With all the exciting opportunities in game development, learning the essential programming skills is paramount. One of the most useful features in GDScript, Godot’s built-in scripting language, is formatting strings. You can create more dynamic and interactive scenarios, making the gaming experience more immersive for players with this feature. Let’s dive deeper and explore the string formatting system in GDScript.
Table of contents
What is GDScript String Format?
String format in GDScript is essentially a tool that enables customization of string values, or text, in code. It harnesses powerful inbuilt functions which allow us to format strings in various ways. This could involve embedding variables or changing character cases, enabling us to enrich the functionality and versatility of our code.
What is it for?
String formatting provides versatility in game development. It becomes useful in situations where we need to display changing values in the game’s user interface. Whether it’s showcasing a player’s score, updating inventory items, or generating dynamic dialogue, string formatting is an essential tool. With string formatting, we can update the text displayed based on the game state.
Why should I learn it?
With GDScript’s string formatting capabilities, you’ll be developing more interactive games which sell and engage players. Beyond Godot and game development, knowing how to properly format strings is an important skill for any programmer or developer. It’s a universal concept you’ll come across in almost any programming language.
Basics of GDScript String Formatting
Let’s begin by understanding how the format() function works. It allows us to insert variables into a string dynamically, using a special notation known as placeholders, denoted by “{}”.
var my_name = "Alex" print("Hello, {}".format(my_name)) # prints "Hello, Alex"
Here, ‘my_name’ is the variable and ‘{}’ is the placeholder. When the format function is called, it replaces the ‘{}’ with the value of the variable we supply.
It is possible to use multiple placeholders in a string. You can also reference the variable for a placeholder by its positional index, making the string more readable. Let’s see a practical example:
var my_name = "Alex" var my_age = 30 print("{0} is {1} years old.".format(my_name, my_age)) # prints "Alex is 30 years old."
Manipulating Strings with GDScript
GDScript offers functions to manipulate strings directly. For instance, you may want to capitalize the first letter of a string, or perhaps convert all letters to uppercase. Here’s how you can do it:
var my_string = "hello world" print(my_string.capitalize()) # prints "Hello world" print(my_string.to_upper()) # prints "HELLO WORLD"
Let’s look at some more methods for string manipulation. Below, we’ll convert our string to lowercase and remove any leading or trailing whitespace:
var my_string = " HELLO WORLD " print(my_string.to_lower()) # prints "hello world " print(my_string.strip_edges()) # prints "HELLO WORLD"
These examples should provide a solid foundation for GDScript string formatting and manipulation. Remember, mastering these basic principles will greatly enhance your game development capability and code readability.
Advanced GDScript String Formatting
GDScript also supports more complex string formatting (such as adjusting the field size for variables or controlling decimal places), which can be useful if you need more control over your output format. We achieve this by adding modifiers within the placeholder.
Let’s say you want to display a score where the field size for the score is always five digits. You can achieve this by adding a modifier inside the placeholder like so:
var score = 123 print("Your score: {:05d}".format(score)) # prints "Your score: 00123"
Here, ’05d’ specifies a field width of five digits, and ‘0’ is the fill character.
Formatting Floating Numbers
Similarly, when dealing with floating numbers (numbers with decimal points), you can control the number of decimal places. Here’s how:
var pi_value = 3.14159 print("Value of Pi to 2 decimal places: {:.2f}".format(pi_value)) # prints "Value of Pi to 2 decimal places: 3.14"
With the ‘f’ specifier and argument before it ‘.2’, we indicate that the variable is a floating-point number with two digits after the decimal point.
Aligning Strings
String formatting in GDScript also allows us to align text as per our needs. Depending on your game’s UI design, you may want to right-align, left-align, or center text.
var my_string = "Hello" print("{:*>10}".format(my_string)) # prints "*****Hello" (right align) print("{:*<10}".format(my_string)) # prints "Hello*****" (left align) print("{:*^10}".format(my_string)) # prints "**Hello***" (center align)
‘*’ is the fill character, and ’10’ is the field width. Note the use of ”, and ‘^’ to signify left align, right align, and center align, respectively. Using these formatting principles can make your game display more visually organized, improving the player’s experience.
Conclusion
String formatting is a powerful tool in GDScript and an essential skill in game development. These techniques will serve you well as you proceed with your game creation journey. Always remember to practice and apply these principles to create engaging, dynamic gameplay experiences. Happy coding!
Enhanced Formatting with Named Placeholders
In GDScript, you can also use named placeholders for enhanced readability. This technique becomes useful when dealing with multiple variables, making your code easier to read and manage.
var player = "Alex" var coins = 150 print("Player {name} has collected {collected} coins".format(name=player, collected=coins)) # prints "Player Alex has collected 150 coins"
Here, ‘name’ and ‘collected’ are named placeholders, which make our string formatting more concise and expressive.
Escaping Placeholders
In some situations, you might need to include a literal brace character, ‘{‘ or ‘}’, in a string. To ensure GDScript doesn’t treat it as a placeholder, you ‘escape’ it by doubling it. Let’s see this in practice:
var product = "Game" print("You can get a 50% discount on any {item} on Black Friday!!".format(item=product)) # prints "You can get a 50% discount on any Game on Black Friday!!"
But what if we want the output as “You can get a 50% discount on any {Game} on Black Friday!!”? To escape the braces, we just double them:
var product = "Game" print("You can get a 50% discount on any {{}} on Black Friday!!".format(product)) # prints "You can get a 50% discount on any {Game} on Black Friday!!"
Manipulating String Characters
GDScript has several helpful functions to analyze and manipulate the characters in a string. Here are a few to explore:
To count the number of occurrences of a specific character or substring:
var my_string = "Hello, World!" print(my_string.count("l")) # prints "3", as "l" occurs three times.
And to find the first occurrence of a specific character or substring:
var my_string = "Hello, World!" print(my_string.find("o")) # prints "4", as "o" first appears at index 4.
Remember, string indices start from 0. Thus, the first character of the string is at index 0, the second character at index 1, and so on.
These string manipulation capabilities of GDScript can greatly enhance the interactivity and dynamism of your in-game dialogues and text displays.
Nothing beats learning more than getting your hands dirty with code. We encourage you to fiddle around with what you’ve learned here and explore new boundaries. Stay tuned for more thrilling tutorials from us at Zenva. Happy coding!
Where to Go Next?
Armed with your newfound knowledge of GDScript string formatting and manipulation, your journey into the exciting world of game development has only just begun. To keep this momentum going, check out our comprehensive Godot Game Development Mini-Degree. This mini-degree covers an array of topics, including rendering graphics, mastering GDScript, gameplay control flow, combat systems, item collection, UI systems, and much more.
No matter your level of experience, there is something to gain from this series of accessible and engaging courses. You’ll have an opportunity to create games in various genres, like RPGs, RTS games, and survival games. This will expose you to broader aspects of game development, making you a more versatile and competent game developer.
In line with our commitment to providing a holistic learning experience, we at Zenva offer several more Godot-specific courses. From beginners to experienced developers, our courses are tailored to suit the needs of everyone. So go ahead and dive deeper into the world of game development with us!
Conclusion
Understanding how to efficiently manipulate strings can drastically improve the quality of your work as a game developer. This invaluable skill translates into creating more engaging, dynamic, and interactive gameplay experiences. As you continue on your journey to becoming a proficient game developer, these newfound skills will pave the way to creating some truly innovative and immersive games.
Here at Zenva, we invite you to continue building on what you’ve learned. Enroll in our Godot Game Development Mini-Degree and see where your creativity and our combined knowledge can lead. No matter where you are in your coding journey, there’s always more to learn, and we’re here to help you every step of the way.
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
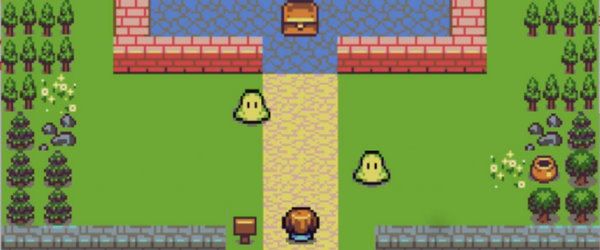
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.