Welcome to our interactive tutorial on Dev-C++! This fantastic integrated development environment, more commonly known as an IDE, is a brilliant tool for any fledgling coder embarking on their programming journey with C++. And guess what? Even if you’re a seasoned programmer looking to brush up old concepts or learn something new, you’re in the right place!
Table of contents
What is Dev-C++?
Dev-C++ is a full-featured IDE encompassing a wide array of functionalities that makes it a favorite amongst coders. Think of it as an all-in-one suite, where you can write, compile, execute, and debug your C++ code.
Why Dev-C++?
One may wonder, with a variety of other compilers available, why choose Dev-C++? A few reasons stand out:
- Friendly User Interface: Dev-C++ boasts of a very intuitive and clean GUI which makes it easier for beginners to navigate and write code.
- Free and Open Source: Being free and open source, you can inspect its source code, modify it or even distribute it.
- Portable: You can carry it in your USB drive and use it on any Windows PC you encounter.
- Feature-Rich: It opens doors to a whole world of programming with numerous libraries and templates that it supports. For example, graphics, networking, algorithms, etc.
Exciting, right? So, let’s dive straight into some hands-on coding!
Getting Started with Dev-C++
Starting with Dev-C++, the first step is indeed writing a simple “Hello, World!” program. This tradition is a neat way to wet your feet into the vast ocean of programming language. Let’s get coding!
#include int main() { std::cout<<"Hello, World!"; return 0; }
In this piece of code, we use the std::cout command to print “Hello, World!”. The angle brackets (<<) are streaming operators.
Working with Variables
In C++, we commonly use three basic variable types: int (for integer), char (for character), and float (for floating point numbers). Let’s look at an example:
#include int main() { int age = 21; char initial = 'A'; float pi = 3.14; std::cout<<"Age: "<<age<<"\nInitial: "<<initial<<"\nValue of Pi: "<<pi; return 0; }
Here, an integer variable ‘age’ is assigned the value 21, a character variable ‘initial’ is assigned ‘A’, and a float variable ‘pi’ is given the value of 3.14.
Conditionals in C++
Conditional statements are crucial control structures that allow our program to make decisions. The generic structure of an if-else statement is as follows:
#include int main() { int age = 15; if (age>=18) { std::cout<<"You are eligible to vote!"; } else { std::cout<<"You are not eligible to vote."; } return 0; }
This piece of code checks for the eligibility to vote based on the age. If the age is >=18 years, it prints “You are eligible to vote!”, else it prints “You are not eligible to vote.”
Loops in C++
Loops are fundamental control structures that keep executing a block of code until a certain condition is met. The basic for loop is demonstrated below:
#include int main() { for(int i = 1; i <= 10; i++) { std::cout<<i<<"\n"; } return 0; }
In this piece of code, a for loop is used to print numbers from 1 to 10. The loop continues until the condition i <= 10 is met.
Arrays in C++
Arrays are commonly used data structures that can store a fixed-size list of items of the same type. Let’s take an example:
#include int main() { int numbers[5] = {1, 2, 3, 4, 5}; for(int i = 0; i < 5; i++) { std::cout<<numbers[i]<<"\n"; } return 0; }
In this code snippet, an integer array called ‘numbers’ stores the first 5 natural numbers. A for loop then iterates over these numbers to print them.
Functions in C++
Functions are reusable pieces of programs that perform a specific task. Functions prevent us from rewriting the same code again and again.
#include void greet() { std::cout<<"Hello, welcome to Zenva Academy!"; } int main() { greet(); return 0; }
In this code snippet, a function named ‘greet’ is defined which simply prints a welcome message to the user. This function is then called inside the ‘main’ function.
In C++, we can also use functions with parameters and return types. Let’s take an example:
#include int add(int a, int b) { return a + b; } int main() { int sum = add(5, 10); std::cout<<"The sum is "<<sum; return 0; }
In this piece of code, we’ve defined a function ‘add’ that takes two integer parameters ‘a’ and ‘b’, and returns their sum. Within the ‘main’ function, we’ve called this ‘add’ function with arguments 5 and 10, and stored the result in ‘sum’.
User Inputs in C++
Reading user input is another important aspect of programming. In C++, this can be done using ‘std::cin’.
#include int main() { int age; std::cout<>age; std::cout<<"You are "<<age<<" years old."; return 0; }
In this example, ‘std::cin’ is used to read an integer input from the user that is then stored in the variable ‘age’. The age is later printed using ‘std::cout’.
We hope that this tutorial on Dev-C++ gave you an insightful look into the basics of C++ programming. Remember, practice is key to mastering any language, so do give these examples a try. Happy coding!
Classes and Objects in C++
In C++, we often talk about classes and objects. A class is a blueprint for creating objects, and an object is an instance of a class.
#include class Car { public: int speed; std::string color; void accelerate() { speed += 5; std::cout << "The car speeds up to "<< speed <<" mph.\n"; } }; int main() { Car ferrari; ferrari.speed = 10; ferrari.color = "red"; ferrari.accelerate(); std::cout << "The Ferrari is " << ferrari.color; return 0; }
In this code, a class named ‘Car’ with two attributes (speed and color) and a method ‘accelerate’ is created. Later, an object ‘ferrari’ of the class ‘Car’ is instantiated.
Controlling Access with Private and Public
In C++, we have access specifiers that define the scope of a member within a class. The most commonly used ones are ‘public’ and ‘private’.
#include class Box { private: int length; public: void setLength(int l) { if(l > 0) { length = l; } } int getLength() { return length; } }; int main() { Box box1; box1.setLength(5); std::cout<<"Length of box is "<<box1.getLength(); return 0; }
In this example, ‘length’ is a private member of the class ‘Box’. It’s manipulated using public member functions ‘setLength’ and ‘getLength’.
Handling Exceptions
C++ provides us with an exceptional handling framework to deal with runtime errors in a systematic manner. A simple try-catch block is illustrated below:
#include int main() { int num1, num2; std::cout<>num1>>num2; try { if(num2 == 0) { throw "Division by zero error"; } else { std::cout<<"The division is "<< num1/num2; } } catch(const char* exp) { std::cout<<exp; } return 0; }
This piece of code catches an exception thrown when a division by zero is attempted, providing a safer way to handle exceptions.
Working with Files
C++ provides support for handling files to let us write, read, and manipulate data from and to files.
#include #include int main() { std::ofstream outfile ("output.txt"); outfile << "This is a file handling example."; outfile.close(); std::string line; std::ifstream infile ("output.txt"); while (getline(infile, line)) { std::cout << line << '\n'; } infile.close(); return 0; }
This example first creates or opens a file named ‘output.txt’, writes a line into it, and then closes the file. After that, it opens the file again for reading and prints its content on the screen.
By running through all these examples, we can see how versatile and comprehensive C++ and the Dev-C++ environment can be. Whether you’re creating classes and objects or writing intricate loops, practice is critical. Keep those ideas flowing and the keys clicking!
Where to Go Next?
The journey of learning never truly ends – it only morphs into rediscoveries and further explorations. This is particularly true for a versatile language like C++. Luckily for you, we’re keen on helping you continue this journey in the best way possible.
We invite you to further your learning journey with our C++ Programming Academy. The courses offered in this academy cover a wide scope, from the basics of C++ to more advance topics, like object-oriented programming and game mechanics with SFML. You’ll have the chance to work on exciting projects and build a portfolio that will surely impress.
Additionally, for a more diverse range of options, you can explore our selection of C++ Courses. Remember, no matter what your current skill level, we’re here to help you grow and achieve your coding aspirations. Happy coding!
Conclusion
Our journey through the world of C++ programming with Dev-C++ has been truly memorable, with each step revealing new insights and secrets about coding. Now that you possess this arsenal of knowledge, we believe you have the power to create amazing projects and solutions, all while enhancing your coding skills.
Remember, every great programmer started with a single line of code, and we’re here to guide you through it all. Continue your thrilling coding journey with us at Zenva Academy’s C++ Programming Bundle. Adventure awaits, fellow coder!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
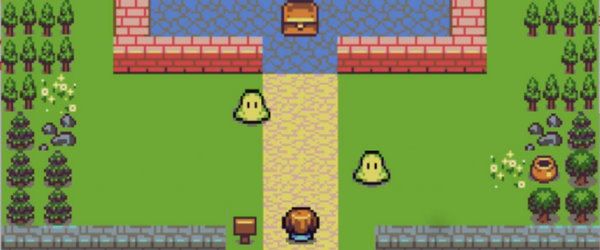
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.