Welcome to Zenva’s tutorial on Roblox Debugging – an important aspect of every coder’s journey, even more so for those focused on game creation. This guide will demystify the process of bug clearing, squashing potential problems and glitches that might be obstructing your path to a seamless gaming experience. Despite seeming a daunting concept initially, debugging can become a rewarding and enlightening process with just a bit of knowledge and practice.
Table of contents
So, What is Roblox Debugging?
At its core, Roblox Debugging is the process of identifying and rectifying issues within your Roblox code. These problems, commonly known as ‘bugs’, often lead to unexpected behavior, crashes, or other unwanted outcomes in your game world.
What is it used for?
Debugging in Roblox is a crucial tool to ensure your game functions as you intend it to. By uncovering bugs in the code, you’re better equipped to provide players with a harmonious, enjoyable game experience. Remember – the less distractions and problems a user faces, the more immersed they can become in the world you’ve created.
Why should I learn Roblox Debugging?
By learning Roblox Debugging, you not only improve the performance of your game but also deepen your understanding of coding. It allows you to pinpoint specific problematic areas, analyze what went wrong, and learn from it, expanding your coding expertise in the process.
Getting Started with Roblox Debugging
First off, let’s understand how to access the Debugging Menu in Roblox Studio. It’s as simple as opening the ‘View’ tab on the upper menu, and clicking on the ‘Output’ and ‘Script Analysis’ options.
//Debugging Menu Access: //1. Open Roblox Studio. //2. Click on 'View' tab. //3. Select 'Output' and 'Script Analysis'.
Logging Messages with print() Function
The print() function in Roblox is a simple yet powerful tool for debugging. Developers often use it to keep tabs on what’s happening in the code during runtime.
print("Hello, Zenva!")
The example above would output the message ‘Hello, Zenva!’ in the console during the run-time of the script, proving incredibly useful for understanding the flow of your code.
Error Message Interpretation
It’s an inevitable part of game development that you’d run into errors. However, understanding these error messages is essential to hone your debugging skills.
//Suppose you have a line with an error, such as: 1 + nil
In the case above, the console would output something akin to the following:
Error: line(1): attempt to <a href="https://gamedevacademy.org/lua-math-tutorial-complete-guide/" title="Lua Math Tutorial – Complete Guide" target="_blank" rel="noopener">perform arithmetic</a> on a nil value /* The error message usually contains: - The line number where the error occurred. - A description of the error.
Using If Statements for Debugging
If statements can be an effective tool for circumventing or identifying particular issues in your code.
//Suppose you have a function that handles player damage: function takeDamage(player, damage) player.HP -= damage end //To ensure 'player' exists and 'damage' is a number, you could modify it like so: function takeDamage(player, damage) if player and typeof(damage) == 'number' then player.HP -= damage else print('Invalid inputs to takeDamage function') end end
In the example above, the function only operates if the player exists and the damage is a number. If not, it would log a message in the console, aiding in identifying the issue.
Understanding Warns
Another powerful debugging tool Roblox offers is the ‘warn’ function which helps in displaying messages in yellow within the output window. Thus, you can easily distinguish the warn messages in a sea of prints and logs.
warn("This is only a warning!")
In this snippet, the console would output a yellow line reading ‘This is only a warning!’, thus quickly grabbing your attention to a potential issue.
Debugging User-Generated Events
It’s also important to debug events initiated by user interaction. For example, let’s consider a situation where the user fires a weapon:
//Let's assume 'fireGun' function fires the weapon: function fireGun(player) if player.ammo > 0 then //fire the gun player.ammo -= 1 else warn('Out of ammo!') end end
Here, the gun should only fire if the player has ammo. If not, a warning is issued in the console. This helps you ensure players cannot fire without ammo.
Using Breakpoints and Stepping Through Code
Roblox Studio supports breakpoints, which are points in the code that, when hit during execution, pause the program. You can then step through code one line, or statement, at a time, examining the output and watching how data changes.
//Assuming the following script: local myArray = {10, 20, 30, 40, 50} for i, v in ipairs(myArray) do print(i, v) end
To set a breakpoint, you simply need to click on the line number beside the code. In the script above, you might put a breakpoint on the print statement to examine how values of ‘i’ and ‘v’ change each iteration.
These are just some of the powerful tools and strategies at your disposal for debugging in Roblox. By learning to utilize them, you can boost your coding efficiency, create better performing games, and elevate your skillset to new heights. Happy debugging!
Unleashing the Power of Debugging
As we dive deeper into debugging, let’s explore more tools and techniques you can use to squash bugs and improve your games. Remember that each of these tools has its place, and understanding when to use one over the other is part of mastering the debugging process.
The pcall Function
Roblox provides a built-in function, pcall (protected call), which allows a block of code to be executed without breaking the entire script when an error occurs.
//Assume we have a function which could potentially generate an error, like divide: function divide(a, b) return a / b end //Normally, calling divide(9, 0) would result in an error crashing our script. //But using pcall: local success, result = pcall(divide, 9, 0) if success then print(result) else warn('An error occurred: '..result) end
In this example, we attempt to execute the divide function within a protected call. If it’s successful, we print the result. If it leads to an error, it doesn’t crash our script, but instead, it lets us handle the error gracefully by issuing a warning in the console.
The xpcall Function
An extension of pcall is the xpcall (extended protected call) function, which allows you to define a specific ‘error handling’ function to run in case of an error.
function errorHandling(err) //Do something with the error, such as logging it: warn('An error occurred: '..err) end local success, result = xpcall(divide, errorHandling, 9, 0)
In this snippet, if an error occurs during our attempted division, we are running custom error handling logic with xpcall, providing us with a great deal of flexibility for debugging.
The assert Function
‘Assert’ is another helpful debugging function that can be used to check if a condition is true. If not, it will immediately cause an error, allowing you to stop a potentially harmful operation from proceeding.
function addPositiveNumbers(a, b) assert(a >= 0 and b >= 0, "Inputs must be positive numbers!") return a + b end
Here, the function addition only proceeds if both numbers are positive. If they aren’t, the script stops and an error message is triggered.
Debugging Functions with Unknown Arguments
Another advantageous debugging tip is dealing with functions that may have variable, or unknown, argument counts. A potential strategy is using table.unpack along with the {…} spread operator.
function unknownArgs(...) local args = {...} for i, v in ipairs(args) do print('Argument '..i..' = '..tostring(v)) end end unknownArgs(1, "two", 3, "four", 5)
This function accepts an unknown number of arguments and prints each one out. This understanding helps you make sense of your code by seeing what arguments, and in what order, are passed into any particular function.
Now that we’ve explored robust debugging strategies, you can approach any bug with confidence that you have the tools and the know-how to conquer it. With patience, a dedicated mindset, and your newfound debugging knowledge, you’ll undeniably feel more confident every step of the way on your coding journey.
Where to Go Next
With the knowledge acquired from this tutorial, you’ve begun a critical journey on debugging in Roblox, enhancing both your coding prowess and ability to build smoother and more enjoyable gaming experiences.
At Zenva, we are passionate about helping you further your learning and broaden your horizons. We offer a diverse range of courses, from beginner to professional levels, in programming, game development and artificial intelligence. With over 250 supported courses, you can gain insights, strengthen your skills, and earn valuable certifications.
For those keen on diving into the fascinating world of game creation using Roblox Studio and Lua, we highly recommend our Roblox Game Development Mini-Degree. This collection of thorough, project-based courses spans a variety of genres, equipping you with the skills to create everything from obstacle courses to multiplayer FPS games. With our instructors – experienced gamers and certified coders – you’ll learn at your own pace, with 24/7 access to course material, regular updates to stay current with industry trends, and the opportunity to strengthen your learning through coding challenges and quizzes.
We also offer an extensive array of Roblox courses catering to different interests and coding levels. So, no matter where you are in your development journey, looking for an introduction or advancing your skills, we’ve got you covered.
At Zenva, we believe in your coding potential and aim to equip you with the right knowledge and tools to transform your ideas into reality. Here’s to your ongoing coding journey and the amazing games you’re bound to create!
Conclusion
Debugging in Roblox might seem daunting initially, but it’s an empowering tool in your hands to create an immersive and seamless gaming world. As you’ve seen throughout this tutorial, it enables you to not only mark and rectify issues in your code, but also deepen your understanding of coding principles and logic.
Here at Zenva, we’re excited to accompany and assist you throughout your coding journey. With our industry-leading resources, interactive lessons, and hands-on, project-based courses like the Roblox Game Development Mini-Degree, you’re on your way to mastering debugging in Roblox and beyond, ready to take on the world of game creation! So dive in, cultivate your knowledge, squash those bugs, and let your gaming imagination flow!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
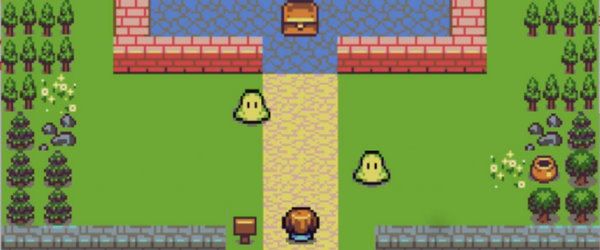
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.