Welcome to this fun and engaging GDScript tutorial where we take a deep dive into the fascinating world of vectors, specifically, the vector2. Possibly you’ve heard of vectors in other programming languages, or maybe this is your first introduction to these magical tools. Either way, by the end of this tutorial, you’ll not only understand why vectors are essential elements of game creation, but also, you’ll be able to apply these principles on your own.
Vector2 in GDScript, an essential built-in type in the Godot game engine, allows for a versatile approach to manipulating 2D space. Whether you’re designing a game with intricate physics, crafting dynamic player movements, or even implementing a UI system, Vector2 is your swiss knife.
Table of contents
What is Vector2?
A Vector2 in GDScript is a two-component representation of a physical quantity that has both magnitude (size) and direction. It is most commonly used to represent 2D positions and movements within the Godot engine. A Vector2 consists of two values usually denoted as X and Y.
Why Should I Learn About Vector2 in GDScript?
Game creation in Godot without understanding and utilizing Vector2 is like trying to design a chessboard without knowing the movements of the pieces. The Vector2 component is central to Godot 2D game development, offering a depth of strategies to implement precise movements, control object interactions, enable pathfinding, and beyond.
Moreover, understanding vectors in GDScript can open doors for not only 2D, but also 3D game development as you progress in your learning journey. Having clarity about this foundational concept can improve your game design skills, enhance your problem-solving ability, and most importantly, bring your game ideas to life.
How to use Vector2 in GDScript
Enough with the talking, let’s dive right in. Our journey will begin with creating and assigning values to a Vector2.
var vector = Vector2(2, 3)
Above, we define a Vector2 with the values 2 (X component) and 3 (Y component). These values represent the magnitude in the X and Y directions, respectively.
Once a vector is defined, we can manipulate the individual components. Let’s change the X component of our vector.
vector.x = 5
The above line changes the X component of our ‘vector’ to 5.
What about accessing the values in a Vector2? Simple, just use ‘.x’ or ‘.y’ with your vector variable.
print(vector.x) print(vector.y)
Vector2 Operations
Vector2 supports a suite of operations, such as addition, subtraction, multiplication and more, which can enable unique expressions of motion and distance in your game world. Let’s look into some of them.
1) The simplest operation is Vector Addition.
var vector1 = Vector2(2, 3) var vector2 = Vector2(3, 4) var result = vector1 + vector2
The ‘result’ will be a new Vector2 representing the sum of ‘vector1’ and ‘vector2’, that is (5, 7).
2) Vector Subtraction also follows the similar pattern.
result = vector1 - vector2
The ‘result’ now contains a new Vector2 representing the difference between ‘vector1’ and ‘vector2’, which is (-1, -1).
3) Scaling a vector can be useful for when you want to change the length (magnitude) of the vector without altering its direction. You can accomplish this with a multiplication operation.
result = vector1 * 2
This code will multiply every component of ‘vector1’ by 2, effectively scaling its original size.
More Vector2 Operations
Understanding operations with vectors doesn’t stop there, there are a plethora of other exciting and meaningful operations that you can perform.
4) Division can be applied similarly to multiplication, but instead, it reduces the magnitude of the vector.
result = vector1 / 2
This will divide every component of ‘vector1’ by 2, in effect, reducing its magnitude by half.
5) What about comparing vectors for equality? GDScript has you covered.
print(vector1 == vector2)
This code will return ‘true’ if ‘vector1’ and ‘vector2’ have the same x and y values, and ‘false’ otherwise.
6) Godot also provides us with the dot product operation, which is a crucial tool for determining the angle between two vectors.
var dotProduct = vector1.dot(vector2)
The ‘dotProduct’ here stores the result of the dot product operation between the vectors ‘vector1’ and ‘vector2’.
7) We can calculate the length of our vector using the ‘length()’ function.
print(vector1.length())
This will print out the magnitude or length of the ‘vector1’.
8) Not only can we calculate the length of a vector, but we can also normalize it. Which means setting the length/magnitude of a Vector2 to 1 while keeping its direction intact. This is beneficial while dealing with unit vectors.
vector1 = vector1.normalized()
The ‘vector1’ now holds a normalized version of itself, with a magnitude of 1.
Remember, GDScript provides even more vector functions than we’ve covered here. The goal of this tutorial is to cover the basics and encourage further exploration and learning. You’ll find that understanding and making good use of vectors in GDScript is an incredible asset you’ll want to have under your belt while working with Godot.
As the leading online academy for coding, game creation, and more, we at Zenva are dedicated to delivering high-quality and engaging content to our learners. We hope you enjoyed this Vector2 tutorial and look forward to assisting you in future lessons!
Vector2 in Action: Practical Examples
Now that we’ve gone through the basics, let’s take a look at how to use Vector2 for a more practical purpose: movement and collision detection in a game setting.
Please pay attention to how Vector2 can assist in the simulation of real-world physics and the path-finding algorithm, a common navigation method used in games.
Moving an Object
Want to move an object in your 2D game? Vector2 is the key! Let’s say, for instance, we want to move a spaceship in an upward direction.
var speed = 200 var direction = Vector2(0, -1) var velocity = direction * speed
Here, we have created a ‘direction’ vector pointing up (the negative Y-direction in 2D space) and scaled it by a ‘speed’. The scaled result is stored in the ‘velocity’ variable, applied in the game logic for the spaceship.
Calculating Distance
Vector2 can also assist us in calculating the distance between two points, which can be useful for collision detection or triggering events when an object is within a certain range.
var pointA = Vector2(2, 3) var pointB = Vector2(5, 7) var distance = pointA.distance_to(pointB)
Now the ‘distance’ variable contains the distance from pointA to pointB.
Rotating a Vector
On occasions, you may need to rotate the direction of a vector in your game. Here’s how to do that.
var direction = Vector2(0, -1) direction = direction.rotated(PI/2)
Above, the direction vector is rotated 90 degrees (PI/2 radians) to the right.
Pathfinding with Vector2
Vector2 can also come in handy when dealing with AI pathfinding algorithms. If you want to have an enemy character move towards a player character, you can do it as follows.
var enemy_position = Vector2(2, 3) var player_position = Vector2(5, 7) var direction_to_player = (player_position - enemy_position).normalized()
Here we calculate the difference between the player’s position and the enemy’s position, normalize it, and obtain a vector point from the enemy position to the player position.
So there you have it! This tutorial has provided you some insights into how powerful and practical Vector2 is in GDScript. It’s undeniable that mastering Vector2 is essential to game creation with Godot. We wish to see you apply these Vector2 operations in your own Godot projects and build exciting, innovative games. Happy coding!
Where to Go Next?
So, you’ve dipped your toes into the vast universe of Vector2 and GDScript. Now you’re probably wondering, how to keep learning and expanding your game development skills. Look no further! Discover our Godot Game Development Mini-Degree, an extensive program that covers a range of topics in game development like 2D and 3D assets, combat mechanics, gameplay control flow, UI systems, and more.
Whether you’re a coding novice seeking a career in game development or an experienced dev wanting to master the Godot engine, this mini-degree caters to all. Our flexible learning model allows for live coding lessons, interactive quizzes, alongside development in actual Godot projects – a truly immersive learning experience matched with real-world applications!
And the adventure doesn’t stop there! At Zenva, we strive to provide a rich learning environment for coding enthusiasts. Check out our broader collection of Godot Courses for even more insights and knowledge. Remember, the realm of coding and game development offers endless opportunities. Go forth and conquer them, one Vector2 at a time!
Conclusion
Embracing the world of vectors in GDScript, particularly with Vector2, is a significant stride for anyone aspiring to excel in game creation with Godot. Not only does understanding Vector2 empower you to construct dynamic and engaging game environments, but it also paves the way towards an exciting journey of endless potentialities in this realm of 2D and 3D game development.
Have you been intrigued by the prospects of harnessing the power of Vector2 for your game creation venture? Why not take the next big step in your learning journey? Join us at Zenva’s Godot Game Development Mini-Degree where we transform coding enthusiasts into industry-ready developers. Venture into the vast universe of coding, game design, and more with us today!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
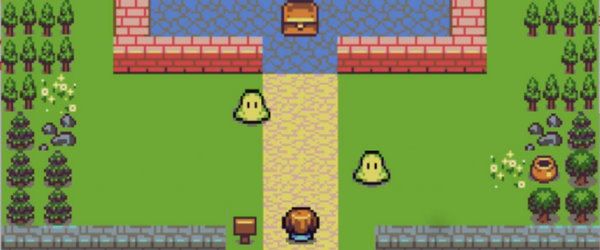
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.