Table of contents
C++: A Beginner’s Guide
Delving into coding can seem like a daunting task for beginners, but worry not! We’re here to make that journey not only smoother but more fun and engaging. Our special focus today is on C++, a universally popular language that opens the door to exciting areas like game development and high-performance computing.
What is C++?
C++ is an intermediate-level language that offers a blend of high-level and low-level programming features. Created as an extension to the C programming language, C++ brings more flexibility and efficiency into coding with its features like classes and objects, encapsulation, inheritance, and polymorphism.
Why learn C++?
But why should you, as a beginner, resolve to learn C++? Here are some key points that make C++ a valuable asset in your coding journey:
- Wide Application: C++ is used in diverse areas including game development, operating systems, and even in medical and engineering applications.
- Performance: C++ programs are known for their efficient execution and better control over system resources.
- Base for other languages: C++ forms the base for understanding more complex programming languages, thus making your learning curve smoother.
Setting up your C++ Environment
Before you dip your toes into coding, you need to set up your C++ environment. Here, we’ll use an online compiler for simplicity, but you can use any C++ compiler like GCC, Clang, or Visual Studio on your system.
// Your first C++ Program #include<iostream> int main() { std::cout << "Hello, World!"; return 0; }
In the above program, ‘#include<iostream>’ is a library inclusion statement. ‘int main()’ is the main function where our program starts. ‘std::cout’ is used to output the line ‘Hello, World!’.
Data Types and Variables
C++ supports several different data types. These are fundamental to how you’ll manipulate your data within C++. Take a look at the following example:
// Your C++ Program #include<iostream> int main() { int myNum = 5; // Integer (whole number) float myFloatNum = 5.99; // Floating point number double myDoubleNum = 9.98; // Floating point number char myLetter = 'D'; // Character bool myBoolean = true; // Boolean std::string myText = "Hello"; // String return 0; }
This code shows the usage of different data types like integer, float, double, character, boolean, and string, and how to declare variables of these types.
Operators in C++
C++ uses a set of operators allowing you to perform operations between variables. Arithmetic operators, for example, resemble those you’d use in basic math.
// Your C++ Program #include<iostream> int main() { int x = 10; int y = 20; int z = x + y; // addition int k = x * y; // multiplication std::cout << z << std::endl; std::cout << k << std::endl; return 0; }
The output of the above program will be 30 and 200, respectively. It showcases the usage of addition and multiplication operators on integer variables.
Control Flows
C++ includes control structures that allow for more complicated execution paths. For example, ‘if’, ‘else if’ and ‘else’ statements in C++ allow your program to selectively execute certain blocks of code.
// Your C++ Program #include<iostream> int main() { int x = 20; int y = 20; if (x > y) { std::cout << "x is greater than y" << std::endl; } else if (x < y) { std::cout << "x is less than y" << std::endl; } else { std::cout << "x and y are equal" << std::endl; } return 0; }
In the above program, the output will be ‘x and y are equal’. Thus, it demonstrates the basic if, else if and else Conditional Statements in C++.
Loops in C++
Loops are powerful tools in the programming world that enable repetitive execution of a block of code.
There are three types of loops in C++: ‘for’, ‘while’, and ‘do-while.
Let’s take a look at examples of each loop structure:
// 'for' loop in C++ #include<iostream> int main() { for(int i = 1; i <= 5; i++) { std::cout << "Number: " << i << std::endl; } return 0; }
In the above ‘for’ loop, the variable ‘i’ is initialized to 1. The loop will continue executing as long as ‘i’ is less than or equal to 5. With each iteration, ‘i’ is increased by 1.
// 'while' loop in C++ #include<iostream> int main() { int i = 1; while(i <= 5) { std::cout << "Number: " << i << std::endl; i++; } return 0; }
In the ‘while’ loop, the condition is checked before the loop is executed. If the condition is true, then the loop will continue.
// 'do-while' loop in C++ #include<iostream> int main() { int i = 1; do { std::cout << "Number: " << i << std::endl; i++; } while(i <= 5); return 0; }
The ‘do-while’ loop checks the condition at the end of the loop. This ensures that the loop block is executed at least once.
Functions in C++
Functions in C++ support the concepts of reusability and modularity. A function can be defined as a set of code meant to perform a specific task.
// Function in C++ #include<iostream> void sayHello() { std::cout << "Hello, World!" << std::endl; } int main() { sayHello(); return 0; }
A function is declared and defined before the main method in the above example. We invoke this function in the main method.
Classes in C++
In object-oriented C++, classes act as blueprints for creating objects. Classes can contain functions and variables, which represent the states and behaviors of objects.
// Class in C++ #include<iostream> class MyClass { public: int myNum; std::string myString; }; int main() { MyClass myObj; myObj.myNum = 15; myObj.myString = "Hello"; std::cout << myObj.myNum << std::endl; std::cout << myObj.myString << std::endl; return 0; }
In the above example, a class ‘MyClass’ is declared with public variables ‘myNum’ and ‘myString’. The values for these attributes are set through an object ‘myObj’ in the ‘main()’ function.
By learning these foundational components of C++, you’re taking your first steps into the vast world of programming! It might seem like a big task to learn a programming language like C++, but with consistent practice and a hands-on approach, you’ll find yourself growing as a coder. Keep on learning and keep on coding!
Arrays and Strings in C++
Arrays and strings are essential structures in C++ programming. An array is a collection of similar data types stored in contiguous memory locations. A string, on the other hand, is an array of characters.
Let’s take a look at an array example in C++:
// Array in C++ #include<iostream> int main() { int myArray[3] = {10, 20, 30}; // An array of integers for(int i=0; i<3; i++) { std::cout << myArray[i] << std::endl; } return 0; }
The above example illustrates an array with three elements, which are printed onto the console using a ‘for’ loop.
Strings in C++ are used to store sequences of characters in an array format:
// String in C++ #include<iostream> #include<string> int main() { std::string myString = "Hello, World!"; std::cout << myString << std::endl; return 0; }
In the above program, the ‘string’ data type is used to declare a string variable, ‘myString’, and the value is then printed to the console.
Pointers in C++
Pointers in C++ are a powerful but tricky feature. A pointer is a variable whose value is the address of another variable in the memory. Let’s see how we can declare and use pointers in C++:
// Pointer in C++ #include<iostream> int main() { int x = 10; int* p = &x; std::cout << "The value stored in 'x' is: " << x << std::endl; std::cout << "The address of 'x' is: " << p << std::endl; std::cout << "The value pointed by 'p' is: " << *p << std::endl; return 0; }
In the above example, ‘p’ is a pointer to ‘x’. The ‘p’ pointer stores the memory address of ‘x’, and the * operator is used to get the value stored at that address.
STL in C++
The C++ Standard Library, also known as the STL, offers a set of ready-to-use templates to make programming more intuitive and efficient.
Let’s look at a basic STL example using vectors, which are dynamic arrays:
// Vector in C++ #include<iostream> #include<vector> int main() { std::vector<int> myVector = {1, 2, 3, 4, 5}; for(int i = 0; i < myVector.size(); i++) { std::cout << myVector[i] << std::endl; } return 0; }
In the above program, we’ve declared a vector of integers and printed it using a ‘for’ loop.
Another useful STL tool is the ‘map’ container, which stores elements in a key-value pairing:
// Map in C++ #include<iostream> #include<map> int main() { std::map<std::string, int> myMap; myMap["Alice"] = 28; myMap["Bob"] = 32; std::cout << "Alice: " << myMap["Alice"] << std::endl; std::cout << "Bob: " << myMap["Bob"] << std::endl; return 0; }
In this example, the ‘map’ ‘myMap’ stores the relationship between names (key) and ages (value), which we subsequently print onto the console.
C++ is a vast language and this tutorial only scratches the surface. By learning and continuously practising the basics and trying out new things as you learn, you can become proficient in C++. Remember, practice is the key – so happy coding!
Where to Go Next: Continuing Your C++ Journey
The journey of learning C++ doesn’t end here. The key to mastering any skill is continuous learning and practice. The power of C++ can be harnessed in various domains – from operating systems to video games. Dive deeper, grasp the intricacies, and challenge yourself to solve complex problems with C++.
We recommend checking out our C++ Programming Academy. Our academy caters to all levels of learners, from novice to professionals. The courses focus on developing simple games, honing your logical thinking, and strengthening your grasp of programming concepts. They come with the flexibility of learning at your pace, coupled with hands-on, project-based courses.
To get an overview of our wide variety of C++ courses, you can also take a look at our C++ Course catalog. Remember, the world of coding unfolds with each line of code you write. So, continue writing, debugging, and exploring! Happy coding!
Conclusion
Embarking on your coding journey with C++ is an exciting step towards unlocking new possibilities in the world of programming. Its robustness and versatility make it a solid and practical choice for a wide range of applications – from game development to system software and everything in between.
At Zenva, we are ready to equip you with the knowledge you’ll need to conquer your coding aspirations with C++. Whether you’re a beginner just stepping into this dynamic field or an experienced programmer desiring to enhance your C++ skills, we’re here to guide you each step of the way. Happy coding!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
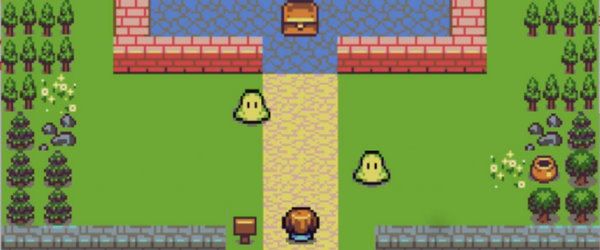
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.