Welcome to an exciting journey into the world of GDScript functions! If you have a keen interest in game development, or you’re simply looking to add a new programming language to your repertoire, then you’re surely in the right place.
Table of contents
Understanding GDScript
First, let’s understand the basics of GDScript. GDScript is a high-level, dynamically typed programming language that is principally used for creating games with the Godot engine. If you are familiar with Python, you will easily catch up with GDScript as they share a lot of similarities in syntax and structure.
What are GDScript functions?
GDScript functions are the building blocks of your game logic in Godot. They represent blocks of code that can carry out specific tasks, and can be reused throughout your game.
What are GDScript functions used for?
The primary use of GDScript functions is for game logic. They can handle things like player movement, game state changes, interaction with game objects, and much more.
Why learn GDScript functions?
Learning GDScript functions is vital for developing games in the Godot engine. Knowing how to create and manipulate functions enables you to design more complex and interactive games, thereby improving the overall player experience.
The beauty about GDScript functions lies in their reusability. Rather than writing the same piece of code multiple times, functions allow you to write once and use anywhere in your game. This leads to cleaner, more maintainable code.
This tutorial aims to provide a comprehensive understanding of GDScript functions, filled with simple game mechanics and analogies to ensure an engaging learning experience. Whether you’re an early stage learner or an experienced coder, there’s something valuable for you in this tutorial. So let’s jump right in and start creating!
Defining a GDScript Function
Defining a function in GDScript is done using the keyword func, followed by the name of the function, and then parentheses (). Inside the parentheses, you can specify parameters that your function takes, if any. The function body is contained within braces {}.
func my_function(): pass # This is an empty function
If your function takes parameters, you would include them inside the parentheses like so:
func my_function_with_parameters(param1, param2): pass # This is an empty function with parameters
GDScript Function Return Values
GDScript allows functions to return a value to the caller. This is done using the return keyword followed by the value or expression to return. If no return is specified, the function will implicitly return null.
func get_total_score(score1, score2): return score1 + score2
Calling a GDScript Function
Calling a GDScript function is as simple as mentioning the function by name followed by parentheses. If the function takes parameters, these would go inside the parentheses.
get_total_score(10, 20) # This will return 30
Built-in GDScript Function
Godot offers a variety of built-in GDScript functions that can be used to manipulate various aspects of your game. For instance, the print() function outputs text to the console.
print("Hello, Zenva Learners!") # Outputs "Hello, Zenva Learners!" to the console
In the next part of our tutorial, we would go in depth into building more complex GDScript functions and using more built-in functions. Happy coding!
Understanding Scope in GDScript Functions
Before we proceed, it’s crucial to understand the concept of scope in GDScript. Scope pertains to where a variable or a function is accessible within your code. Variables declared inside a function are local to that function and cannot be accessed outside of it.
func calculate_score(): var score = 10 # Local variable print(score)
In the example above, score is a local variable because it is declared within the function ‘calculate_score()’. It is accessible only within this function.
GDScript Function Expressions
GDScript supports using expressions inside functions. An expression could be a mathematical operation, a call to another function, or a logical expression.
func calculate_total(score1, score2): return score1 * score2 # Multiplication expression
In this example, the return statement uses a multiplication operation to compute the product of ‘score1’ and ‘score2’.
func calculate_total(score1, score2): var total = score1 + score2 # Addition expression return total
Here, we used an addition operation to calculate the total and stored the result in the variable ‘total’.
GDScript Recursive Functions
Recursive functions are a special type of function that call themselves within their own function body. This is extremely useful when solving problems that can be broken down into simpler, repetitive tasks. A simple implementation might look like this:
func factorial(n): if n == 1: return 1 else: return n * factorial(n - 1) # Function calls itself
The ‘factorial’ function above calculates the factorial of a number ‘n’ by recursively multiplying ‘n’ by the factorial of ‘n – 1’ until ‘n’ equals 1.
GDScript Default Parameter Values
GDScript functions also support default parameter values. If you do not pass a value for a parameter with a default value, the function will use the default value.
func add_bonus(score, bonus = 5): return score + bonus
In this example, the ‘add_bonus’ function takes an optional second parameter ‘bonus’ which defaults to 5. If ‘bonus’ is not provided when calling the function, it will use the default value of 5.
That’s it for now! We’ll explore more complex GDScript functions in future articles. Keep coding, keep exploring.
GDScript Anonymous Functions
One cool feature in GDScript is the provision of anonymous functions, also known as lambdas. These are functions that are not bound to an identifier and can be used to create inline functions.
var square = func(x) : x * x # Anonymous function print(square(5)) # Outputs 25
The anonymous function here takes a parameter ‘x’ and returns the square of ‘x’.
Passing Functions As Parameters
In GDScript, functions are first-class citizens, meaning you can pass functions as parameters to other functions.
func call_twice(func, arg): return func(arg, arg)
This function, `call_twice`, takes another function `func` and an argument `arg`. It calls the function `func` with `arg` as parameter twice.
func sum(x, y): return x + y print(call_twice(sum, 10)) # Outputs 20
In the example above, sum is passed as an argument to `call_twice`, which then calls `sum` with 10 as argument, twice.
GDScript Signal Functions
Signals are a tool in Game Development for making different game components communicate with each other. In GDScript, you can create signals using the `signal` keyword.
signal score_updated # Declaring a signal
You can emit this signal from within a function using `emit_signal()` method.
var score = 0 func add_score(additional_score): score += additional_score emit_signal("score_updated")
Conclusion
In this tutorial, we’ve delved into a comprehensive exploration of GDScript functions. We’ve covered defining, calling, and working with different types of functions such as recursive, anonymous and signal functions.
The understanding of GDScript functions is pivotal for any aspiring game developer. Equipped with this knowledge, developers can proceed to create action-packed and interactive video games on the Godot Engine!
Keep exploring, keep getting better, and keep raising the bar for your gaming adventures. Your potential is endless, and as always, we at Zenva are here to guide you along your game development journey. Cheers to many more lines of creative coding!
What’s Next?
Building upon your newly acquired knowledge, the next big step to enhancing your Godot development skills is hands-on practice. Whether you aspire to create a simple 2D game or a 3D blockbuster, the key is to refine your understanding of the various features and complexities that the Godot engine has to offer.
To help you along the way, we’ve developed a comprehensive program, our Godot Game Development Mini-Degree. It covers a wide range of topics, including using 2D and 3D assets, game mechanics for RPGs, RTS games, survival games, and platformers, control flow, and even UI systems. This program is fitting for both beginners and experienced developers, with step-by-step guidance provided throughout.
If you desire to venture into more specialized subjects or need a rounded understanding of different aspects of Godot, you can also explore our broad collection of Godot Courses.
At Zenva, we offer a plethora of beginner-to-professional courses in programming, game development, and AI to enable you to learn coding, develop games, and earn certificates. With our support, you can go from an absolute beginner to a professional game developer. The game development world is vast and thrilling, and we’re excited to accompany you on this rewarding journey.
Conclusion
Our journey through the realm of GDScript functions has been an engaging and enlightening one, providing you with a valuable asset to enhance your game development capabilities. As we’ve demonstrated throughout, mastering these functions is crucial to fully harnessing the power of the Godot engine, paving the way for the creation of imaginative and immersive gaming experiences.
The world of game development is vast and exciting, with countless opportunities for aspiring creators like you. At Zenva, we provide an array of comprehensive courses that will equip you with all the skills you need to navigate this landscape confidently. Our Godot Game Development Mini-Degree is the perfect platform to polish your GDScript prowess and fuel your game development adventure. So, embrace your passion for game creation and join us on this journey. Let’s code, create, and conquer the gaming world together!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
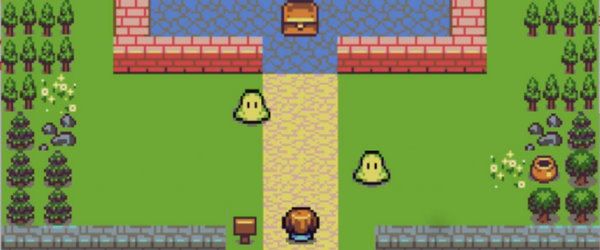
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.