Dive into the world of game development with GDScript and elevate your programming skills to another level. Welcome to our comprehensive guide to GDScript switch, a powerful tool underpinning game mechanics and interactivity in countless games.
Table of contents
What is GDScript Switch?
GDScript Switch is a control flow construct used to select one pathway of execution from multiple possibilities. Akin to a branching path in a forest, switches enable game characters to undertake different actions based on unique conditions.
What is it for?
Without GDScript Switch, you may find yourself writing a lengthy chain of ‘if’ and ‘elif’ statements to handle different outcomes. Switch simplifies this process with a more concise, readable, and efficient pattern.
Why Should I Learn It?
Mastering GDScript switches is a crucial step for any aspiring game developer, affording you greater flexibility and control over your game’s dynamics. From determining a player’s movement to branching narratives, it’s a key instrument in your coding orchestra.
Basic GDScript Switch Usage
Let’s start with a basic switch statement in GDScript. Suppose you have a game character that can behave differently based on the tools they have currently selected.
tool = 'sword' match tool: 'sword': print('The character chose the sword') 'shield': print('The character chose the shield') 'bow': print('The character chose the bow')
In this code, if ‘tool’ equals ‘sword’, the print statement for ‘sword’ will execute. If ‘tool’ equals ‘shield’, the print statement for ‘shield’ will execute, and so on.
Using Enums with Switch
In GDScript, Enums are a useful way to define a type that consists of a set of named values, such as game states.
enum gameState {menu, started, paused, gameover} var currentGameState = gameState.menu match currentGameState: gameState.menu: print('Game is in menu state.') gameState.started: print('Game started.') gameState.paused: print('Game is paused.') gameState.gameover: print('Game is over.')
With Enums, you’re able to make your switch cases understandable, maintainable, and less prone to errors.
Different Actions Based on Value Ranges
In GDScript, you can use switch cases that handle ranges of values. This can be the player’s health, for example.
player_health = 50 match player_health: 76..100: print("Health is High") 51..75: print("Health is Medium") 26..50: print("Health is Low") 1..25: print("Health is critical") _: print("Player is dead")
In this example, if the player’s health is between 76 and 100, it labels the health as high. If it’s between 51 and 75, it labels the health as medium, and so on.
Using The Default Case (‘_’)
It’s always good practice to have a default case to capture everything the switch statement hasn’t explicitly defined.
tool = 'axe' match tool: 'sword': print('The character chose the sword') 'shield': print('The character chose the shield') 'bow': print('The character chose the bow') _: print('This tool is not supported')
In this case, the ‘_:’ syntax represents the default scenario. If ‘tool’ is ‘axe’, no specific case statement matches, so the print statement in the default case will execute.
Dealing With Complex Match Conditions
The ‘match’ statement is like a more powerful version of ‘switch’ from other languages. It can also handle complex conditions well.
game_entity = ['orc', 100] match game_entity: ['dragon', 200]: print('The entity is a dragon with 200 HP.') ['orc', _]: print('The entity is an orc.') [_, 100]: print('The entity has 100 HP.') _: print('Unknown entity')
In this example, it’s matching an array of two elements. The ‘game_entity’ indicates the type and HP of a character. If the character is an orc (irrespective of HP), ‘The entity is an orc.’ would print. If the character’s HP is 100, ‘The entity has 100 HP’ would print.
Combining Values
In GDScript, you can combine values in a match statement to check multiple conditions:
tool, isEquipped = 'knife', false match tool, isEquipped: 'sword', true: print('The player is equipped with a sword!') 'shield', true: print('The player is equipped with a shield!') 'knife', true: print('The player is equipped with a knife!') _, false: print('The player is not equipped!')
In this example ‘match’ is checking both the tool type and whether it is equipped.
Nested Matches
If you have a condition that requires another condition to be evaluated first, you can nest matches within matches.
weapon = 'dagger' enraged = true match weapon: 'sword': print('The player has a sword. Now checking rage...') match enraged: true: print('The player is enraged and swings for 2x damage!') 'axe': print('The player has an axe and does not know the concept of rage')
In this case, if the weapon is a sword, another match statement checks if the player is enraged. If the player is both wielding a sword and enraged, the output will be ‘The player is enraged and swings for 2x damage!’
Ignoring Values
Sometimes, you might want to ignore some values when matching. In this case, you can use ‘_’.
items = ['potion', 2] match items: ['potion', _]: print('Player has a potion! I don’t care how many.') ['elixir', _]: print('Player has an elixir! I don’t care how many.') [_,_]: print('Player does not have a potion or an elixir.')
In this example, it matched the item type (first array element) and ignored the count (second array element).
Remember, programming empowers us to bring our videogame worlds into existence precisely as we envision them. Mastering the ‘match’ construct is one clear path towards that goal. It’s time to take control. Happy coding!
Pattern Sequence
In GDScript, how you sequence your patterns is crucial since the engine evaluates them from top to bottom. If a match is found, it will break out of the structure, and the remaining patterns will not be evaluated. Let’s consider a simple example to illustrate this:
npc_action = 'attack' match npc_action: 'attack', 'run': print('The NPC decided to attack or run') 'sleep': print('The NPC is asleep')
In this example, even though ‘attack’ will match the first case, the print statement will never be executed since ‘run’ doesn’t match. This demonstrates how crucial pattern sequence can be.
Matching with Dictionaries
Just like arrays, match can be used with dictionaries:
var character = {"name": "Thror", "race": "dwarf"} match character: {"name": _, "race": "dwarf"}: print("The character is a dwarf") {"name": _, "race": _}: print("Unknown race")
This code matches the race attribute of the character dictionary. If the race attribute is “dwarf”, it will print ‘The character is a dwarf’. For any other race, it will print ‘Unknown race’.
Multiple Patterns for a Single Code Block
A useful feature of GDScript’s match statement is that you can list multiple patterns to be matched by a single block of code:
state = 'idle' match state: 'attack', 'run', 'jump': print('The player is in action state') 'idle': print('The player is idle')
Here ‘attack’, ‘run’, and ‘jump’ are each separate patterns, but they all lead to the same outcome: printing ‘The player is in action state’.
Using More Advanced Patterns
GDScript match also supports advanced patterns like types and classes. You can match patterns based on their type or class:
var entity = [Vector2(10, 20), 'orc'] match entity: [is Vector2, 'orc']: print('An orc located at '+str(entity[0])) [is Vector2, _]: print('An unknown entity located at '+str(entity[0]))
In this example, we have an array that consists of a location represented by a Vector2 and a type represented by a string. Imagine a game where the engine needs to decide how to react to different types of entities. When the entity is ‘orc’ it outputs ‘An orc located at (10, 20)’. For an unknown entity, it outputs ‘An unknown entity located at (10, 20)’. It’s a handy way to manage entity interaction.
A deep understanding of GDScript’s switch mechanism allows programmers to write more readable, efficient, and maintainable code. With it, you’re equipped to tackle any challenge that comes your way as a game developer. Stick with us, your journey to becoming a master developer is just getting started.
Where to Go Next?
Congratulations on diving into the exciting intricacies of the GDScript switch statement! But don’t stop here. There’s a whole universe of game development concepts and tools waiting for you to explore. Continue to challenge yourself, experiment, learn, and before you know it, you’ll be creating immersive games all on your own.
We suggest steering your gaming journey ahead with our fully-featured Godot Game Development Mini-Degree. This mini-degree offers a comprehensive curriculum with seven project-based courses that practically guide you through creating your very own 2D and 3D games using Godot 4. The knowledge and experience gained through this mini-degree open the doors to endless opportunities in the ever-growing game development industry.
Looking for more? Explore our collection of expansive Godot Courses. At Zenva, whether you’re a beginner just setting foot in the gaming realm or a professional looking to polish your skills, we’ve got a course for you. Code, create, and conquer. Your game development journey continues here!
Conclusion
Exploring GDScript switch statements is just one part of your path to becoming a well-rounded game developer. Crafting compelling games involves a combination of technical skills, creativity, and persistence. The hard work put in learning these skills is not just rewarding; it’s also a lot of fun!
Remember, every step you take brings you closer to creating the games you’ve dreamed of. Don’t stop learning and innovating! Keep the momentum going and join us on our Godot Game Development Mini-Degree for a further deep dive into the world of game design and development with Godot. Exciting adventures await in the realm of game development!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
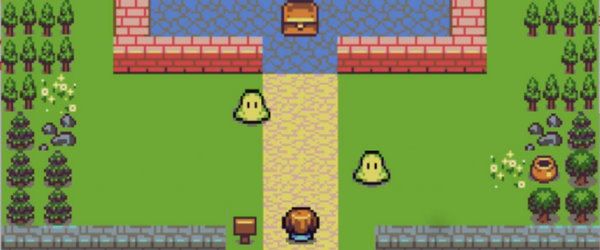
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.