In today’s interconnected world, diagnosing and analyzing network systems is a vital skill. This is especially important in the realms of cybersecurity and network administration. Yet, how does one easily and effectively dive into the seemingly daunting sea of network packets? Enter Scapy, a powerful Python library for networking.
Table of contents
What is Scapy?
Scapy is a robust packet manipulation program and library. It is implemented in Python and allows its users to interact with the network at low levels. Essentially, you can sniff, dissect, forge and manipulate packets in countless ways, all through the comfort of Python scripting.
Why Should You Learn Scapy?
With Scapy, you gain control over the very backbone of the internet – packets. This knowledge and skillset is not just essential for network engineers and system administrators, but also proves invaluable for aspiring game developers and programmers. Mastering Scapy will help you perform:
- Network discovery and security auditing
- Network testing and quality assurance
- Advanced network packet manipulation for gaming
As Scapy’s easy-to-use, intuitive structure fosters an engaging learning experience, understanding the ins and outs of networking becomes more accessible and less intimidating. Now that you know the ‘what’ and the ‘why’, let’s delve into the hands-on portion of this tutorial.
Getting Started With Scapy
Before we get into working with Scapy, ensure you have it installed on your system.
pip install scapy
Basic Packet Creation
At the heart of Scapy is the ability to create packets. Here’s a simple way to create an IP packet.
from scapy.all import * packet = IP(dst="192.168.1.1")/ICMP() send(packet)
This will create and send a ping ICMP packet to the IP address “192.168.1.1”.
Packet Sniffing
Scapy can also sniff packets from your network. Here’s a simple example to demonstrate packet sniffing.
from scapy.all import * packets = sniff(count=10) packets.summary()
This will sniff 10 packets on the network and then summarize their data.
Packet Manipulation
But what if we want to manipulate an existing packet? Here’s how to do it.
from scapy.all import * packets = sniff(count=1) packets[0][IP].src = "192.168.1.1" send(packets)
This code will first sniff a packet, then change its source IP address to “192.168.1.1”, then send it back out.
Packet Dissection
A key part of understanding network data is being able to dissect packets into their individual components.
from scapy.all import * packets = sniff(count=1) packets[0].show()
The “show” method here will print a detailed breakdown of the packet’s contents.
Scapy is a powerful, versatile tool for anyone who wants to delve into the world of network packets – be it for security, network management, or game development. As with any programming skills, mastering Scapy takes practice. So, get on coding, create, dissect, manipulate packets and explore the possibilities of networking!
Crafting Specific Packets
In some cases, you might want to customize a packet to mimic a certain communication or test specific responses. Let’s say we want to create a TCP packet:
from scapy.all import * packet = IP(dst="192.168.1.1")/TCP(dport=80) send(packet)
This will create and send a TCP packet to the IP address “192.168.1.1” over port 80, commonly used for HTTP traffic.
Packet Layer
Scapy allows you to manipulate specific layers of a packet as well. Here’s how you can modify the TCP layer.
from scapy.all import * packet = IP(dst="192.168.1.1")/TCP(dport=80) packet[TCP].sport = 443 send(packet)
This code changes the source port of the TCP packet to 443, commonly used for HTTPS traffic.
Packet Fields
Scapy also allows manipulation of individual packet fields. Want to change the sequence number field of a TCP packet? It’s as easy as this:
from scapy.all import * packet = IP(dst="192.168.1.1")/TCP(dport=80) packet[TCP].seq = 9999 send(packet)
This piece of code changes the sequence number of the TCP packet to 9999.
Packet Payloads
Not only can you manipulate packets at a high level, but Scapy can also handle payloads. Let’s create a TCP packet with a specific payload.
from scapy.all import * payload = "Hello, Zenva!" packet = IP(dst="192.168.1.1")/TCP(dport=80)/payload send(packet)
This will create and send a TCP packet with the payload “Hello, Zenva!” to the IP address “192.168.1.1”.
At its core, Scapy is designed to make network packet handling more accessible and intuitive. It allows us to pierce through the intricate layers of the network envelope, read its secrets, and even draft our own. Play around with the examples, create more complex scenarios, and delve deeper into Scapy’s capabilities, embarking on an exciting journey through the vast, enthralling universe of network packets.
Where to Go Next?
Having taken your first steps into the world of network packet sniffing, manipulation and dissection, you might be wondering, where do I go from here? The answer is simple – keep learning, keep growing!
One such stellar learning path that we provide is our Python Mini-Degree. The Python Mini-Degree is an exhaustive collection of courses that demystify the elegant and versatile Python language. Apt for newbies and experienced coders alike, the Python Mini-Degree covers an array of topics:
- Fundamental coding principles
- Algorithms
- Object-oriented programming
- Game and app development
Our holistic approach to teaching Python is project-based, emphasizing hands-on experience. You’ll get your hands dirty coding, creating exciting projects like a medical diagnosis bot and a to-do list app.
Python or otherwise, step into our world of exciting, accessible programming coursework. Here’s where to find a more broad collection of our Python courses.
Conclusion
That wraps up our journey into the vast, fascinating world of network packet sniffing, manipulation, and analysis with Scapy. With these new skills, you can go from merely using the internet to understanding its underlying system. The ability to dissect and manipulate network packets empowers you to create more secure, efficient, and innovative solutions, regardless of your field.
At Zenva, we’re ever-ready to accompany you on your learning expedition. As you continue your exploration of networking, Python scripting working with Scapy, we invite you to explore our comprehensive Python Mini-Degree aswell. Let’s continue this exciting journey of discovery and mastery, together. Happy coding!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
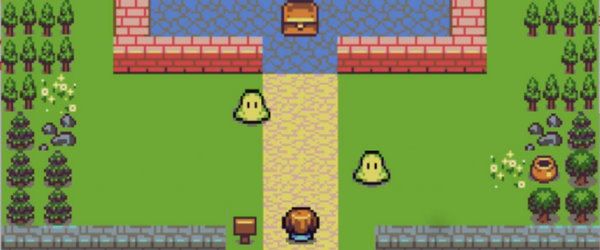
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.