by the ‘&’ operator.
Objects and Classes
C++ is an Object-Oriented Programming (OOP) language, which means it utilizes objects and classes to execute the code. Let’s create a simple class and an object of that class:
#include class Student { public: std::string name; void greet() { std::cout << "Hello, " << name << "\n"; } }; int main() { Student student1; student1.name = "Zenva"; student1.greet(); return 0; }
In the above code, we define a class `Student` with the data member ‘name’ and a member function `greet()`, which displays a greeting message. Then, we create an object `student1` of the `Student` class and use it to access the class members.
Error Handling
Error handling is a crucial part of writing robust, fail-proof code. C++ does this with exceptions. Here’s how:
#include double division(int a, int b) { if (b == 0) { throw "Division by zero condition!"; } return (a/b); } int main () { int x = 50; int y = 0; try { double z = division(x, y); std::cout << z << "\n"; } catch (const char* msg) { std::cerr << msg << "\n"; } return 0; }
In the above code, we define a function called `division` that throws an error message if there is an attempt to divide by zero. This exception is caught and handled in the `main` function within the `try…catch` block.
These examples should give a taste of the broad range of capabilities C++ offers. At Zenva, we strive to provide resources that help you not just to learn but to master the intricacies of languages like C++.
Strings
Strings are a sequence of characters. C++ has library functions to manipulate strings. Here’s how you can define and manipulate strings:
#include #include int main () { std::string str1 = "Hello"; std::string str2 = "Zenva"; std::string str3; str3 = str1 + " " + str2; std::cout << str3 << "\n"; return 0; }
In the above code, we create two strings str1
and str2
and then concatenate them using the ‘+’ operator.
File I/O
C++ provides methods to handle Input/Output (I/O) operations through the fstream
library for file manipulation. Here’s a basic code snippet showing file writing operation:
#include #include int main () { std::ofstream outfile("test.txt"); outfile << "Zenva the leading online academy\n"; outfile.close(); return 0; }
This code creates a file named ‘test.txt’ and writes a string into it using the ofstream
object.
Templates
Templates in C++ allow functions and classes to operate with generic types. This allows for more flexible and reusable code:
#include template T maximum(T num1, T num2) { return (num1 > num2) ? num1 : num2; } int main() { int int1 = 5, int2 = 10; std::cout << "Maximum Integer: " << maximum(int1, int2) << "\n"; double float1 = 3.5, float2 = 7.2; std::cout << "Maximum Float: " << maximum(float1, float2) << "\n"; return 0; }
This code illustrates a template function maximum
which returns the maximum of two input values of any data type.
STL – Standard Template Library
The Standard Template Library (STL) is a powerful, must-learn feature of C++, which has ready-to-use templates for various data structures, algorithms, and iterators. Here’s a basic usage of STL vector:
#include #include int main() { std::vector v; for (int i = 1; i <= 5; i++) { v.push_back(i); } std::cout << "Size : " << v.size(); std::cout << "\nElements : "; for (int i=0; i<v.size(); i++) { std::cout << v[i] << " "; } return 0; }
This code demonstrates the usage of an STL vector. Vectors are similar to dynamic arrays with the ability to resize itself automatically when an element is inserted or deleted.
A solid understanding of these topics should equip you with a strong foundation in C++, and be a springboard into more advanced topics and applications. We at Zenva are excited to guide you through your learning journey as you delve deeper into this versatile and widely used programming language.
We hope you found this tutorial engaging and insightful, and you’re now more prepared to dive further into the wonderful world of C++. The journey doesn’t stop here. To keep learning and advancing your C++ skills, we encourage you to check out our C++ Programming Academy. This comprehensive course has been tailor-made, focusing on building simple games like text-based RPGs, clicker games and roguelike dungeon crawlers, sure to take your programming journey to the next level.
Moreover, we offer an expansive collection of C++ Courses to further complement your learning. Our project-based courses are flexible and could be accessed 24/7, allowing learners to progress at their own pace. More than just learning to code, the courses at Zenva empower you to create and innovate with confidence and proficiency.
C++ is a powerful language with a high demand in the industry, from game development to AI. The road to mastering C++ may seem intimidating at first, but with Zenva, you have a personal tool-kit and ally in your journey. From beginner to professional, we’re behind you every step of the way. So keep learning, keep creating, and let’s code the future together!
Conclusion
Mastering C++ opens up an assortment of opportunities for everyone from hobbyist programmers to career developers. It’s a pathway to creating games, developing competitive algorithms, and even launching yourself into the evolving landscape of artificial intelligence.
Remember, coding is not just about learning, it is also about creating, innovating, and applying these skills to solve real-world problems. Don’t just stop here, explore more with our comprehensive C++ Programming Academy and turn your code into your canvas. Happy coding!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
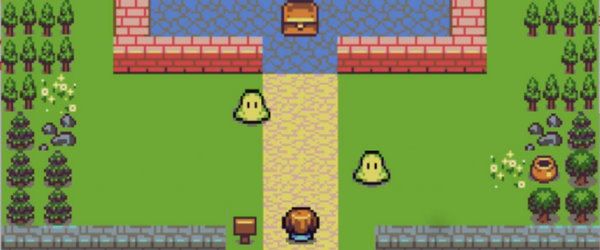
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.